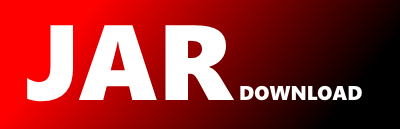
com.github.yin.flags.MapParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-flags Show documentation
Show all versions of java-flags Show documentation
Easy to use command-line parser, which enables you to
define cmdline flags directly the class they are used in.
package com.github.yin.flags;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.Nonnull;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
/**
* Parses a {@link Map} of flags and {@link String} values.
*/
public class MapParser implements Parser
© 2015 - 2025 Weber Informatics LLC | Privacy Policy