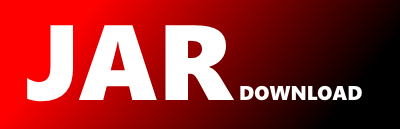
com.github.yiuman.citrus.support.utils.ConvertUtils Maven / Gradle / Ivy
package com.github.yiuman.citrus.support.utils;
import cn.hutool.core.convert.Convert;
import org.springframework.expression.ExpressionParser;
import org.springframework.expression.spel.standard.SpelExpressionParser;
import org.springframework.util.ReflectionUtils;
import java.beans.BeanInfo;
import java.beans.IntrospectionException;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.*;
import java.util.stream.Collectors;
/**
* 转换工具类
*
* @author yiuman
* @date 2020/4/6
*/
public final class ConvertUtils {
private final static ExpressionParser EXPRESSION_PARSER = new SpelExpressionParser();
private ConvertUtils() {
}
public static T parseEl(String el, Class clazz) {
return EXPRESSION_PARSER.parseExpression(el).getValue(clazz);
}
public static Object parseEl(String el) {
return EXPRESSION_PARSER.parseExpression(el).getValue();
}
public static T convert(Class clazz, S source) throws Exception {
T t = clazz.newInstance();
org.springframework.beans.BeanUtils.copyProperties(source, t);
return t;
}
public static List listConvert(Class clazz, Collection source) {
return source.stream().map(LambdaUtils.functionWrapper(item -> convert(clazz, item))).collect(Collectors.toList());
}
/**
* 对象转Map
*
* @param s 对象
* @param 类型
*/
public static Map objectToMap(S s) throws IntrospectionException, InvocationTargetException, IllegalAccessException {
Map map = new HashMap<>();
BeanInfo beanInfo = Introspector.getBeanInfo(s.getClass());
PropertyDescriptor[] pds = beanInfo.getPropertyDescriptors();
for (PropertyDescriptor pd : pds) {
if ("class".equals(pd.getName())) {
continue;
}
Object invokeValue = pd.getReadMethod().invoke(s);
map.put(pd.getDisplayName(), invokeValue == null ? "" : invokeValue);
}
return map;
}
/**
* 枚举转ListMap
*
* @param enumClass 枚举类型
* @param 枚举
* @return List-Map Map中包括枚举类型的所有字段
*/
public static > List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy