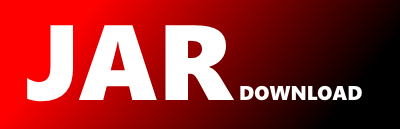
com.github.xiaoyuge5201.component.AliYunSmsClient Maven / Gradle / Ivy
The newest version!
package com.github.xiaoyuge5201.component;
import com.alibaba.fastjson.JSONObject;
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.IAcsClient;
import com.aliyuncs.dysmsapi.model.v20170525.SendSmsRequest;
import com.aliyuncs.dysmsapi.model.v20170525.SendSmsResponse;
import com.aliyuncs.exceptions.ClientException;
import com.aliyuncs.http.MethodType;
import com.aliyuncs.profile.DefaultProfile;
import com.aliyuncs.profile.IClientProfile;
import com.github.xiaoyuge5201.enums.AliyunSmsApiErrorCode;
import com.github.xiaoyuge5201.exception.SmsException;
import com.github.xiaoyuge5201.props.AliYunSMSProperties;
import com.google.gson.Gson;
import java.util.HashMap;
import java.util.Map;
import java.util.Random;
/**
* 阿里雲
*
* @author yugb
*/
public class AliYunSmsClient {
private Gson gson;
/**
* 阿里云短信
*/
private AliYunSMSProperties aliYunSMSProperties;
public AliYunSmsClient(Gson gson, AliYunSMSProperties aliYunSMSProperties) {
this.gson = gson;
this.aliYunSMSProperties = aliYunSMSProperties;
}
/**
* 发送短信
*
* @param phone 手机
* @param templateCode 模板id
*/
public JSONObject doSendSms(String phone, String templateCode) {
IClientProfile profile = DefaultProfile.getProfile(aliYunSMSProperties.getRegionId(), aliYunSMSProperties.getAccessKeyId(), aliYunSMSProperties.getAccessKeySecret());
JSONObject jsonObject = new JSONObject();
try {
//验证码
String code = String.valueOf(new Random().nextInt(666666) + 100000);
Map map = new HashMap<>(1);
map.put("code", code);
DefaultProfile.addEndpoint(aliYunSMSProperties.getEndPoint(), aliYunSMSProperties.getRegionId(),
aliYunSMSProperties.getProduct());
IAcsClient client = new DefaultAcsClient(profile);
SendSmsRequest request = new SendSmsRequest();
request.setMethod(MethodType.POST);
//必填:短信签名-可在短信控制台中找到
request.setSignName(aliYunSMSProperties.getSignName());
//必填:短信模板-可在短信控制台中找到
request.setTemplateCode(templateCode);
//可选:模板中的变量替换JSON串,如模板内容为"亲爱的${name},您的验证码为${code}"时,此处的值为
request.setTemplateParam(gson.toJson(map));
request.setPhoneNumbers(phone);
request.setConnectTimeout((int) aliYunSMSProperties.getConnectionTimeout().toMillis());
request.setReadTimeout((int) aliYunSMSProperties.getReadTimeout().toMillis());
SendSmsResponse sendSmsResponse = client.getAcsResponse(request);
if (!"OK".equals(sendSmsResponse.getCode())) {
//throw new SmsException("短信验证码出错:" + AliyunSmsApiErrorCode.getMessage(sendSmsResponse.getCode()));
jsonObject.fluentPut("status", sendSmsResponse.getCode());
} else {
jsonObject.fluentPut("status", 200);
jsonObject.fluentPut("code", code);
}
jsonObject.fluentPut("mesg", AliyunSmsApiErrorCode.getMessage(sendSmsResponse.getCode()));
} catch (ClientException e) {
e.printStackTrace();
} catch (SmsException smsException) {
smsException.printStackTrace();
}
return jsonObject;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy