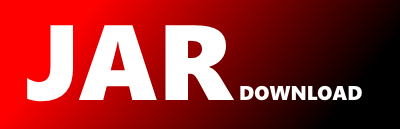
com.badlogic.gdx.physics.bullet.dynamics.btHingeConstraint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gdx-bullet-teavm Show documentation
Show all versions of gdx-bullet-teavm Show documentation
Tool to generate libgdx to javascript using teaVM
/*-------------------------------------------------------
* This file was generated by JParser
*
* Do not make changes to this file
*-------------------------------------------------------*/
package com.badlogic.gdx.physics.bullet.dynamics;
import com.badlogic.gdx.math.Matrix4;
import com.badlogic.gdx.math.Vector3;
import com.badlogic.gdx.physics.bullet.linearmath.btTransform;
import com.badlogic.gdx.physics.bullet.linearmath.btVector3;
/**
* @author xpenatan
*/
public class btHingeConstraint extends btTypedConstraint {
public static btHingeConstraint WRAPPER_GEN_01 = new btHingeConstraint(false);
public btHingeConstraint(btRigidBody rbA, Vector3 pivotInA, Vector3 axisInA) {
btVector3 btpivotInA = btVector3.TEMP_0;
btVector3 btaxisInA = btVector3.TEMP_1;
btVector3.convert(pivotInA, btpivotInA);
btVector3.convert(axisInA, btaxisInA);
initObject(createNative((int) rbA.getCPointer(), (int) btpivotInA.getCPointer(), (int) btaxisInA.getCPointer()), true);
}
public btHingeConstraint(btRigidBody rbA, btRigidBody rbB, Matrix4 rbAFrame, Matrix4 rbBFrame) {
this(rbA, rbB, rbAFrame, rbBFrame, false);
}
public btHingeConstraint(btRigidBody rbA, btRigidBody rbB, Matrix4 rbAFrame, Matrix4 rbBFrame, boolean useReferenceFrameA) {
btTransform btrbAFrame = btTransform.TEMP_0;
btTransform btrbBFrame = btTransform.TEMP_1;
btTransform.convert(rbAFrame, btrbAFrame);
btTransform.convert(rbBFrame, btrbBFrame);
initObject(createNative((int) rbA.getCPointer(), (int) rbB.getCPointer(), (int) btrbAFrame.getCPointer(), (int) btrbBFrame.getCPointer(), useReferenceFrameA), true);
}
@org.teavm.jso.JSBody(params = {"rigidBodyAddr", "pivotInAAddr", "axisInAAddr"}, script = "var jsObj = new Bullet.btHingeConstraint(rigidBodyAddr, pivotInAAddr, axisInAAddr); return Bullet.getPointer(jsObj);")
private static native int createNative(int rigidBodyAddr, int pivotInAAddr, int axisInAAddr);
@org.teavm.jso.JSBody(params = {"rbAAddr", "rbBAddr", "rbAFrameAddr", "rbBFrameAddr", "useReferenceFrameA"}, script = "var jsObj = new Bullet.btHingeConstraint(rbAAddr, rbBAddr, rbAFrameAddr, rbBFrameAddr, useReferenceFrameA); return Bullet.getPointer(jsObj);")
private static native int createNative(int rbAAddr, int rbBAddr, int rbAFrameAddr, int rbBFrameAddr, boolean useReferenceFrameA);
@org.teavm.jso.JSBody(params = {"addr"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btHingeConstraint); Bullet.destroy(jsObj);")
private static native void deleteNative(int addr);
public btHingeConstraint(boolean cMemoryOwn) {
}
public btHingeConstraint() {
}
public void setLimit(float low, float high, float softness, float biasFactor, float relaxationFactor) {
setLimitNATIVE((int) cPointer, low, high, softness, biasFactor, relaxationFactor);
}
@org.teavm.jso.JSBody(params = {"addr", "low", "high", "softness", "biasFactor", "relaxationFactor"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btHingeConstraint);jsObj.setLimit(low, high, softness, biasFactor, relaxationFactor);")
private static native void setLimitNATIVE(int addr, float low, float high, float softness, float biasFactor, float relaxationFactor);
public void setLimit(float low, float high, float softness, float biasFactor) {
setLimitNATIVE((int) cPointer, low, high, softness, biasFactor);
}
@org.teavm.jso.JSBody(params = {"addr", "low", "high", "softness", "biasFactor"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btHingeConstraint);jsObj.setLimit(low, high, softness, biasFactor);")
private static native void setLimitNATIVE(int addr, float low, float high, float softness, float biasFactor);
public void setLimit(float low, float high, float softness) {
setLimitNATIVE((int) cPointer, low, high, softness);
}
@org.teavm.jso.JSBody(params = {"addr", "low", "high", "softness"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btHingeConstraint);jsObj.setLimit(low, high, softness);")
private static native void setLimitNATIVE(int addr, float low, float high, float softness);
public void setLimit(float low, float high) {
setLimitNATIVE((int) cPointer, low, high);
}
@org.teavm.jso.JSBody(params = {"addr", "low", "high"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btHingeConstraint);jsObj.setLimit(low, high);")
private static native void setLimitNATIVE(int addr, float low, float high);
public void enableAngularMotor(boolean enableMotor, float targetVelocity, float maxMotorImpulse) {
enableAngularMotorNATIVE((int) cPointer, enableMotor, targetVelocity, maxMotorImpulse);
}
@org.teavm.jso.JSBody(params = {"addr", "enableMotor", "targetVelocity", "maxMotorImpulse"}, script = "var jsObj = Bullet.wrapPointer(addr, Bullet.btHingeConstraint);jsObj.enableAngularMotor(enableMotor, targetVelocity, maxMotorImpulse);")
private static native void enableAngularMotorNATIVE(int addr, boolean enableMotor, float targetVelocity, float maxMotorImpulse);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy