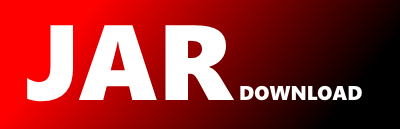
com.github.yulichang.toolkit.sql.SqlScriptUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mybatis-plus-join-core Show documentation
Show all versions of mybatis-plus-join-core Show documentation
An enhanced toolkit of Mybatis-Plus to simplify development.
package com.github.yulichang.toolkit.sql;
import com.baomidou.mybatisplus.core.toolkit.Constants;
import com.baomidou.mybatisplus.core.toolkit.StringUtils;
import org.apache.ibatis.type.JdbcType;
import org.apache.ibatis.type.TypeHandler;
/**
* copy mp 3.4.3 {@link com.baomidou.mybatisplus.core.toolkit.sql.SqlScriptUtils}
*/
@SuppressWarnings("all")
public abstract class SqlScriptUtils implements Constants {
/**
*
* 获取 带 if 标签的脚本
*
*
* @param sqlScript sql 脚本片段
* @return if 脚本
*/
public static String convertIf(final String sqlScript, final String ifTest, boolean newLine) {
String newSqlScript = sqlScript;
if (newLine) {
newSqlScript = NEWLINE + newSqlScript + NEWLINE;
}
return String.format("%s ", ifTest, newSqlScript);
}
/**
*
* 获取 带 trim 标签的脚本
*
*
* @param sqlScript sql 脚本片段
* @param prefix 以...开头
* @param suffix 以...结尾
* @param prefixOverrides 干掉最前一个...
* @param suffixOverrides 干掉最后一个...
* @return trim 脚本
*/
public static String convertTrim(final String sqlScript, final String prefix, final String suffix,
final String prefixOverrides, final String suffixOverrides) {
StringBuilder sb = new StringBuilder("").toString();
}
/**
*
* 生成 choose 标签的脚本
*
*
* @param whenTest when 内 test 的内容
* @param otherwise otherwise 内容
* @return choose 脚本
*/
public static String convertChoose(final String whenTest, final String whenSqlScript, final String otherwise) {
return "" + NEWLINE
+ "" + NEWLINE
+ "" + otherwise + " " + NEWLINE
+ " ";
}
/**
*
* 生成 foreach 标签的脚本
*
*
* @param sqlScript foreach 内部的 sql 脚本
* @param collection collection
* @param index index
* @param item item
* @param separator separator
* @return foreach 脚本
*/
public static String convertForeach(final String sqlScript, final String collection, final String index,
final String item, final String separator) {
StringBuilder sb = new StringBuilder("").toString();
}
/**
*
* 生成 where 标签的脚本
*
*
* @param sqlScript where 内部的 sql 脚本
* @return where 脚本
*/
public static String convertWhere(final String sqlScript) {
return "" + NEWLINE + sqlScript + NEWLINE + " ";
}
/**
*
* 生成 set 标签的脚本
*
*
* @param sqlScript set 内部的 sql 脚本
* @return set 脚本
*/
public static String convertSet(final String sqlScript) {
return "" + NEWLINE + sqlScript + NEWLINE + " ";
}
/**
*
* 安全入参: #{入参}
*
*
* @param param 入参
* @return 脚本
*/
public static String safeParam(final String param) {
return safeParam(param, null);
}
/**
*
* 安全入参: #{入参,mapping}
*
*
* @param param 入参
* @param mapping 映射
* @return 脚本
*/
public static String safeParam(final String param, final String mapping) {
String target = HASH_LEFT_BRACE + param;
if (StringUtils.isBlank(mapping)) {
return target + RIGHT_BRACE;
}
return target + COMMA + mapping + RIGHT_BRACE;
}
/**
*
* 非安全入参: ${入参}
*
*
* @param param 入参
* @return 脚本
*/
public static String unSafeParam(final String param) {
return DOLLAR_LEFT_BRACE + param + RIGHT_BRACE;
}
public static String mappingTypeHandler(Class extends TypeHandler>> typeHandler) {
if (typeHandler != null) {
return "typeHandler=" + typeHandler.getName();
}
return null;
}
public static String mappingJdbcType(JdbcType jdbcType) {
if (jdbcType != null) {
return "jdbcType=" + jdbcType.name();
}
return null;
}
public static String mappingNumericScale(Integer numericScale) {
if (numericScale != null) {
return "numericScale=" + numericScale;
}
return null;
}
public static String convertParamMapping(Class extends TypeHandler>> typeHandler, JdbcType jdbcType, Integer numericScale) {
if (typeHandler == null && jdbcType == null && numericScale == null) {
return null;
}
String mapping = null;
if (typeHandler != null) {
mapping = mappingTypeHandler(typeHandler);
}
if (jdbcType != null) {
mapping = appendMapping(mapping, mappingJdbcType(jdbcType));
}
if (numericScale != null) {
mapping = appendMapping(mapping, mappingNumericScale(numericScale));
}
return mapping;
}
private static String appendMapping(String mapping, String other) {
if (mapping != null) {
return mapping + COMMA + other;
}
return other;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy