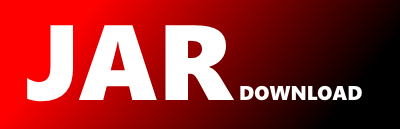
com.github.yin.cli.parsing.LongKeyValueParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-cli-flags Show documentation
Show all versions of java-cli-flags Show documentation
Easy to use command-line parser, which enables you to
define cmdline flags directly the class they are used in.
The newest version!
package com.github.yin.cli.parsing;
import com.google.common.collect.ImmutableMultimap;
import com.google.common.collect.Iterators;
import java.util.Iterator;
/**
* Parses program arguments and recognize long-format cli, each flag name argument must be followed by its value.
* This these restrictions make it easy to build up the command-line from a script. Flag name must start with double
* dash '--' and values may be any string. If any argument does not conform to requirement on its position, or flag is
* not followed by a value, an {@link IllegalArgumentException}} is raised.
*
* Example: program --flag value --input models.txt --input scene.txt
*/
public class LongKeyValueParser {
private final String[] args;
public LongKeyValueParser(String[] args) {
this.args = args;
}
public ImmutableMultimap parse() {
ImmutableMultimap.Builder builder = ImmutableMultimap.builder();
ArgumentReader reader = new ArgumentReader(args);
while(reader.hasNext()) {
String flag = reader.key();
String value = reader.value();
builder.put(flag, value);
}
return builder.build();
}
static class ArgumentReader {
private final Iterator iterator;
public ArgumentReader(String[] args) {
this.iterator = Iterators.forArray(args);
}
public boolean hasNext() {
return iterator.hasNext();
}
public String key() {
String next = iterator.next();
if (next.startsWith("--")) {
return next.substring(2);
} else {
throw new IllegalArgumentException("Argument '" + next + "' is not a flag");
}
}
public String value() {
String next = iterator.next();
return next;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy