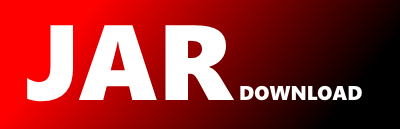
com.github.ykrasik.jaci.util.opt.Opt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaci-utils Show documentation
Show all versions of jaci-utils Show documentation
Java Annotation Command Interface
/******************************************************************************
* Copyright (C) 2015 Yevgeny Krasik *
* *
* Licensed under the Apache License, Version 2.0 (the "License"); *
* you may not use this file except in compliance with the License. *
* You may obtain a copy of the License at *
* *
* http://www.apache.org/licenses/LICENSE-2.0 *
* *
* Unless required by applicable law or agreed to in writing, software *
* distributed under the License is distributed on an "AS IS" BASIS, *
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. *
* See the License for the specific language governing permissions and *
* limitations under the License. *
******************************************************************************/
package com.github.ykrasik.jaci.util.opt;
import com.github.ykrasik.jaci.util.function.Func;
import com.github.ykrasik.jaci.util.function.Pred;
import java.io.Serializable;
import java.util.*;
/**
* Just like Java 8's or Guava's Optional.
* Uses a different name to avoid name confusion.
*
* @param Argument type.
*
* @author Yevgeny Krasik
*/
public abstract class Opt implements Iterable, Serializable {
private static final long serialVersionUID = 0;
protected Opt() { }
/**
* @param {@code Absent} value type.
* @return An empty {@code Opt} instance that contains no value.
*/
public static Opt absent() {
return Absent.instance();
}
/**
* @param value Value to wrap in an {@code Opt}, must be non-null.
* @param Value type.
* @return A non-empty {@code Opt} instance with the given value.
* @throws NullPointerException If value is null.
*/
public static Opt of(T value) {
return new Present<>(Objects.requireNonNull(value, "value"));
}
/**
* @param value Possibly nullable value.
* @param Value type.
* @return A non-empty {@code Opt} if the value is non-null, or an empty one otherwise.
*/
public static Opt ofNullable(T value) {
return (value == null) ? Opt.absent() : new Present<>(value);
}
/**
* Create an {@code Opt} instance out of a {@code Collection}. The collection's size may not be greater than 1.
* Returns a non-empty {@code Opt} with the collection's only element if the collection is non-empty,
* or an empty {@code Opt} otherwise.
*
* @param col Collection to extract an {@code Opt} out of. The collection's size may not be greater than 1.
* @param Collection type.
* @return A non-empty {@code Opt} if the {@code Collection} has a single element, or an empty one otherwise.
* @throws IllegalArgumentException If the collection's size is greater than 1.
*/
public static Opt fromCollection(Collection col) {
if (col.isEmpty()) {
return absent();
}
if (col.size() == 1) {
return of(col.iterator().next());
}
throw new IllegalArgumentException("Cannot create an Opt from a Collection with a size greater then 1!");
}
/**
* @return {@code true} if this {@code Opt} contains a value, otherwise {@code false}.
*/
public abstract boolean isPresent();
/**
* @return This {@code Opt}'s non-null value.
* @throws NoSuchElementException If this {@code Opt} is empty.
*/
public abstract T get();
/**
* @return This {@code Opt}'s value if this {@code Opt} is non-empty, or {@code null} otherwise.
*/
public abstract T getOrElseNull();
/**
* @param defaultValue Default value to return if this {@code Opt} is empty.
* @return This {@code Opt}'s value if this {@code Opt} is non-empty, or {@code defaultValue} otherwise.
*/
public abstract T getOrElse(T defaultValue);
/**
* @param alternative Alternative {@code Opt} to return if this {@code Opt} is empty.
* @return This {@code Opt} if it is non-empty, or the alternative {@code Opt} otherwise.
*/
public abstract Opt orElse(Opt extends T> alternative);
/**
* @param function {@code Function} to apply to this {@code Opt}'s value, if this {@code Opt} is non-empty.
* May return {@code null}, in which case an empty {@code Opt} will be returned.
* @param Returned {@code Opt} type.
* @return An {@code Opt} containing the result of applying {@code function} to this {@code Opt}'s value
* if this {@code Opt} is non-empty, or an empty {@code Opt} otherwise.
*/
public abstract Opt map(Func super T, V> function);
/**
* @param function {@code Function} to apply to this {@code Opt}'s value, if this {@code Opt} is non-empty.
* Different from {@link #map(Func)} in that {@code function} must return an {@code Opt}.
* @param Returned {@code Opt} type.
* @return The {@code Opt} result of applying {@code function} to this {@code Opt}'s value if this {@code Opt} is non-empty,
* or an empty {@code Opt} otherwise.
*/
public abstract Opt flatMap(Func super T, Opt> function);
/**
* @param predicate {@code Predicate} to apply to this {@code Opt}'s value if this {@code Opt} is non-empty.
* @return {@code true} if this {@code Opt} is non-empty and applying the {@code predicate} to this {@code Opt}'s
* value returns {@code true}, otherwise returns {@code false}.
*/
public abstract boolean exists(Pred super T> predicate);
/**
* @param predicate {@code Predicate} to apply to this {@code Opt}'s value if this {@code Opt} is non-empty.
* @return This {@code Opt} if this {@code Opt} is non-empty and applying the predicate to it returns {@code true},
* otherwise returns an empty {@code Opt}.
*/
public abstract Opt filter(Pred super T> predicate);
/**
* @return A singleton {@code List} containing this {@code Opt}'s value if this {@code Opt} is non-empty,
* or an empty {@code List} otherwise.
*/
public abstract List toList();
/**
* @return A singleton {@code Set} containing this {@code Opt}'s value if this {@code Opt} is non-empty,
* or an empty {@code Set} otherwise.
*/
public abstract Set toSet();
/**
* @param key Key to use for mapping if this {@code Opt} is non-empty.
* @param Key type.
* @return A singleton {@code Map} containing a single mapping from the given {@code key} to this {@code Opt}'s value
* if this {@code Opt} is non-empty, or an empty {@code Map} otherwise.
*/
public abstract Map toMap(K key);
@Override
public final Iterator iterator() {
return toList().iterator();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy