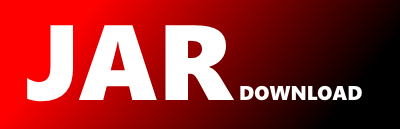
com.github.xiaoyuge5201.StaticFileModifyUtils Maven / Gradle / Ivy
package com.github.xiaoyuge5201;
import cn.hutool.core.io.FileUtil;
import com.github.xiaoyuge5201.request.ResponseResult;
import org.apache.commons.lang3.StringEscapeUtils;
import org.springframework.util.ResourceUtils;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.List;
/**
* 在線修改靜態文件
*
* @author yugb
* @since 2021-04-22
*/
public class StaticFileModifyUtils {
/**
* 获取所有的静态文件树形结构数据, 用于树形结构展示
*
* @return 获取所有的静态文件树形结构数据
* @throws FileNotFoundException 异常信息
*/
public static ResponseResult list() throws FileNotFoundException {
String filePath = ResourceUtils.getURL("classpath:").getPath();
List fileList = new ArrayList<>();
getAllFilePaths(filePath, fileList, 0, "");
return ResponseResult.success(fileList);
}
/**
* 递归获取文件地址
*
* @param filePath 文件地址
* @param filePathList 文件路径列表
* @param level 级别
* @param parentPath 上级路径
* @return 文件路径列表
*/
private static List getAllFilePaths(String filePath, List filePathList, Integer level, String parentPath) {
File[] files = new File(filePath).listFiles();
if (files == null) {
return filePathList;
}
for (File file : files) {
int num = filePathList.size() + 1;
StaticFile staticFile = new StaticFile();
staticFile.setName(file.getName());
staticFile.setId(num);
staticFile.setpId(level);
if (file.isDirectory()) {
if (level == 0) {
if (file.getName().equals("templates") || file.getName().equals("static")) {
staticFile.setOpen(true);
filePathList.add(staticFile);
parentPath = "/" + file.getName();
getAllFilePaths(file.getAbsolutePath(), filePathList, num, parentPath);
num++;
}
} else {
filePathList.add(staticFile);
String subParentPath = parentPath + "/" + file.getName();
getAllFilePaths(file.getAbsolutePath(), filePathList, num, subParentPath);
num++;
}
} else {
if (level != 0) {
staticFile.setFilePath(parentPath + "/" + file.getName());
filePathList.add(staticFile);
num++;
}
}
}
return filePathList;
}
/**
* 根据某个文件的路径获取文件内容
*
* @param filePath 文件路径
* @return 文件内容
* @throws FileNotFoundException 异常信息
*/
public static ResponseResult getContent(String filePath) throws FileNotFoundException {
String path = ResourceUtils.getURL("classpath:").getPath();
String content = FileUtil.readUtf8String(path + filePath);
return ResponseResult.success(content);
}
/**
* 将修改后的内容写入到源路径中
*
* @param staticFile 文件
* @return 结果
* @throws FileNotFoundException 异常信息
*/
public static ResponseResult write(StaticFileRequestVO staticFile) throws FileNotFoundException {
String path = ResourceUtils.getURL("classpath:").getPath();
File file = new File(path + staticFile.getFilePath());
long lastModified = file.lastModified();
FileUtil.writeUtf8String(StringEscapeUtils.unescapeHtml4(staticFile.getContent()), path + staticFile.getFilePath());
file.setLastModified(lastModified);
return ResponseResult.success();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy