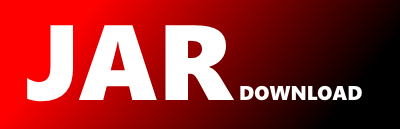
com.bixuebihui.test.dal.HistoryHandlebarsTemplateList Maven / Gradle / Ivy
package com.bixuebihui.test.dal;
/*
* HistoryHandlebarsTemplateList
*
* Notice! Automatically generated file!
* Do not edit the pojo and dal packages,use `maven tablegen:gen`!
* Code Generator originally by J.A.Carter
* Modified by Xing Wanxiang 2008-2021
* email: [email protected]
*/
import java.sql.*;
import java.time.*;
import java.util.List;
import io.r2dbc.spi.ConnectionFactory;
import io.r2dbc.spi.Row;
import io.r2dbc.spi.Connection;
import com.bixuebihui.test.business.*;
import com.bixuebihui.test.pojo.*;
import com.bixuebihui.DbException;
import com.bixuebihui.test.BaseList;
import javax.annotation.processing.Generated;
import reactor.core.publisher.Mono;
@Generated("com.github.yujiaao:tablegen")
public class HistoryHandlebarsTemplateList extends BaseList
{
/**
* Don't direct use the HistoryHandlebarsTemplateList, use HistoryHandlebarsTemplateManager instead.
*/
protected HistoryHandlebarsTemplateList(ConnectionFactory ds)
{
super(ds);
}
@SuppressWarnings("AlibabaClassNamingShouldBeCamel")
public static final class F{
public static final String HISTORY_ID = "history_id";
public static final String TEMPLATE_ID = "template_id";
public static final String TEMPLATE_CODE = "template_code";
public static final String DESCRIPTION = "description";
public static final String DATASOURCE_ID = "datasource_id";
public static final String BASE_TEMPLATE_ID = "base_template_id";
public static final String TEMPLATE_BODY = "template_body";
public static final String OPERATOR_ID = "operator_id";
public static final String VERSION = "version";
public static final String IS_DEL = "is_del";
public static final String CREATED_STIME = "created_stime";
public static final String MODIFIED_STIME = "modified_stime";
public static String[] getAllFields() {
return new String[] { HISTORY_ID, TEMPLATE_ID, TEMPLATE_CODE, DESCRIPTION, DATASOURCE_ID, BASE_TEMPLATE_ID, TEMPLATE_BODY, OPERATOR_ID, VERSION, IS_DEL, CREATED_STIME, MODIFIED_STIME };
}
}
protected String getDeleteSql(){
return "delete from "+this.getTableName()+" where history_id=? ";
}
@Override
protected String getInsertSql(){
return "insert into "+this.getTableName()+" ( template_id ,template_code ,description ,datasource_id ,base_template_id ,template_body ,operator_id ,version ,is_del ,created_stime ,modified_stime ) values ( ? , ? , ? , ? , ? , ? , ? , ? , ? , ? , ? )";
}
@Override
protected String getUpdateSql(){
return "update "+this.getTableName()+" set template_id=?, template_code=?, description=?, datasource_id=?, base_template_id=?, template_body=?, operator_id=?,version= version +1, is_del=?, created_stime=?, modified_stime=? where history_id=?";
}
@Override
protected Object[] getInsertObjs(HistoryHandlebarsTemplate info){
return new Object[]{ info.getTemplateId(),info.getTemplateCode(),info.getDescription(),info.getDatasourceId(),info.getBaseTemplateId(),info.getTemplateBody(),info.getOperatorId(),info.getVersion(),info.getIsDel(),info.getCreatedStime(),info.getModifiedStime() };
}
@Override
protected Object[] getUpdateObjs(HistoryHandlebarsTemplate info){
return new Object[]{ info.getTemplateId(),info.getTemplateCode(),info.getDescription(),info.getDatasourceId(),info.getBaseTemplateId(),info.getTemplateBody(),info.getOperatorId(),info.getIsDel(),info.getCreatedStime(),info.getModifiedStime(),info.getHistoryId() };
}
/**
* Get table name.
*/
@Override
public String getTableName()
{
return "history_handlebars_template";
}
/**
* Get key name.
*/
@Override
public String getKeyName()
{
return F.HISTORY_ID;
}
/**
* Updates the object from a selected ResultSet.
*/
@Override
public HistoryHandlebarsTemplate mapRow(Row r)
{
HistoryHandlebarsTemplate res = new HistoryHandlebarsTemplate();
res.setHistoryId(r.get(F.HISTORY_ID, Integer.class));
res.setTemplateId(r.get(F.TEMPLATE_ID, Integer.class));
res.setTemplateCode(r.get(F.TEMPLATE_CODE, String.class));
res.setDescription(r.get(F.DESCRIPTION, String.class));
res.setDatasourceId(r.get(F.DATASOURCE_ID, Integer.class));
res.setBaseTemplateId(r.get(F.BASE_TEMPLATE_ID, Integer.class));
res.setTemplateBody(r.get(F.TEMPLATE_BODY, String.class));
res.setOperatorId(r.get(F.OPERATOR_ID, Integer.class));
res.setVersion(r.get(F.VERSION, Integer.class));
res.setIsDel(r.get(F.IS_DEL, Integer.class));
res.setCreatedStime(r.get(F.CREATED_STIME, LocalDateTime.class));
res.setModifiedStime(r.get(F.MODIFIED_STIME, LocalDateTime.class));
return res;
}
@Override
public Integer getId(HistoryHandlebarsTemplate info) {
return info.getHistoryId();
}
@Override
public void setId(HistoryHandlebarsTemplate info, Integer id) {
info.setHistoryId(id);
}
@Override
public Mono setIdLong(HistoryHandlebarsTemplate info, Mono id) {
return id.flatMap(it ->{ info.setHistoryId((int)it.longValue()); return Mono.empty();});
}
/**
* Updates the current object values into the database with version condition as an optimistic database lock.
*/
public Mono updateByKeyAndVersion(HistoryHandlebarsTemplate info, Connection cn)
{
return dbHelper.executeNoQuery(getUpdateSql()+" and version=?", new Object[]{
info.getHistoryId(),
info.getTemplateId(),
info.getTemplateCode(),
info.getDescription(),
info.getDatasourceId(),
info.getBaseTemplateId(),
info.getTemplateBody(),
info.getOperatorId(),
info.getIsDel(),
info.getCreatedStime(),
info.getModifiedStime(),
info.getHistoryId(),info.getVersion()
}, cn ).map(x -> x==1);
}
public Mono updateByKeyAndVersion(HistoryHandlebarsTemplate info)
{
return dbHelper.executeNoQuery(getUpdateSql()+" and version=?", new Object[]{
info.getHistoryId(),
info.getTemplateId(),
info.getTemplateCode(),
info.getDescription(),
info.getDatasourceId(),
info.getBaseTemplateId(),
info.getTemplateBody(),
info.getOperatorId(),
info.getIsDel(),
info.getCreatedStime(),
info.getModifiedStime(),
info.getHistoryId(),info.getVersion()
}).map(x -> x==1);
}
/**
* Deletes from the database for table history_handlebars_template with connection
*/
@Override
public Mono deleteByKey( Integer history_id, Connection cn )
{
return dbHelper.executeNoQuery(getDeleteSql(),
new Object[]{ history_id },
cn).map(x -> x>=1);
}
/**
* Deletes from the database for table history_handlebars_template
*/
@Override
public Mono deleteByKey( Integer history_id )
{
return dbHelper.executeNoQuery(getDeleteSql(),
new Object[]{ history_id }).map(x -> x>=1);
}
/**
* Inserts the dummy record of HistoryHandlebarsTemplate object values into the database.
*/
@Override
public Mono insertDummy()
{
HistoryHandlebarsTemplate info = new HistoryHandlebarsTemplate();
java.util.Random rnd = new java.util.Random();
info.setTemplateCode(Integer.toString(Math.abs(rnd.nextInt(Integer.MAX_VALUE)), 36));
info.setDescription(Integer.toString(Math.abs(rnd.nextInt(Integer.MAX_VALUE)), 36));
info.setTemplateBody(Integer.toString(Math.abs(rnd.nextInt(Integer.MAX_VALUE)), 36));
return getNextKey().flatMap(id -> {
setId(info, id);
return Mono.just(info);
}).flatMap(dd -> this.insert(dd));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy