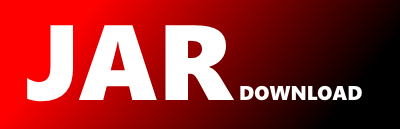
com.github.yiuman.citrus.support.cache.RedisCache Maven / Gradle / Ivy
package com.github.yiuman.citrus.support.cache;
import com.baomidou.mybatisplus.core.toolkit.CollectionUtils;
import org.springframework.data.redis.core.RedisTemplate;
import java.util.*;
/**
* redis缓存
*
* @author yiuman
* @date 2020/6/16
*/
public class RedisCache implements Cache {
private final RedisTemplate redisTemplate;
public RedisCache(RedisTemplate redisTemplate) {
this.redisTemplate = redisTemplate;
}
@Override
public void save(K key, V value) {
redisTemplate.opsForValue().set(key, value);
}
@Override
public V find(K key) {
return redisTemplate.opsForValue().get(key);
}
@Override
public void update(K key, V value) {
this.save(key, value);
}
@Override
public void remove(K key) {
redisTemplate.delete(key);
}
@Override
public boolean contains(K key) {
return redisTemplate.hasKey(key);
}
@SuppressWarnings("unchecked")
@Override
public Collection keys() {
Set keys = redisTemplate.getConnectionFactory().getConnection().keys("*".getBytes());
if (CollectionUtils.isEmpty(keys)) {
return null;
}
final Set ketSet = new HashSet<>(keys.size());
keys.forEach(keyByte -> ketSet.add((K) redisTemplate.getKeySerializer().deserialize(keyByte)));
return ketSet;
}
@Override
public Map map() {
Collection keys = keys();
final Map map = new HashMap<>(keys.size());
keys.forEach(key -> map.put(key, this.find(key)));
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy