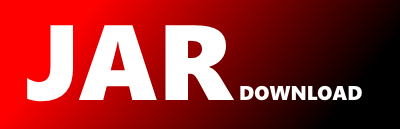
org.yx.db.conn.DBConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sumk Show documentation
Show all versions of sumk Show documentation
A quick developing framewort for internet company
/**
* Copyright (C) 2016 - 2030 youtongluan.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.yx.db.conn;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import org.yx.conf.AppInfo;
import org.yx.db.enums.DBType;
import org.yx.db.sql.DBSettings;
import org.yx.exception.SumkException;
import org.yx.log.Logs;
import org.yx.util.S;
public class DBConfig {
final DBType type;
final int weight;
final int readWeight;
final Map properties;
public DBConfig(DBType type, int weight, int readWeight, Map properties) {
this.type = type;
this.weight = weight;
this.readWeight = readWeight;
this.properties = properties;
}
public String getProperty(String name) {
return this.properties.get(name);
}
public Map getProperties() {
return properties;
}
public DBType getType() {
return type;
}
public int getWeight() {
return weight;
}
public int getReadWeight() {
return readWeight;
}
@Override
public String toString() {
return "DBConfig [type=" + type + ", weight=" + weight + ", read_weight=" + readWeight + ", properties="
+ properties + "]";
}
public static DBConfig create(Map p) throws Exception {
DBType type = DBType.ANY;
int weight = 0, readWeight = 0;
Map properties = new HashMap<>();
Set set = p.keySet();
for (String key : set) {
String v = p.get(key);
if (v == null || v.isEmpty()) {
Logs.db().debug("db config {}={} isempty,ignore it.", key, v);
continue;
}
switch (key.toLowerCase()) {
case "type":
type = DBConfig.parseFromConfigFile(v);
break;
case "weight":
weight = Integer.parseInt(v);
break;
case "password":
if (AppInfo.getBoolean("sumk.db.password.encry", false)) {
byte[] bs = S.base64().decode(v.getBytes());
v = new String(S.cipher().decrypt(bs, DBSettings.getPasswordKey()));
}
properties.put(key, v);
break;
case "read_weight":
case "readweight":
readWeight = Integer.parseInt(v);
break;
default:
properties.put(key, v);
break;
}
}
return new DBConfig(type, weight, readWeight, properties);
}
private static DBType parseFromConfigFile(String type) {
String type2 = type.toLowerCase();
switch (type2) {
case "w":
case "write":
return DBType.WRITE;
case "r":
case "read":
return DBType.READONLY;
case "wr":
case "rw":
case "any":
return DBType.ANY;
default:
throw new SumkException(2342312, type + " is not correct db type");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy