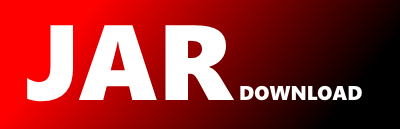
com.badlogic.gdx.physics.bullet.collision.btBvhTriangleMeshShape Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gdx-bullet-teavm Show documentation
Show all versions of gdx-bullet-teavm Show documentation
Tool to generate libgdx to javascript using teaVM
/*-------------------------------------------------------
* This file was generated by JParser
*
* Do not make changes to this file
*-------------------------------------------------------*/
package com.badlogic.gdx.physics.bullet.collision;
import com.badlogic.gdx.graphics.g3d.model.MeshPart;
import com.badlogic.gdx.utils.Array;
import com.badlogic.gdx.physics.bullet.linearmath.btVector3;
import com.badlogic.gdx.math.Vector3;
/**
* @author xpenatan
*/
public class btBvhTriangleMeshShape extends btTriangleMeshShape {
public static btBvhTriangleMeshShape WRAPPER_GEN_01 = new btBvhTriangleMeshShape(false);
protected final static Array instances = new Array();
protected static btBvhTriangleMeshShape getInstance(final Array meshParts) {
for (final btBvhTriangleMeshShape instance : instances) {
if (instance.meshInterface instanceof btTriangleIndexVertexArray && btTriangleIndexVertexArray.compare((btTriangleIndexVertexArray) (instance.meshInterface), meshParts))
return instance;
}
return null;
}
public static btBvhTriangleMeshShape obtain(final Array meshParts) {
btBvhTriangleMeshShape result = getInstance(meshParts);
if (result == null) {
result = new btBvhTriangleMeshShape(btTriangleIndexVertexArray.obtain(meshParts), true);
instances.add(result);
}
result.obtain();
return result;
}
public btBvhTriangleMeshShape(btStridingMeshInterface meshInterface, boolean useQuantizedAabbCompression) {
initObject(createNative((int) meshInterface.getCPointer(), useQuantizedAabbCompression), true);
}
private btStridingMeshInterface meshInterface = null;
/**
* @return The {@link btStridingMeshInterface} this shape encapsulates.
*/
public btStridingMeshInterface getMeshInterface() {
return meshInterface;
}
@org.teavm.jso.JSBody(params = {"meshInterfaceAddr", "useQuantizedAabbCompression"}, script = "var jsObj = new Bullet.btBvhTriangleMeshShape(meshInterfaceAddr, useQuantizedAabbCompression); return Bullet.getPointer(jsObj);")
private static native int createNative(int meshInterfaceAddr, boolean useQuantizedAabbCompression);
@org.teavm.jso.JSBody(params = {"addr"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btBvhTriangleMeshShape); Bullet.destroy(jsObj);")
private static native void deleteNative(int addr);
public btBvhTriangleMeshShape(boolean cMemoryOwn) {
}
public btBvhTriangleMeshShape() {
}
public void performRaycast(btTriangleCallback callback, btVector3 raySource, btVector3 rayTarget) {
performRaycastNATIVE((int) cPointer, (int) callback.getCPointer(), (int) raySource.getCPointer(), (int) rayTarget.getCPointer());
}
@org.teavm.jso.JSBody(params = {"addr", "callbackAddr", "raySourceAddr", "rayTargetAddr"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btBvhTriangleMeshShape);jsObj.performRaycast(callbackAddr, raySourceAddr, rayTargetAddr);")
private static native void performRaycastNATIVE(int addr, int callbackAddr, int raySourceAddr, int rayTargetAddr);
public void performRaycast(btTriangleCallback callback, Vector3 raySourceGDX, Vector3 rayTargetGDX) {
btVector3.convert(raySourceGDX, btVector3.TEMP_0);
btVector3 raySource = btVector3.TEMP_0;
btVector3.convert(rayTargetGDX, btVector3.TEMP_1);
btVector3 rayTarget = btVector3.TEMP_1;
performRaycastNATIVE((int) cPointer, (int) callback.getCPointer(), (int) raySource.getCPointer(), (int) rayTarget.getCPointer());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy