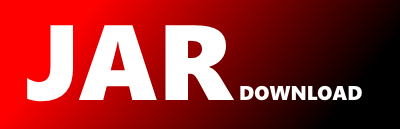
com.github.xphsc.collect.Sorts Maven / Gradle / Ivy
package com.github.xphsc.collect;
import com.github.xphsc.lang.Validator;
import com.github.xphsc.bean.Comparators;
import com.github.xphsc.bean.PropertyComparator;
import com.github.xphsc.bean.comparator.ReverseComparator;
import com.github.xphsc.collect.sort.SortField;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.sql.Timestamp;
import java.util.*;
/**
* Created by ${huipei.x} on 2017-5-31.
*/
public class Sorts {
/**
* 排序list。只需要返回一个可排序的值,例如Integer。
* @param list
* @param sortField
*/
public static > void sortList(List list, final SortField sortField) {
if(list == null || sortField == null) {
return;
}
Collections.sort(list, new Comparator() {
@Override
public int compare(T left, T right) {
boolean isLeftNull = left == null;
boolean isRightNull = right == null;
if (isLeftNull && isRightNull) {
return 0;
} else if (isLeftNull) {
return sortField.isNullFirst() ? -1 : 1;
} else if (isRightNull) {
return sortField.isNullFirst() ? 1 : -1;
}
R comparableLeft = sortField.apply(left);
R comparableRight = sortField.apply(right);
isLeftNull = comparableLeft == null;
isRightNull = comparableRight == null;
if (isLeftNull && isRightNull) {
return 0;
} else if (isLeftNull) {
return sortField.isNullFirst() ? -1 : 1;
} else if (isRightNull) {
return sortField.isNullFirst() ? 1 : -1;
}
return sortField.isAsc() ? comparableLeft.compareTo(comparableRight)
: comparableRight.compareTo(comparableLeft);
}
});
}
public static void sortList(List list, final SortField>... sortFields) {
if(list == null || sortFields == null || sortFields.length == 0) {
return;
}
Collections.sort(list, new Comparator() {
@SuppressWarnings("unchecked")
@Override
public int compare(T left, T right) {
for(SortField> sortField : sortFields) {
boolean isLeftNull = left == null;
boolean isRightNull = right == null;
if(isLeftNull && isRightNull) {
continue;
} else if (isLeftNull) {
return sortField.isNullFirst() ? -1 : 1;
} else if (isRightNull) {
return sortField.isNullFirst() ? 1 : -1;
}
Comparable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy