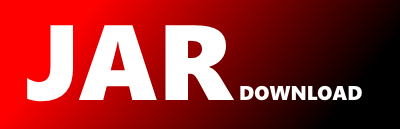
com.github.xphsc.io.ByteArray Maven / Gradle / Ivy
package com.github.xphsc.io;
import com.github.xphsc.lang.Assert;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
/**
* Created by ${huipei.x} on 2017-6-18.
*/
public class ByteArray {
private final byte[] bytes;
private final int offset;
private final int length;
public ByteArray(byte[] bytes) {
this(bytes, 0, Integer.MIN_VALUE);
}
public ByteArray(byte[] bytes, int offset, int length) {
Assert.isNull(bytes, "bytes");
Assert.isTrue(offset >= 0, "offset", offset);
if (length == Integer.MIN_VALUE) {
length = bytes.length - offset;
}
Assert.isTrue(length >= 0 && length <= bytes.length - offset, "length");
this.bytes = bytes;
this.offset = offset;
this.length = length;
}
public byte[] getRawBytes() {
return bytes;
}
public int getOffset() {
return offset;
}
public int getLength() {
return length;
}
public byte[] toByteArray() {
byte[] copy = new byte[length];
System.arraycopy(bytes, offset, copy, 0, length);
return copy;
}
public InputStream toInputStream() {
return new ByteArrayInputStream(bytes, offset, length);
}
public void writeTo(OutputStream out) throws IOException {
out.write(bytes, offset, length);
}
@Override
public String toString() {
return "byte[" + length + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy