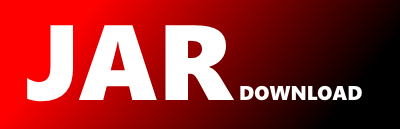
com.github.xphsc.collect.Iterators Maven / Gradle / Ivy
package com.github.xphsc.collect;
import com.github.xphsc.bean.Closure;
import com.github.xphsc.bean.comparator.Transformer;
import com.github.xphsc.collect.iterator.EmptyIterator;
import com.github.xphsc.collect.iterator.ResettableIterator;
import java.util.Iterator;
public class Iterators {
public static boolean isEmpty(Iterator> iterator) {
return iterator == null || !iterator.hasNext();
}
public static void forEach(Iterator iterator, Closure
© 2015 - 2024 Weber Informatics LLC | Privacy Policy