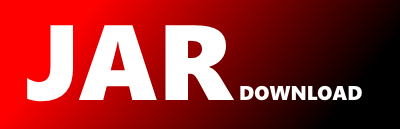
com.paas.aspect.PaaSErrorExceptionHandler Maven / Gradle / Ivy
package com.paas.aspect;
import com.alibaba.fastjson.JSON;
import com.paas.bean.BasicResponse;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.autoconfigure.web.ErrorProperties;
import org.springframework.boot.autoconfigure.web.ResourceProperties;
import org.springframework.boot.autoconfigure.web.reactive.error.DefaultErrorWebExceptionHandler;
import org.springframework.boot.web.reactive.error.ErrorAttributes;
import org.springframework.context.ApplicationContext;
import org.springframework.http.HttpStatus;
import org.springframework.util.StringUtils;
import org.springframework.web.reactive.function.server.*;
import org.springframework.web.server.ResponseStatusException;
import org.springframework.web.server.ServerWebExchange;
import java.net.URI;
import java.util.Map;
/**
* @ClassName PaaSErrorExceptionHandler
* @Date 2020/7/16 11:08
* @Auther wangyongyong
* @Version 1.0
* @Description paas reactive 平台异常处理返回处理
*/
public class PaaSErrorExceptionHandler extends DefaultErrorWebExceptionHandler
{
private static final Logger logger = LoggerFactory.getLogger(RequestAspect.class);
private static final String RESPONSE_BODY = "com.paas.aspect.RequestAspect.responseBody";
/**
* Gateway request URL attribute name.
*/
private static final String GATEWAY_REQUEST_URL_ATTR = "org.springframework.cloud.gateway.support.ServerWebExchangeUtils.gatewayRequestUrl";
/**
* Create a new {@code DefaultErrorWebExceptionHandler} instance.
*
* @param errorAttributes the error attributes
* @param resourceProperties the resources configuration properties
* @param errorProperties the error configuration properties
* @param applicationContext the current application context
*/
public PaaSErrorExceptionHandler(ErrorAttributes errorAttributes, ResourceProperties resourceProperties, ErrorProperties errorProperties, ApplicationContext applicationContext)
{
super(errorAttributes, resourceProperties, errorProperties, applicationContext);
}
@Override
protected RouterFunction getRoutingFunction(ErrorAttributes errorAttributes)
{
return RouterFunctions.route(RequestPredicates.all(), this::renderErrorResponse);
}
/**
* 获取异常属性
*/
@Override
protected Map getErrorAttributes(ServerRequest request, boolean includeStackTrace)
{
ServerWebExchange exchange = request.exchange();
URI requestUrl = exchange.getAttribute(GATEWAY_REQUEST_URL_ATTR);
BasicResponse vo = new BasicResponse();
Exception exception = exchange.getAttribute("org.springframework.boot.web.reactive.error.DefaultErrorAttributes.ERROR");
if (null != exception)
{
if (exception instanceof ResponseStatusException)
{
vo.setStatus(((ResponseStatusException) exception).getStatus().value());
vo.setMessage(exception.getMessage());
}
else
{
vo.setStatus(HttpStatus.SERVICE_UNAVAILABLE.value());
vo.setMessage(HttpStatus.SERVICE_UNAVAILABLE.getReasonPhrase());
String rtnValue = JSON.toJSONString(vo);
if (!StringUtils.isEmpty(requestUrl))
{
logger.info("------ > Call Service | Request Url: " + requestUrl);
logger.info("------ > Call Service | Response Status: " + null);
logger.info("------ > Call Service | Response Content: " + exception.getMessage());
}
logger.error(exception.getMessage(), exception);
exchange.getAttributes().put(RESPONSE_BODY, rtnValue);
return JSON.parseObject(rtnValue, Map.class);
}
}
String rtnValue = JSON.toJSONString(vo);
if (!StringUtils.isEmpty(requestUrl))
{
logger.info("------ > Call Service | Request Url: " + requestUrl);
logger.info("------ > Call Service | Response Status: " + vo.getStatus());
logger.info("------ > Call Service | Response Content: " + rtnValue);
}
exchange.getAttributes().put(RESPONSE_BODY, rtnValue);
return JSON.parseObject(rtnValue, Map.class);
}
@Override
protected int getHttpStatus(Map errorAttributes)
{
return HttpStatus.OK.value();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy