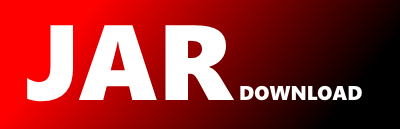
com.badlogic.gdx.physics.bullet.collision.btCollisionShape Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gdx-bullet-teavm Show documentation
Show all versions of gdx-bullet-teavm Show documentation
Tool to generate libgdx to javascript using teaVM
/*-------------------------------------------------------
* This file was generated by JParser
*
* Do not make changes to this file
*-------------------------------------------------------*/
package com.badlogic.gdx.physics.bullet.collision;
import com.badlogic.gdx.math.Vector3;
import com.badlogic.gdx.physics.bullet.BulletBase;
import com.badlogic.gdx.physics.bullet.linearmath.btVector3;
import com.badlogic.gdx.physics.bullet.linearmath.btTransform;
import com.badlogic.gdx.math.Matrix4;
/**
* @author xpenatan
*/
public class btCollisionShape extends BulletBase {
public static btCollisionShape WRAPPER_GEN_01 = new btCollisionShape(false);
public void calculateLocalInertia(float mass, Vector3 inertia) {
btVector3 out = btVector3.TEMP_0;
btVector3.convert(inertia, out);
calculateLocalInertia((int) cPointer, mass, (int) out.getCPointer());
btVector3.convert(out, inertia);
}
@org.teavm.jso.JSBody(params = {"addr", "mass", "inertiaAddr"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btBoxShape); var inertiaJSObj = Bullet.wrapPointer(inertiaAddr, Bullet.btVector3); jsObj.calculateLocalInertia(mass, inertiaJSObj);")
private static native void calculateLocalInertia(int addr, float mass, int inertiaAddr);
public btCollisionShape(boolean cMemoryOwn) {
}
public btCollisionShape() {
}
public void setLocalScaling(btVector3 scaling) {
setLocalScalingNATIVE((int) cPointer, (int) scaling.getCPointer());
}
@org.teavm.jso.JSBody(params = {"addr", "scalingAddr"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btCollisionShape);jsObj.setLocalScaling(scalingAddr);")
private static native void setLocalScalingNATIVE(int addr, int scalingAddr);
public void setLocalScaling(Vector3 scalingGDX) {
btVector3.convert(scalingGDX, btVector3.TEMP_0);
btVector3 scaling = btVector3.TEMP_0;
setLocalScalingNATIVE((int) cPointer, (int) scaling.getCPointer());
}
public void calculateLocalInertia(float mass, btVector3 inertia) {
calculateLocalInertiaNATIVE((int) cPointer, mass, (int) inertia.getCPointer());
}
@org.teavm.jso.JSBody(params = {"addr", "mass", "inertiaAddr"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btCollisionShape);jsObj.calculateLocalInertia(mass, inertiaAddr);")
private static native void calculateLocalInertiaNATIVE(int addr, float mass, int inertiaAddr);
public void setMargin(float margin) {
setMarginNATIVE((int) cPointer, margin);
}
@org.teavm.jso.JSBody(params = {"addr", "margin"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btCollisionShape);jsObj.setMargin(margin);")
private static native void setMarginNATIVE(int addr, float margin);
public float getMargin() {
return getMarginNATIVE((int) cPointer);
}
@org.teavm.jso.JSBody(params = {"addr"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btCollisionShape);var returnedJSObj = jsObj.getMargin();return returnedJSObj;")
private static native float getMarginNATIVE(int addr);
public int getShapeType() {
return getShapeTypeNATIVE((int) cPointer);
}
@org.teavm.jso.JSBody(params = {"addr"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btCollisionShape);var returnedJSObj = jsObj.getShapeType();return returnedJSObj;")
private static native int getShapeTypeNATIVE(int addr);
public void getAabb(btTransform t, btVector3 aabbMin, btVector3 aabbMax) {
getAabbNATIVE((int) cPointer, (int) t.getCPointer(), (int) aabbMin.getCPointer(), (int) aabbMax.getCPointer());
}
@org.teavm.jso.JSBody(params = {"addr", "tAddr", "aabbMinAddr", "aabbMaxAddr"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btCollisionShape);jsObj.getAabb(tAddr, aabbMinAddr, aabbMaxAddr);")
private static native void getAabbNATIVE(int addr, int tAddr, int aabbMinAddr, int aabbMaxAddr);
public void getAabb(Matrix4 tGDX, Vector3 aabbMinGDX, Vector3 aabbMaxGDX) {
btTransform.convert(tGDX, btTransform.TEMP_0);
btTransform t = btTransform.TEMP_0;
btVector3.convert(aabbMinGDX, btVector3.TEMP_0);
btVector3 aabbMin = btVector3.TEMP_0;
btVector3.convert(aabbMaxGDX, btVector3.TEMP_1);
btVector3 aabbMax = btVector3.TEMP_1;
getAabbNATIVE((int) cPointer, (int) t.getCPointer(), (int) aabbMin.getCPointer(), (int) aabbMax.getCPointer());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy