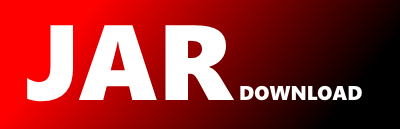
io.magician.tcp.codec.impl.http.HttpProtocolCodec Maven / Gradle / Ivy
package io.magician.tcp.codec.impl.http;
import io.magician.tcp.TCPServerConfig;
import io.magician.tcp.attach.AttachUtil;
import io.magician.tcp.attach.AttachmentModel;
import io.magician.tcp.codec.impl.http.request.MagicianHttpExchange;
import io.magician.tcp.codec.impl.websocket.WebSocketCodec;
import io.magician.tcp.codec.impl.websocket.connection.WebSocketExchange;
import io.magician.tcp.codec.ProtocolCodec;
import io.magician.tcp.workers.Worker;
import io.magician.tcp.codec.impl.http.parsing.HttpMessageParsing;
import io.magician.tcp.codec.impl.http.routing.RoutingParsing;
import java.io.ByteArrayOutputStream;
import java.nio.channels.SelectionKey;
/**
* HTTP协议解析器
*/
public class HttpProtocolCodec implements ProtocolCodec
© 2015 - 2024 Weber Informatics LLC | Privacy Policy