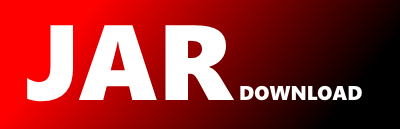
com.github.yulichang.wrapper.resultmap.MybatisLabelFree Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mybatis-plus-join-core Show documentation
Show all versions of mybatis-plus-join-core Show documentation
An enhanced toolkit of Mybatis-Plus to simplify development.
package com.github.yulichang.wrapper.resultmap;
import com.baomidou.mybatisplus.core.metadata.TableInfo;
import com.baomidou.mybatisplus.core.toolkit.Assert;
import com.baomidou.mybatisplus.core.toolkit.CollectionUtils;
import com.baomidou.mybatisplus.core.toolkit.support.SFunction;
import com.github.yulichang.toolkit.LambdaUtils;
import com.github.yulichang.toolkit.MPJReflectionKit;
import com.github.yulichang.toolkit.TableHelper;
import com.github.yulichang.toolkit.support.ColumnCache;
import com.github.yulichang.toolkit.support.FieldCache;
import com.github.yulichang.wrapper.segments.SelectCache;
import lombok.Getter;
import java.util.*;
import java.util.function.Predicate;
/**
* 无泛型约束 实现自由映射
*
* @author yulichang
* @since 1.4.4
*/
@Getter
public class MybatisLabelFree implements Label {
private String property;
private Class> javaType;
private Class ofType;
private List resultList;
/**
* wrapper里面的引用
*/
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy