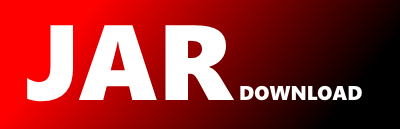
com.paas.aspect.RequestAspect Maven / Gradle / Ivy
package com.paas.aspect;
import com.alibaba.fastjson.JSON;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.annotation.Order;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.Arrays;
import java.util.stream.Collectors;
/**
* @ClassName RequestAspect
* @Description 打印出入参
* Version 0.0.1
**/
@Aspect
@Order(-1)
public class RequestAspect
{
private final Logger logger = LoggerFactory.getLogger(RequestAspect.class);
@Pointcut("@annotation(org.springframework.web.bind.annotation.GetMapping)")
public void getMappingPoint()
{
}
@Pointcut("@annotation(org.springframework.web.bind.annotation.PostMapping)")
public void postMappingPoint()
{
}
@Pointcut("@annotation(org.springframework.web.bind.annotation.RequestMapping) " +
"&& !execution(* org.springframework.boot.autoconfigure.web.servlet.error.BasicErrorController.*(..))")
public void requestMappingPoint()
{
}
@Around("getMappingPoint() || postMappingPoint() || requestMappingPoint()")
public Object around(ProceedingJoinPoint point) throws Throwable
{
long startCurrentTime = System.currentTimeMillis();
logger.info("------ > Req Bind Data: " + ((null == point.getArgs()) ? "null" : Arrays.stream(point.getArgs())
.filter(o -> !(o instanceof HttpServletRequest || o instanceof HttpServletResponse))
.map(JSON::toJSONString).collect(Collectors.joining(" | "))));
Object result = point.proceed();
long endCurrentTime = System.currentTimeMillis();
logger.info("------ > Rsp Bind Data: " + ((null == result) ? "null" : JSON.toJSONString(result)) + " method cost: " + (endCurrentTime - startCurrentTime));
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy