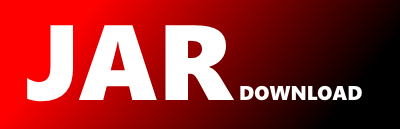
com.github.xphsc.util.MapUtil Maven / Gradle / Ivy
package com.github.xphsc.util;
import org.apache.commons.lang3.ObjectUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.Validate;
import java.beans.BeanInfo;
import java.beans.IntrospectionException;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.*;
/**
* Created by ${huipei.x} on 2017-5-31.
*/
public class MapUtil {
public MapUtil() {
}
public static Map toMap(Object obj) {
HashMap map = null;
if(obj == null) {
return map;
} else {
map = new HashMap();
try {
BeanInfo e = Introspector.getBeanInfo(obj.getClass());
PropertyDescriptor[] propertyDescriptors = e.getPropertyDescriptors();
PropertyDescriptor[] var4 = propertyDescriptors;
int var5 = propertyDescriptors.length;
for(int var6 = 0; var6 < var5; ++var6) {
PropertyDescriptor property = var4[var6];
String key = property.getName();
if(!key.equals("class")) {
Method getter = property.getReadMethod();
Object value = getter.invoke(obj, new Object[0]);
map.put(key, value);
}
}
} catch (Exception var11) {
map = null;
}
return map;
}
}
public static Map bean2MapObject(Object object){
if(object == null){
return null;
}
Map map = new HashMap();
try {
BeanInfo beanInfo = Introspector.getBeanInfo(object.getClass());
PropertyDescriptor[] propertyDescriptors = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor property : propertyDescriptors) {
String key = property.getName();
if (!key.equals("class")) {
Method getter = property.getReadMethod();
Object value = getter.invoke(object);
map.put(key, value);
}
}
} catch (Exception e) {
e.printStackTrace();
}
return map;
}
public static Object map2Bean(Map map,Object object){
if(map == null || object == null){
return null;
}
try {
BeanInfo beanInfo = Introspector.getBeanInfo(object.getClass());
PropertyDescriptor[] propertyDescriptors = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor property : propertyDescriptors) {
String key = property.getName();
if (map.containsKey(key)) {
Object value = map.get(key);
Method setter = property.getWriteMethod();
setter.invoke(object, value);
}
}
} catch (IntrospectionException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
return object;
}
public static Map toStringMap(Object obj) {
HashMap map = null;
if(obj == null) {
return map;
} else {
map = new HashMap();
try {
BeanInfo e = Introspector.getBeanInfo(obj.getClass());
PropertyDescriptor[] propertyDescriptors = e.getPropertyDescriptors();
PropertyDescriptor[] var4 = propertyDescriptors;
int var5 = propertyDescriptors.length;
for(int var6 = 0; var6 < var5; ++var6) {
PropertyDescriptor property = var4[var6];
String key = property.getName();
if(!key.equals("class")) {
Method getter = property.getReadMethod();
map.put(key, getter.invoke(obj, new Object[0]).toString());
}
}
} catch (Exception var10) {
map = null;
}
return map;
}
}
public static int getInt(Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy