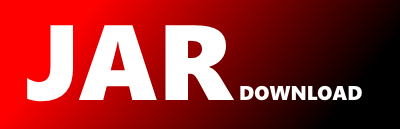
com.github.yiuman.citrus.support.model.Page Maven / Gradle / Ivy
package com.github.yiuman.citrus.support.model;
import com.github.yiuman.citrus.support.crud.view.RecordExtender;
import com.github.yiuman.citrus.support.crud.view.TableView;
import com.github.yiuman.citrus.support.crud.view.impl.SimpleTableView;
import lombok.extern.slf4j.Slf4j;
import org.springframework.util.ObjectUtils;
import org.springframework.util.ReflectionUtils;
import java.lang.reflect.Field;
import java.util.*;
/**
* 分页页面对象
*
* @author yiuman
* @date 2020/5/7
*/
@Slf4j
public class Page extends com.baomidou.mybatisplus.extension.plugins.pagination.Page
implements Key {
/**
* 主键属性名称
*/
private String itemKey;
/**
* 实体的主键属性
*/
private Field keyFiled;
/**
* 记录的扩展属性,key为记录主键值,value则是需要扩展的属性,
*/
private Map> recordExtend;
/**
* 一个视图的描述对象,如表格
*
* @see SimpleTableView
* @see TableView
* @see RecordExtender
*/
private Object view;
public Page() {
}
public String getItemKey() {
return itemKey;
}
public void setItemKey(String itemKey) {
this.itemKey = itemKey;
}
public Map> getRecordExtend() {
return recordExtend;
}
public void setRecordExtend(Map> recordExtend) {
this.recordExtend = recordExtend;
}
@SuppressWarnings("unchecked")
@Override
public List getRecords() {
List records = super.getRecords();
if (this.recordExtend == null
&& !ObjectUtils.isEmpty(itemKey)
&& Objects.nonNull(view)
&& view instanceof RecordExtender) {
this.recordExtend = new HashMap<>(records.size());
RecordExtender recordExtender = (RecordExtender) view;
records.forEach(record -> {
Map result = recordExtender.apply(record);
if (Objects.nonNull(result)) {
recordExtend.put(key(record), result);
}
});
}
return records;
}
public Object getView() {
return view;
}
public void setView(Object view) {
this.view = view;
}
@Override
public String key(T entity) {
try {
if (entity instanceof Map) {
return ((Map, ?>) entity).get(itemKey).toString();
}
keyFiled = Optional.ofNullable(keyFiled)
.orElse(ReflectionUtils.findField(entity.getClass(), itemKey));
if (Objects.isNull(keyFiled)) {
return entity.toString();
}
keyFiled.setAccessible(true);
return keyFiled.get(entity).toString();
} catch (Exception ex) {
log.warn("获取主键异常", ex);
return entity.toString();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy