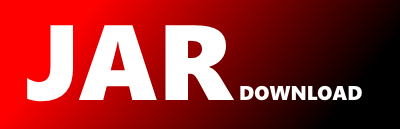
com.github.yulichang.toolkit.MPJReflectionKit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mybatis-plus-join-core Show documentation
Show all versions of mybatis-plus-join-core Show documentation
An enhanced toolkit of Mybatis-Plus to simplify development.
package com.github.yulichang.toolkit;
import com.baomidou.mybatisplus.core.toolkit.Assert;
import com.github.yulichang.toolkit.support.FieldCache;
import org.apache.ibatis.reflection.Reflector;
import java.lang.reflect.Field;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.lang.reflect.WildcardType;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Function;
import java.util.stream.Collectors;
/**
* 反射工具类
*
* @author yulichang
* @since 1.3.7
*/
@SuppressWarnings("unused")
public final class MPJReflectionKit {
private static final Map, FieldStringMap> CLASS_FIELD_CACHE = new ConcurrentHashMap<>();
private static final Map, List> CLASS_FIELD_LIST_CACHE = new ConcurrentHashMap<>();
private static final Map EMPTY_MAP = new HashMap<>();
@Deprecated
@SuppressWarnings("DeprecatedIsStillUsed")
private static final Map, Class>> PRIMITIVE_WRAPPER_TYPE_MAP = new IdentityHashMap<>(8);
@SuppressWarnings("MismatchedQueryAndUpdateOfCollection")
private static final Map, Class>> PRIMITIVE_TYPE_TO_WRAPPER_MAP = new IdentityHashMap<>(8);
static {
PRIMITIVE_WRAPPER_TYPE_MAP.put(Boolean.class, boolean.class);
PRIMITIVE_WRAPPER_TYPE_MAP.put(Byte.class, byte.class);
PRIMITIVE_WRAPPER_TYPE_MAP.put(Character.class, char.class);
PRIMITIVE_WRAPPER_TYPE_MAP.put(Double.class, double.class);
PRIMITIVE_WRAPPER_TYPE_MAP.put(Float.class, float.class);
PRIMITIVE_WRAPPER_TYPE_MAP.put(Integer.class, int.class);
PRIMITIVE_WRAPPER_TYPE_MAP.put(Long.class, long.class);
PRIMITIVE_WRAPPER_TYPE_MAP.put(Short.class, short.class);
PRIMITIVE_WRAPPER_TYPE_MAP.put(String.class, String.class);
for (Map.Entry, Class>> entry : PRIMITIVE_WRAPPER_TYPE_MAP.entrySet()) {
PRIMITIVE_TYPE_TO_WRAPPER_MAP.put(entry.getValue(), entry.getKey());
}
}
/**
* Collection字段的泛型
*/
public static Class> getGenericType(Field field) {
Type type = field.getGenericType();
//没有写泛型
if (!(type instanceof ParameterizedType)) {
return Object.class;
}
ParameterizedType pt = (ParameterizedType) type;
Type[] actualTypeArguments = pt.getActualTypeArguments();
Type argument = actualTypeArguments[0];
//通配符泛型 ? , ? extends XXX , ? super XXX
if (argument instanceof WildcardType) {
//获取上界
Type[] types = ((WildcardType) argument).getUpperBounds();
return (Class>) types[0];
}
return (Class>) argument;
}
/**
* 获取该类的所有属性列表
*
* @param clazz 反射类
*/
public static Map getFieldMap(Class> clazz) {
return CLASS_FIELD_CACHE.computeIfAbsent(clazz, key -> getFieldList(key).stream().collect(Collectors.toMap(f ->
f.getField().getName().toUpperCase(Locale.ENGLISH), Function.identity(), (o, n) -> n, FieldStringMap::new)));
}
public static List getFieldList(Class> clazz) {
if (clazz == null) {
return Collections.emptyList();
}
List fieldList = CLASS_FIELD_LIST_CACHE.get(clazz);
if (fieldList != null) {
return fieldList;
}
List list = ReflectionKit.getFieldList(clazz);
List cache = list.stream().map(f -> {
FieldCache fieldCache = new FieldCache();
fieldCache.setField(f);
try {
Reflector reflector = new Reflector(clazz);
Class> getterType = reflector.getGetterType(f.getName());
fieldCache.setType(Objects.isNull(getterType) ? f.getType() : getterType);
} catch (Throwable throwable) {
fieldCache.setType(f.getType());
}
return fieldCache;
}).collect(Collectors.toList());
CLASS_FIELD_LIST_CACHE.put(clazz, cache);
return cache;
}
public static boolean isPrimitiveOrWrapper(Class> clazz) {
Assert.notNull(clazz, "Class must not be null");
return (clazz.isPrimitive() || PRIMITIVE_WRAPPER_TYPE_MAP.containsKey(clazz));
}
public static T getFieldValue(Object object, String fieldName) {
try {
Field field = object.getClass().getDeclaredField(fieldName);
field.setAccessible(true);
//noinspection unchecked
return (T) field.get(object);
} catch (NoSuchFieldException | IllegalAccessException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy