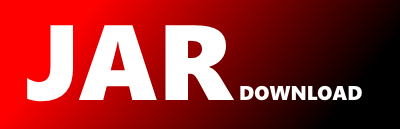
org.yelong.support.redis.jedis.AbstractJedisCacheManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yelong-support Show documentation
Show all versions of yelong-support Show documentation
对各种开源框架的包装、支持、拓展。这里也包含的yelong-core与orm框架的整合。
默认对所有依赖为 scope 为 provided 。您需要针对自己的需要进行再次依赖
package org.yelong.support.redis.jedis;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import org.apache.commons.lang3.ClassUtils;
import org.apache.commons.lang3.StringUtils;
import org.yelong.core.annotation.Nullable;
import org.yelong.core.cache.CacheEntity;
import org.yelong.core.cache.CacheEntitys;
import org.yelong.core.cache.DefaultCacheEntity;
import redis.clients.jedis.Jedis;
/**
* 抽象的jedis缓存管理器
*
* 默认存储为Json
*
* @author PengFei
* @since 1.3.0
*/
public abstract class AbstractJedisCacheManager implements JedisCacheManager {
@Override
public CacheEntity putCache(String key, CacheEntity cacheEntity) {
T entity = cacheEntity.get();
if (null == entity) {
return null;
}
String redisKey = getRedisKey(key);
getJedis().set(redisKey, objectToJson(entity));
getJedis().set(getValueClassNameKey(key), entity.getClass().getName());
return cacheEntity;
}
@Override
public CacheEntity putCache(String key, T entity) {
return putCache(key, new DefaultCacheEntity(entity));
}
@SuppressWarnings("unchecked")
@Override
public CacheEntity getCache(String key) {
String redisKey = getRedisKey(key);
String value = getJedis().get(redisKey);
if (StringUtils.isBlank(value)) {
return CacheEntitys.emptyCacheEntity();
}
String valueClassNameKey = getValueClassNameKey(key);
String valueClassName = getJedis().get(valueClassNameKey);
if (StringUtils.isBlank(valueClassName)) {
throw new RuntimeException("没有找到" + key + "值的类型!");
}
Class> valueClass;
try {
valueClass = ClassUtils.getClass(valueClassName);
} catch (ClassNotFoundException e) {
throw new RuntimeException(e);
}
Object entity = jsonToObject(value, valueClass);
return new DefaultCacheEntity((T) entity);
}
@Override
public Map> getCacheAll() {
Set keys = getKeys();
Map> caches = new HashMap>();
keys.forEach(x -> caches.put(x, getCache(x)));
return caches;
}
@Override
public boolean containsKey(String key) {
String redisKey = getRedisKey(key);
return StringUtils.isNotBlank(getJedis().get(redisKey));
}
@Override
public CacheEntity remove(String key) {
String redisKey = getRedisKey(key);
String valueClassNameKey = getValueClassNameKey(key);
CacheEntity cache = getCache(key);
getJedis().del(redisKey, valueClassNameKey);
return cache;
}
@Override
public void removeQuietly(String key) {
String redisKey = getRedisKey(key);
String valueClassNameKey = getValueClassNameKey(key);
getJedis().del(redisKey, valueClassNameKey);
}
@Override
public Set getKeys() {
String keyPrefix = getKeyPrefix();
if (StringUtils.isBlank(keyPrefix)) {
return getJedis().keys("*");
}
Set keys = getJedis().keys(keyPrefix + "*");
return keys.stream().map(x -> x.substring(keyPrefix.length() + 1)).collect(Collectors.toSet());
}
@Override
public void clear() {
Set keys = getKeys();
keys.forEach(this::remove);
}
/**
* 对象转换为json
*
* @param obj obj
* @return json
*/
protected abstract String objectToJson(Object obj);
/**
* json转换为对象
*
* @param class type
* @param json json
* @param classType class type
* @return 转换后的对象
*/
protected abstract T jsonToObject(String json, Class classType);
/**
* @return jedis
*/
protected abstract Jedis getJedis();
/**
* 键值前缀
*
* 存储在redis中的键值通过添加的缓存键值与键值前缀进行拼接后作为redis的key值
*
* 这不会映射该管理器的其他功能方法
*
* 对于多个Redis缓存管理器,指定不同的键值前缀可以使每个管理器管理不同区域的缓存,防止对Redis中其他的缓存值进行误操作
*
* @return 键值前缀
*/
@Nullable
protected abstract String getKeyPrefix();
/**
* @param key 管理器使用者传入的键值
* @return 包装为Redis中使用的键值
*/
protected abstract String getRedisKey(String key);
/**
* 由于在Redis中存储的json格式的信息,所以需要将存储的对象类型也存储到Redis中
*
* @param key 管理器使用者传入的键值
* @return 值类型的键值
*/
protected abstract String getValueClassNameKey(String key);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy