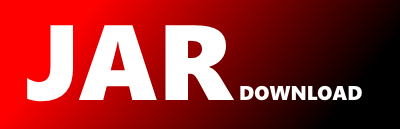
com.github.ygimenez.model.helper.BaseHelper Maven / Gradle / Ivy
package com.github.ygimenez.model.helper;
import com.github.ygimenez.method.Pages;
import com.github.ygimenez.type.Emote;
import net.dv8tion.jda.api.entities.Message;
import net.dv8tion.jda.api.entities.User;
import net.dv8tion.jda.api.interactions.components.Component;
import net.dv8tion.jda.api.interactions.components.LayoutComponent;
import net.dv8tion.jda.api.utils.messages.MessageRequest;
import org.jetbrains.annotations.Nullable;
import java.util.List;
import java.util.concurrent.TimeUnit;
import java.util.function.Predicate;
/**
* Abstract class meant to be extended by builder-like classes, allowing reusability of parameters passed to
* {@link Pages} methods.
*
* @param A class that extends {@link BaseHelper}.
* @param The type of collection used to store pages.
*/
public abstract class BaseHelper, T> {
private final Class subClass;
private final T content;
private final boolean useButtons;
private boolean cancellable = true;
private long time = 0;
private Predicate canInteract = null;
/**
* Constructor for {@link BaseHelper}.
*
* @param subClass A class that extends {@link BaseHelper}.
* @param buttons The collection used to store pages.
* @param useButtons Whether to use interaction buttons or not.
*/
protected BaseHelper(Class subClass, T buttons, boolean useButtons) {
this.subClass = subClass;
this.content = buttons;
this.useButtons = useButtons;
}
/**
* Retrieves the collection used by this helper to store the pages.
*
* @return The underlying collection.
*/
public T getContent() {
return content;
}
/**
* Returns whether the event is configured to use buttons or not.
*
* @return Whether the event is configured to use buttons or not.
*/
public boolean isUsingButtons() {
return useButtons;
}
/**
* Returns whether the {@link Emote#CANCEL} button will be included or not.
*
* @return Whether the event is cancellable or not.
*/
public boolean isCancellable() {
return cancellable;
}
/**
* Set whether the event is cancellable through {@link Emote#CANCEL}.
*
* @param cancellable Whether the event can be cancelled or not (default: true).
* @return The {@link Helper} instance for chaining convenience.
*/
public Helper setCancellable(boolean cancellable) {
this.cancellable = cancellable;
return subClass.cast(this);
}
/**
* Retrieves the timeout for the event, in milliseconds.
*
* @return The timeout for the event.
*/
public long getTimeout() {
return time;
}
/**
* Set the timeout for automatically cancelling the event. Values less than or equal to zero will disable the
* timeout.
*
* @param time The time for the timeout.
* @param unit The unit for the timeout.
* @return The {@link Helper} instance for chaining convenience.
*/
public Helper setTimeout(int time, TimeUnit unit) {
if (unit != null) {
this.time = TimeUnit.MILLISECONDS.convert(time, unit);
}
return subClass.cast(this);
}
/**
* Checks whether the supplied {@link User} can interact with the event.
*
* @param user The {@link User} to check.
* @return Whether the suppied user can interact with the event.
*/
public boolean canInteract(User user) {
return canInteract == null || canInteract.test(user);
}
/**
* Set the condition used to check if a given user can interact with the event buttons.
*
* @param canInteract A {@link Predicate} for checking if a given user can interact with the buttons (default: null).
* @return The {@link Helper} instance for chaining convenience.
*/
public Helper setCanInteract(@Nullable Predicate canInteract) {
this.canInteract = canInteract;
return subClass.cast(this);
}
/**
* Retrieves the {@link List} of {@link Component}s generated by this helper.
*
* @param action A message event (either create or edit).
* @param Generic for a {@link MessageRequest}
* @return The list of components.
*/
public abstract > List getComponents(Out action);
/**
* Prepares the message for being used by the library. This doesn't need to be called manually, this will
* be called during normal flow.
*
* This is no-op when using reaction buttons.
*
* Example:
* {@code helper.apply(channel.sendMessage("Hello world!")).queue();}
*
* @param action A message event (either create or edit).
* @param Generic for a {@link MessageRequest}
* @return The same event, but modified to include the buttons.
*/
public > Out apply(Out action) {
return action.setComponents(getComponents(action));
}
/**
* Calculates whether the {@link Message} needs to have buttons applied onto or not.
*
* @param msg The {@link Message} to be checked.
* @return Whether it needs to be updated or not.
*/
public abstract boolean shouldUpdate(Message msg);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy