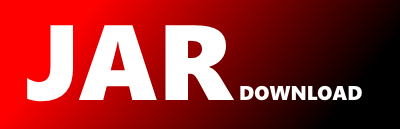
com.github.xphsc.response.ResponseEntity Maven / Gradle / Ivy
package com.github.xphsc.response;
import com.alibaba.fastjson.serializer.SerializeConfig;
import com.alibaba.fastjson.serializer.SerializerFeature;
import com.alibaba.fastjson.serializer.ValueFilter;
import com.github.xphsc.json.JSONHelper;
import com.github.xphsc.json.JSONObject;
import com.github.xphsc.json.JSONValueFilter;
/**
* Created by ${huipei.x} on 2017-5-31.
*/
public class ResponseEntity {
private static SerializeConfig config;
static {
config = new SerializeConfig();
}
static ValueFilter valueFilter=new JSONValueFilter();
private static final SerializerFeature[] features = {
SerializerFeature.WriteDateUseDateFormat,
SerializerFeature.WriteMapNullValue,
SerializerFeature.WriteNullListAsEmpty,
SerializerFeature.WriteNullStringAsEmpty,
SerializerFeature.PrettyFormat,
};
public static String convertResult(Object rows ,int currentPage,int pageSize ,long total)
{
StringBuilder jsonResult = new StringBuilder();
JSONHelper.DEFFAULT_DATE_FORMAT = "yyyy-MM-dd";
jsonResult.append("{");
jsonResult.append("\"status\""+":"+"200");
jsonResult.append(",");
jsonResult.append("\"page\""+":");
jsonResult.append("{");
jsonResult.append("\"currentPage\":" + currentPage+",");
jsonResult.append("\"pageSize\":" + pageSize+",");
jsonResult.append("}");
jsonResult.append(",");
jsonResult.append("\"total\":" + total);
jsonResult.append(",");
jsonResult.append("\"rows\"" + ":" + JSONHelper.toJSONString(rows, valueFilter, features));
jsonResult.append("}");
return jsonResult.toString();
}
public static String convertResult(Object rows ,int currentPage,int pageSize ,long total,long totalPage)
{
StringBuilder jsonResult = new StringBuilder();
JSONHelper.DEFFAULT_DATE_FORMAT = "yyyy-MM-dd";
jsonResult.append("{");
jsonResult.append("\"status\""+":"+"200");
jsonResult.append(",");
jsonResult.append("\"page\""+":");
jsonResult.append("{");
jsonResult.append("\"currentPage\":" + currentPage+",");
jsonResult.append("\"pageSize\":" + pageSize+",");
jsonResult.append("\"totalPage\":" + totalPage);
jsonResult.append("}");
jsonResult.append(",");
jsonResult.append("\"total\":" + total);
jsonResult.append(",");
jsonResult.append("\"rows\"" + ":" + JSONHelper.toJSONString(rows, valueFilter,features));
jsonResult.append("}");
return jsonResult.toString();
}
public static String SuccessResult(Object rows ,long total) {
StringBuilder jsonResult = new StringBuilder();
JSONHelper.DEFFAULT_DATE_FORMAT = "yyyy-MM-dd";
jsonResult.append("{");
jsonResult.append("\"status\""+":"+"200");
jsonResult.append(",");
jsonResult.append("\"msg\"" + ":" +JSONHelper.toJSONString(ResponseStatus.OK.getReasonPhrase()));
jsonResult.append(",");
jsonResult.append("\"total\"" + ":" + total);
jsonResult.append(",");
jsonResult.append("\"rows\"" + ":" + JSONHelper.toJSONString(rows, valueFilter, features));
jsonResult.append("}");
return jsonResult.toString();
}
public static String SuccessResult(Object result,String msg) {
StringBuilder jsonResult = new StringBuilder();
JSONHelper.DEFFAULT_DATE_FORMAT = "yyyy-MM-dd";
jsonResult.append("{");
jsonResult.append("\"status\""+":"+"200");
jsonResult.append(",");
jsonResult.append("\"msg\"" + ":" + JSONHelper.toJSONString(msg));
jsonResult.append(",");
jsonResult.append("\"rows\"" + ":" + JSONHelper.toJSONString(result, valueFilter, features));
jsonResult.append("}");
return jsonResult.toString();
}
public static String SuccessResult(Object result) {
StringBuilder jsonResult = new StringBuilder();
JSONHelper.DEFFAULT_DATE_FORMAT = "yyyy-MM-dd";
jsonResult.append("{");
jsonResult.append("\"status\""+":"+"200");
jsonResult.append(",");
jsonResult.append("\"msg\"" + ":" + JSONHelper.toJSONString(ResponseStatus.OK.getReasonPhrase()));
jsonResult.append(",");
jsonResult.append("\"rows\"" + ":" + JSONHelper.toJSONString(result, valueFilter, features));
jsonResult.append("}");
return jsonResult.toString();
}
public static String SuccessResult() {
JSONObject jsonObject=new JSONObject();
jsonObject.put("status", ResponseStatus.OK.value());
jsonObject.put("msg", ResponseStatus.OK.getReasonPhrase());
return jsonObject.toString();
}
public static String ErrorResult(String msg) {
JSONObject jsonObject=new JSONObject();
jsonObject.put("status", ResponseStatus.BAD_REQUEST.value());
jsonObject.put("msg", msg);
return jsonObject.toString();
}
public static String ErrorResult(int status,Object msg) {
JSONObject jsonObject=new JSONObject();
jsonObject.put("status", status);
jsonObject.put("msg", msg);
return jsonObject.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy