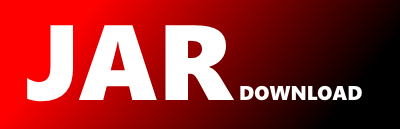
org.xmlet.xsdparser.xsdelements.XsdSimpleContent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xsdParser Show documentation
Show all versions of xsdParser Show documentation
A solution that parses a XSD file into a Java Structure.
The newest version!
package org.xmlet.xsdparser.xsdelements;
import org.xmlet.xsdparser.core.XsdParserCore;
import org.xmlet.xsdparser.core.utils.ParseData;
import org.xmlet.xsdparser.xsdelements.elementswrapper.ConcreteElement;
import org.xmlet.xsdparser.xsdelements.elementswrapper.ReferenceBase;
import org.xmlet.xsdparser.xsdelements.elementswrapper.UnsolvedReference;
import org.xmlet.xsdparser.xsdelements.visitors.XsdAbstractElementVisitor;
import javax.validation.constraints.NotNull;
import java.util.Map;
import java.util.function.Function;
/**
* A class representing the xsd:simpleContent element.
*
* @see xsd:simpleContent description and usage at w3c
*/
public class XsdSimpleContent extends XsdAnnotatedElements {
public static final String XSD_TAG = "xsd:simpleContent";
public static final String XS_TAG = "xs:simpleContent";
public static final String TAG = "simpleContent";
/**
* The {@link XsdRestriction} instance that should be applied to the {@link XsdSimpleContent} instance.
*/
private ReferenceBase restriction;
/**
* The {@link XsdExtension} instance that is present in the {@link XsdSimpleContent} instance.
*/
private ReferenceBase extension;
private XsdSimpleContent(@NotNull XsdParserCore parser, @NotNull Map elementFieldsMapParam, @NotNull Function visitorFunction) {
super(parser, elementFieldsMapParam, visitorFunction);
}
@Override
public void accept(XsdAbstractElementVisitor visitorParam) {
super.accept(visitorParam);
visitorParam.visit(this);
}
/**
* Performs a copy of the current object for replacing purposes. The cloned objects are used to replace
* {@link UnsolvedReference} objects in the reference solving process.
* @param placeHolderAttributes The additional attributes to add to the clone.
* @return A copy of the object from which is called upon.
*/
@Override
public XsdSimpleContent clone(@NotNull Map placeHolderAttributes) {
placeHolderAttributes.putAll(attributesMap);
XsdSimpleContent elementCopy = new XsdSimpleContent(this.parser, placeHolderAttributes, visitorFunction);
elementCopy.restriction = ReferenceBase.clone(parser, this.restriction, elementCopy);
elementCopy.extension = ReferenceBase.clone(parser, this.extension, elementCopy);
elementCopy.cloneOf = this;
elementCopy.parent = null;
return elementCopy;
}
@SuppressWarnings("unused")
public XsdExtension getXsdExtension() {
return extension instanceof ConcreteElement ? (XsdExtension) extension.getElement() : null;
}
@SuppressWarnings("unused")
public XsdRestriction getXsdRestriction(){
return restriction instanceof ConcreteElement ? (XsdRestriction) restriction.getElement() : null;
}
public static ReferenceBase parse(@NotNull ParseData parseData){
return xsdParseSkeleton(parseData.node, new XsdSimpleContent(parseData.parserInstance, convertNodeMap(parseData.node.getAttributes()), parseData.visitorFunction));
}
public void setRestriction(ReferenceBase restriction) {
this.restriction = restriction;
}
public void setExtension(ReferenceBase extension) {
this.extension = extension;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy