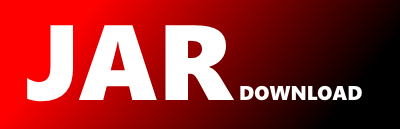
org.yx.common.action.ActInfoUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sumk Show documentation
Show all versions of sumk Show documentation
A quick developing framewort for internet company
/**
* Copyright (C) 2016 - 2030 youtongluan.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.yx.common.action;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.yx.annotation.ExcludeFromParams;
import org.yx.annotation.ExcludeFromResponse;
import org.yx.annotation.spec.ParamSpec;
import org.yx.annotation.spec.Specs;
import org.yx.common.context.CalleeNode;
import org.yx.conf.AppInfo;
import org.yx.log.Logs;
import org.yx.util.S;
import org.yx.util.SumkDate;
import org.yx.validate.FieldParameterHolder;
import org.yx.validate.FieldParameterInfo;
import org.yx.validate.ManuParameterInfo;
import org.yx.validate.ParameterInfo;
import org.yx.validate.Validators;
public final class ActInfoUtil {
public static Object describe(Class> clazz, Class extends Annotation> exclude) {
if (clazz.isArray()) {
return new Object[] { describe(clazz.getComponentType(), exclude) };
}
if (clazz.isPrimitive() || clazz.getName().startsWith("java.") || clazz == SumkDate.class) {
return clazz.getSimpleName();
}
if (Map.class.isAssignableFrom(clazz)) {
return Collections.emptyMap();
}
if (Collection.class.isAssignableFrom(clazz)) {
return Collections.emptyList();
}
if (clazz.isAnnotationPresent(exclude)) {
Logs.http().warn("{}被{}注解了,可能引起一些奇怪的业务反应", clazz.getName(), exclude.getSimpleName());
return null;
}
Map map = new LinkedHashMap<>();
Field[] fs = S.bean().getFields(clazz);
if (AppInfo.getBoolean("sumk.http.act.output.class", false)) {
map.put("$class", clazz.getName());
}
for (Field f : fs) {
if (f.isAnnotationPresent(exclude) || f.getType().isAnnotationPresent(exclude)) {
continue;
}
map.putIfAbsent(f.getName(), describe(f.getType(), exclude));
}
return map;
}
private static Map createMap(String name, CalleeNode node) {
Map map = new LinkedHashMap<>();
map.put("name", name);
map.put("class", node.getDeclaringClass().getName());
map.put("method", node.getMethodName());
map.put("resultType", node.rawMethod().getGenericReturnType().getTypeName());
return map;
}
public static Map simpleInfoMap(String name, CalleeNode node) {
Map map = createMap(name, node);
Map param = new LinkedHashMap<>();
int paramSize = node.argNames() == null ? 0 : node.argNames().size();
Class>[] paramTypes = node.getParameterTypes();
for (int i = 0; i < paramSize; i++) {
Class> paramType = paramTypes[i];
param.put(node.argNames().get(i), describe(paramType, ExcludeFromParams.class));
}
map.put("params", param);
map.put("result", describe(node.getReturnType(), ExcludeFromResponse.class));
return map;
}
public static Map fullInfoMap(String name, CalleeNode node) {
Map map = createMap(name, node);
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy