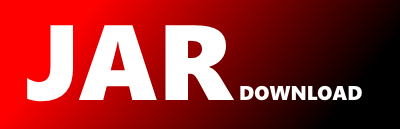
com.lab.dao.BDao Maven / Gradle / Ivy
package com.lab.dao;
import com.lab.model.Company;
import com.github.youyinnn.youdbutils.dao.YouDao;
import com.github.youyinnn.youdbutils.exceptions.NoneffectiveUpdateExecuteException;
import com.github.youyinnn.youdbutils.utils.YouCollectionsUtils;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
/**
*
* @author youyinnn
*
*/
public class BDao extends YouDao {
public void a() throws SQLException {
System.out.println(modelName);
String sql = "SELECT * FROM COMPANY ;";
ResultSet resultSet = modelHandler.executeStatementQuery(sql);
ArrayList arrayList = modelResultFactory.getResultModelList(resultSet);
for (Company o : arrayList) {
System.out.println(o);
}
}
public void b() throws SQLException {
String sql = "SELECT * FROM COMPANY ;";
ResultSet resultSet = modelHandler.executeStatementQuery( sql);
ArrayList arrayList = modelResultFactory.getResultModelList(resultSet);
for (Company o : arrayList) {
System.out.println(o);
}
}
public void c () {
String sql = "INSERT INTO COMPANY (ID,NAME,AGE,ADDRESS,SALARY)" +
"VALUES (3,'paul',22,'California',30000) ;";
String sql1 = "INSERT INTO COMPANY (ID,NAME,AGE,ADDRESS,SALARY)" +
"VALUES (4,'Jame',21,'Norway',50000) ;";
try {
System.out.println(modelHandler.executeStatementInsert(sql));
System.out.println(modelHandler.executeStatementInsert(sql1));
} catch (NoneffectiveUpdateExecuteException e) {
e.printStackTrace();
}
}
public void d (){
String sql = "INSERT INTO COMPANY (ID,NAME,AGE,ADDRESS,SALARY)" +
"VALUES (3,'Jame',21,'Norway',50000) ;";
try {
System.out.println(modelHandler.executeStatementInsert(sql));
} catch (NoneffectiveUpdateExecuteException e) {
e.printStackTrace();
}
}
public void e () {
String sql = "UPDATE company SET name = 'aaa' WHERE id = 55";
try {
System.out.println(modelHandler.executeStatementUpdate(sql));
} catch (NoneffectiveUpdateExecuteException e) {
e.printStackTrace();
}
}
public void f () {
ArrayList all =
modelHandler.getListForAll(YouCollectionsUtils.getYouArrayList("id", "name","address"));
for (Company company : all) {
System.out.println(company);
}
}
public void g(Company company) {
try {
modelHandler.saveModel(company);
Integer id = (Integer) modelHandler.getModelFieldValue("id",
YouCollectionsUtils.getYouHashMap("name",company.getName()),"AND");
System.out.println(id);
} catch (NoneffectiveUpdateExecuteException e) {
e.printStackTrace();
}
}
public void h(HashMap conditionsMap) {
ArrayList listWhereAAndB = modelHandler.getListWhere(conditionsMap, null,"and");
for (Company company : listWhereAAndB) {
System.out.println(company);
}
}
public void i(){
Integer id = (Integer) modelHandler.getModelFieldValue("id",
YouCollectionsUtils.getYouHashMap("name","Jess2"),"AND");
System.out.println(id);
//
//System.out.println(modelHandler.getModel(YouCollectionsUtils.getYouHashMap("name", "Jess33"), null, "AND"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy