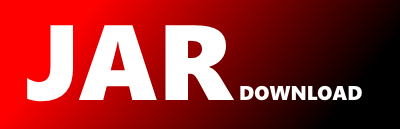
com.github.yoojia.next.lang.Primitives Maven / Gradle / Ivy
package com.github.yoojia.next.lang;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.lang.reflect.Method;
/**
* @author YOOJIA.CHEN ([email protected])
* @version 1.0
*/
public class Primitives {
private static final Logger LOGGER = LoggerFactory.getLogger(Primitives.class);
public static Class> getWrapClass(Class> type){
if(byte.class.equals(type) || Byte.class.equals(type)) return Byte.class;
else if(short.class.equals(type) || Short.class.equals(type)) return Short.class;
else if(int.class.equals(type) || Integer.class.equals(type)) return Integer.class;
else if(long.class.equals(type) || Long.class.equals(type)) return Long.class;
else if(float.class.equals(type) || Float.class.equals(type)) return Float.class;
else if(double.class.equals(type) || Double.class.equals(type)) return Double.class;
else if(char.class.equals(type) || Character.class.equals(type)) return Character.class;
else if(boolean.class.equals(type) || Boolean.class.equals(type)) return Boolean.class;
else if(String.class.equals(type)) return String.class;
else throw new IllegalArgumentException("Not a primitive type: " + type);
}
@SuppressWarnings("unchecked")
public static T valueOf(String value, Class> type){
final Class> wrapType = Primitives.getWrapClass(type);
// 1. String
if(String.class.equals(wrapType)){
return (T) value;
}
// 2. Default value
if(value == null || value.isEmpty()){
value = Boolean.class.equals(wrapType) ? "false" : "0";
}
// 3. Call valueOf(String)
try {
final Method valueOfMethod = wrapType.getMethod("valueOf", String.class);
return (T) valueOfMethod.invoke(wrapType, value);
} catch (NoSuchMethodException e) {
LOGGER.trace("CALL-VALUE-OF", e);
throw new IllegalArgumentException(String.format("Type(%s) WITHOUT static method: valueOf(String) !", wrapType.getSimpleName()));
} catch (Exception e) {
LOGGER.trace("CALL-VALUE-OF", e);
throw new IllegalArgumentException(String.format("Type(%s) fail to invoke method: valueOf(String), Reason: %s",
wrapType.getSimpleName(), Exceptions.message(e)));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy