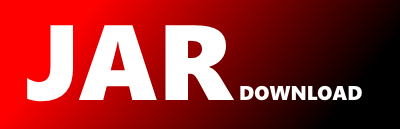
emujava.util.concurrent.impl.PromisesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of backend-teavm Show documentation
Show all versions of backend-teavm Show documentation
Tool to generate libgdx to javascript using teaVM
/*
* Copyright 2016 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package emujava.util.concurrent.impl;
import java.util.function.BiConsumer;
/**
*
*/
final class PromisesImpl implements Promises {
@Override
public Promise allOf(Promise[] promises) {
assert promises.length > 0;
Promise andPromise = new PromiseImpl<>();
BiConsumer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy