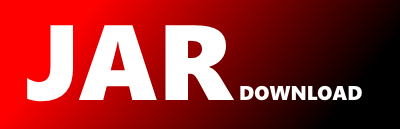
com.github.xphsc.util.BeanUtil Maven / Gradle / Ivy
package com.github.xphsc.util;
import org.apache.commons.beanutils.BeanUtilsBean;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Map;
/**
* Created by ${huipei.x} on 2017-5-25.
*/
public class BeanUtil {
private BeanUtil() {
}
public static boolean isBean(Class> clazz) {
if(ClassUtil.isNormalClass(clazz)) {
Method[] methods = clazz.getMethods();
Method[] arr$ = methods;
int len$ = methods.length;
for(int i$ = 0; i$ < len$; ++i$) {
Method method = arr$[i$];
if(method.getParameterTypes().length == 1 && method.getName().startsWith("set")) {
return true;
}
}
}
return false;
}
public static Object cloneBean(Object bean) throws IllegalAccessException, InstantiationException, InvocationTargetException, NoSuchMethodException {
return BeanUtilsBean.getInstance().cloneBean(bean);
}
public static void copyProperties(Object dest, Object orig) throws IllegalAccessException, InvocationTargetException {
BeanUtilsBean.getInstance().copyProperties(dest, orig);
}
public static void copyProperty(Object bean, String name, Object value) throws IllegalAccessException, InvocationTargetException {
BeanUtilsBean.getInstance().copyProperty(bean, name, value);
}
public static Map describe(Object bean) throws IllegalAccessException, InvocationTargetException, NoSuchMethodException {
return BeanUtilsBean.getInstance().describe(bean);
}
public static String[] getArrayProperty(Object bean, String name) throws IllegalAccessException, InvocationTargetException, NoSuchMethodException {
return BeanUtilsBean.getInstance().getArrayProperty(bean, name);
}
public static String getIndexedProperty(Object bean, String name) throws IllegalAccessException, InvocationTargetException, NoSuchMethodException {
return BeanUtilsBean.getInstance().getIndexedProperty(bean, name);
}
public static String getIndexedProperty(Object bean, String name, int index) throws IllegalAccessException, InvocationTargetException, NoSuchMethodException {
return BeanUtilsBean.getInstance().getIndexedProperty(bean, name, index);
}
public static String getMappedProperty(Object bean, String name) throws IllegalAccessException, InvocationTargetException, NoSuchMethodException {
return BeanUtilsBean.getInstance().getMappedProperty(bean, name);
}
public static String getMappedProperty(Object bean, String name, String key) throws IllegalAccessException, InvocationTargetException, NoSuchMethodException {
return BeanUtilsBean.getInstance().getMappedProperty(bean, name, key);
}
public static String getNestedProperty(Object bean, String name) throws IllegalAccessException, InvocationTargetException, NoSuchMethodException {
return BeanUtilsBean.getInstance().getNestedProperty(bean, name);
}
public static String getProperty(Object bean, String name) throws IllegalAccessException, InvocationTargetException, NoSuchMethodException {
return BeanUtilsBean.getInstance().getProperty(bean, name);
}
public static String getSimpleProperty(Object bean, String name) throws IllegalAccessException, InvocationTargetException, NoSuchMethodException {
return BeanUtilsBean.getInstance().getSimpleProperty(bean, name);
}
public static void populate(Object bean, Map properties) throws IllegalAccessException, InvocationTargetException {
BeanUtilsBean.getInstance().populate(bean, properties);
}
public static void setProperty(Object bean, String name, Object value) throws IllegalAccessException, InvocationTargetException {
BeanUtilsBean.getInstance().setProperty(bean, name, value);
}
public static boolean initCause(Throwable throwable, Throwable cause) {
return BeanUtilsBean.getInstance().initCause(throwable, cause);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy