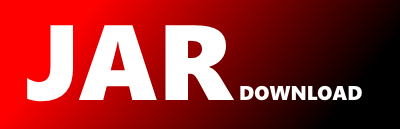
com.github.xphsc.util.DateUtil Maven / Gradle / Ivy
package com.github.xphsc.util;
import com.github.xphsc.date.DateFormat;
import com.github.xphsc.date.DateRegexPattern;
import com.github.xphsc.exception.TimeMatchFormatException;
import java.io.IOException;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.SimpleTimeZone;
/**
* Created by ${huipei.x} on 2017-5-31.
*/
public class DateUtil {
public static final long SEC_MILLIS = 1000L;
public static final long MIN_MILLIS = 60000L;
public static final long HOUR_MILLIS = 3600000L;
public static final long DAY_MILLIS = 86400000L;
public static final long WEEK_MILLIS = 604800000L;
public static final long MONTH_MILLIS = 2592000000L;
public static final long YEAR_MILLIS = 31536000000L;
public static final int SEC = 1;
public static final int MIN_SEC = 60;
public static final int HOUR_SEC = 3600;
public static final int DAY_SEC = 86400;
public static final int WEEK_SE = 604800;
public static final int MONTH_SEC = 2592000;
public static final int YEAR_SEC = 31536000;
public DateUtil() {
}
public static int days(Date time) {
long startTim = time.getTime();
long endTim = System.currentTimeMillis();
long diff = endTim - startTim;
int days = (int)(diff / 1000L / 3600L / 24L);
return days;
}
public static String time(Integer time) {
return time(Long.valueOf((long)time.intValue()));
}
public static String time(Long time) {
long hour = time.longValue() / 3600000L;
time = Long.valueOf(time.longValue() % 3600000L);
long min = time.longValue() / 60000L;
time = Long.valueOf(time.longValue() % 60000L);
long sec = time.longValue() / 1000L;
time = Long.valueOf(time.longValue() % 1000L);
return String.format("%02d:%02d:%02d", new Object[]{Long.valueOf(hour), Long.valueOf(min), Long.valueOf(sec)});
}
public static Date todayStart() {
Calendar cal = Calendar.getInstance();
cal.set(11, 0);
cal.set(13, 0);
cal.set(12, 0);
cal.set(14, 0);
return cal.getTime();
}
public static Date todayEnd() {
Calendar cal = Calendar.getInstance();
cal.set(11, 24);
cal.set(13, 0);
cal.set(12, 0);
cal.set(14, 0);
return cal.getTime();
}
public static Date yesterdayStart() {
Calendar cal = Calendar.getInstance();
cal.add(5, -1);
cal.set(11, 0);
cal.set(13, 0);
cal.set(12, 0);
cal.set(14, 0);
return cal.getTime();
}
public static Date yesterdayEnd() {
Calendar cal = Calendar.getInstance();
cal.set(11, 0);
cal.set(13, 0);
cal.set(12, 0);
cal.set(14, 0);
return cal.getTime();
}
public static Date thisWeekStart() {
Calendar cal = Calendar.getInstance();
cal.set(cal.get(1), cal.get(2), cal.get(5), 0, 0, 0);
cal.set(7, 2);
return cal.getTime();
}
public static Date thisWeekEnd() {
Calendar cal = Calendar.getInstance();
cal.setTime(thisWeekStart());
cal.add(7, 7);
return cal.getTime();
}
public static Date thisMonthStart() {
Calendar cal = Calendar.getInstance();
cal.set(cal.get(1), cal.get(2), cal.get(5), 0, 0, 0);
cal.set(5, cal.getActualMinimum(5));
return cal.getTime();
}
public static Date thisMonthEnd() {
Calendar cal = Calendar.getInstance();
cal.set(cal.get(1), cal.get(2), cal.get(5), 0, 0, 0);
cal.set(5, cal.getActualMaximum(5));
cal.set(11, 24);
return cal.getTime();
}
public static Date thisYearEnd() {
Calendar lastDate = Calendar.getInstance();
int year = lastDate.get(1);
SimpleDateFormat format = new SimpleDateFormat(DateFormat.DATE_FORMAT_SEC );
try {
return format.parse(year + "-12-31 23:59:59");
} catch (ParseException var4) {
return null;
}
}
public static Date thisYearStart() {
Calendar lastDate = Calendar.getInstance();
int year = lastDate.get(1);
SimpleDateFormat format = new SimpleDateFormat(DateFormat.DATE_FORMAT_SEC);
try {
return format.parse(year + "-01-01 00:00:00");
} catch (ParseException var4) {
return null;
}
}
public static Date lastYearEnd() {
Calendar lastDate = Calendar.getInstance();
int year = lastDate.get(1) - 1;
SimpleDateFormat format = new SimpleDateFormat(DateFormat.DATE_FORMAT_SEC);
try {
return format.parse(year + "-12-31 23:59:59");
} catch (ParseException var4) {
return null;
}
}
public static Date lastYearStart() {
Calendar lastDate = Calendar.getInstance();
int year = lastDate.get(1) - 1;
SimpleDateFormat format = new SimpleDateFormat(DateFormat.DATE_FORMAT_SEC);
try {
return format.parse(year + "-01-01 00:00:00");
} catch (ParseException var4) {
return null;
}
}
public static boolean setSystemTime(Date date) {
String osName = System.getProperty("os.name");
try {
String cmd;
SimpleDateFormat dateFormat;
SimpleDateFormat timeFormat;
if(osName.matches("^(?i)Windows.*$")) {
dateFormat = new SimpleDateFormat(DateFormat.DATE_FORMAT_DAY);
timeFormat = new SimpleDateFormat(DateFormat.TIME_FORMAT_SEC);
cmd = String.format("cmd /c time %s", new Object[]{timeFormat.format(date)});
Runtime.getRuntime().exec(cmd);
cmd = String.format("cmd /c date %s", new Object[]{dateFormat.format(date)});
Runtime.getRuntime().exec(cmd);
} else {
dateFormat = new SimpleDateFormat(DateFormat.DATE_FORMAT_DAY_3);
timeFormat = new SimpleDateFormat(DateFormat.TIME_FORMAT_SEC);
cmd = String.format("date -s %s", new Object[]{dateFormat.format(date)});
Runtime.getRuntime().exec(cmd);
cmd = String.format("date -s %s", new Object[]{timeFormat.format(date)});
Runtime.getRuntime().exec(cmd);
}
return true;
} catch (IOException var6) {
return false;
}
}
public static Date converToDateTime(String dateStr) {
SimpleDateFormat sdf = new SimpleDateFormat(DateFormat.DATE_FORMAT_SEC);
try {
return sdf.parse(dateStr);
} catch (Exception e) {
}
return null;
}
public static int getSecondsBetween(Date d1, Date d2) {
Date[] d = new Date[2];
d[0] = d1;
d[1] = d2;
Calendar[] cal = new Calendar[2];
for (int i = 0; i < cal.length; i++) {
cal[i] = Calendar.getInstance();
cal[i].setTime(d[i]);
}
long m = cal[0].getTime().getTime();
long n = cal[1].getTime().getTime();
return (int) ((m - n) / 1000L);
}
//通过生日计算年龄
public static int calCulateForDate(Date dataOfBirth){
Calendar dob = Calendar.getInstance();
dob.setTime(dataOfBirth);
Calendar today = Calendar.getInstance();
int age = today.get(Calendar.YEAR) - dob.get(Calendar.YEAR);
if (today.get(Calendar.MONTH) < dob.get(Calendar.MONTH)) {
age--;
} else if (today.get(Calendar.MONTH) == dob.get(Calendar.MONTH)
&& today.get(Calendar.DAY_OF_MONTH) < dob.get(Calendar.DAY_OF_MONTH)) {
age--;
}
return age;
}
public static Date changeMongodbDate(){
Date date = null;
try {
SimpleDateFormat format = new SimpleDateFormat(DateFormat.DATE_FORMAT_SEC);
String da = format.format(new Date());
format.setCalendar(new GregorianCalendar(new SimpleTimeZone(0, "GMT")));
date = format.parse(da);
} catch (Exception e) {
}
return date;
}
public static String getFriendlyTime(Date dateTime) {
StringBuffer sb = new StringBuffer();
Date current = Calendar.getInstance().getTime();
long diffInSeconds = (current.getTime() - dateTime.getTime()) / 1000;
long sec = (diffInSeconds >= 60 ? diffInSeconds % 60 : diffInSeconds);
long min = (diffInSeconds = (diffInSeconds / 60)) >= 60 ? diffInSeconds % 60 : diffInSeconds;
long hrs = (diffInSeconds = (diffInSeconds / 60)) >= 24 ? diffInSeconds % 24 : diffInSeconds;
long days = (diffInSeconds = (diffInSeconds / 24)) >= 30 ? diffInSeconds % 30 : diffInSeconds;
long months = (diffInSeconds = (diffInSeconds / 30)) >= 12 ? diffInSeconds % 12 : diffInSeconds;
long years = (diffInSeconds = (diffInSeconds / 12));
if (years > 0) {
if (years == 1) {
sb.append("a year");
} else {
sb.append(years + " years");
}
if (years <= 6 && months > 0) {
if (months == 1) {
sb.append(" and a month");
} else {
sb.append(" and " + months + " months");
}
}
} else if (months > 0) {
if (months == 1) {
sb.append("a month");
} else {
sb.append(months + " months");
}
if (months <= 6 && days > 0) {
if (days == 1) {
sb.append(" and a day");
} else {
sb.append(" and " + days + " days");
}
}
} else if (days > 0) {
if (days == 1) {
sb.append("a day");
} else {
sb.append(days + " days");
}
if (days <= 3 && hrs > 0) {
if (hrs == 1) {
sb.append(" and an hour");
} else {
sb.append(" and " + hrs + " hours");
}
}
} else if (hrs > 0) {
if (hrs == 1) {
sb.append("an hour");
} else {
sb.append(hrs + " hours");
}
if (min > 1) {
sb.append(" and " + min + " minutes");
}
} else if (min > 0) {
if (min == 1) {
sb.append("a minute");
} else {
sb.append(min + " minutes");
}
if (sec > 1) {
sb.append(" and " + sec + " seconds");
}
} else {
if (sec <= 1) {
sb.append("about a second");
} else {
sb.append("about " + sec + " seconds");
}
}
sb.append(" ago");
return sb.toString();
}
public static String getIntervalTime(Date dateTime) {
StringBuffer sb = new StringBuffer();
Date current = Calendar.getInstance().getTime();
long diffInSeconds = (current.getTime() - dateTime.getTime()) / 1000;
long sec = (diffInSeconds >= 60 ? diffInSeconds % 60 : diffInSeconds);
long min = (diffInSeconds = (diffInSeconds / 60)) >= 60 ? diffInSeconds % 60 : diffInSeconds;
long hrs = (diffInSeconds = (diffInSeconds / 60)) >= 24 ? diffInSeconds % 24 : diffInSeconds;
long days = (diffInSeconds = (diffInSeconds / 24)) >= 30 ? diffInSeconds % 30 : diffInSeconds;
long months = (diffInSeconds = (diffInSeconds / 30)) >= 12 ? diffInSeconds % 12 : diffInSeconds;
long years = (diffInSeconds = (diffInSeconds / 12));
if (years > 0) {
if (years == 1) {
sb.append("1年");
} else {
sb.append(years + " 年");
}
} else if (months > 0) {
if (months == 1) {
sb.append("1个月");
} else {
sb.append(months + "个月");
}
} else if (days > 0) {
if (days == 1) {
sb.append("1天");
} else {
sb.append(days + "天");
}
} else if (hrs > 0) {
if (hrs == 1) {
sb.append("5小时");
} else {
sb.append(hrs + "小时");
}
} else if (min > 0) {
if (min == 1) {
sb.append("5分钟");
} else {
if(min < 5){
sb.append(5 + "分钟");
}else if(min > 5 && min < 10){
sb.append(10 + "分钟");
}else{
sb.append(min + "分钟");
}
}
} else {
sb.append("1分钟");
}
sb.append("前");
return sb.toString();
}
public static Date modifyDate(int unit,int num){
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date curDate = new Date();
Calendar calendar = Calendar.getInstance();
calendar.setTime(curDate);
Date date = AddAndSubTime(unit,num);
return date;
}
public static Date AddAndSubTime(int currenTime,int num) {
Calendar calendar = Calendar.getInstance();
calendar.add(currenTime, num);
Date startDate = calendar.getTime();
return startDate;
}
public static String date2Str(Date date, String format) {
SimpleDateFormat sdf = new SimpleDateFormat(format);
return sdf.format(date);
}
public static String date2Str(Date date) {
SimpleDateFormat sdf = new SimpleDateFormat(DateFormat.DATE_FORMAT_SEC);
return sdf.format(date);
}
static
public Date str2Date(String strDate, String format) {
Date date = null;
SimpleDateFormat sdf = new SimpleDateFormat(format);
try {
date = sdf.parse(strDate);
} catch (ParseException e) {
e.printStackTrace();
}
return date;
}
@Deprecated
static
public Date str2Date(String strDate) throws TimeMatchFormatException,
ParseException {
strDate = strDate.trim();
SimpleDateFormat sdf = null;
if (RegexUtil.isMatched(strDate, DateRegexPattern.DATE_REG)) {
sdf = new SimpleDateFormat(DateFormat.DATE_FORMAT_DAY);
}
if (RegexUtil.isMatched(strDate, DateRegexPattern.DATE_REG_2)) {
sdf = new SimpleDateFormat(DateFormat.DATE_FORMAT_DAY_2);
}
if (RegexUtil.isMatched(strDate, DateRegexPattern.TIME_SEC_REG)) {
sdf = new SimpleDateFormat(DateFormat.TIME_FORMAT_SEC);
}
if (RegexUtil.isMatched(strDate, DateRegexPattern.DATE_TIME_REG)) {
sdf = new SimpleDateFormat(DateFormat.DATE_FORMAT_SEC);
}
if (RegexUtil.isMatched(strDate, DateRegexPattern.DATE_TIME_MSEC_REG)) {
sdf = new SimpleDateFormat(DateFormat.DATE_FORMAT_MSEC);
}
if (RegexUtil.isMatched(strDate, DateRegexPattern.DATE_TIME_MSEC_T_REG)) {
sdf = new SimpleDateFormat(DateFormat.DATE_FORMAT_MSEC_T);
}
if (RegexUtil.isMatched(strDate, DateRegexPattern.DATE_TIME_MSEC_T_Z_REG)) {
sdf = new SimpleDateFormat(DateFormat.DATE_FORMAT_MSEC_T_Z);
}
if (null != sdf) {
return sdf.parse(strDate);
}
throw new TimeMatchFormatException(
String.format("[%s] can not matching right time format", strDate));
}
static
public Date str2DateUnmatch2Null(String strDate) {
Date date = null;
try {
date = str2Date(strDate);
} catch (Exception e) {
e.printStackTrace();
}
return date;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy