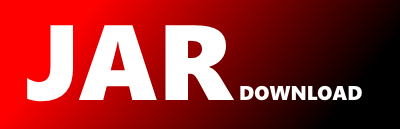
com.yuyenews.resolve.LoadController Maven / Gradle / Ivy
package com.yuyenews.resolve;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.yuyenews.core.annotation.Controller;
import com.yuyenews.core.annotation.EasyMapping;
import com.yuyenews.core.annotation.Resource;
import com.yuyenews.core.model.EasyBeanModel;
import com.yuyenews.easy.server.constant.EasySpace;
import com.yuyenews.resolve.model.EasyMappingModel;
/**
* 加载所有的controller,并完成注入
* @author yuye
*
*/
public class LoadController {
private static Logger log = LoggerFactory.getLogger(LoadController.class);
/**
* 获取全局存储空间
*/
private static EasySpace constants = EasySpace.getEasySpace();
/**
* 创建controller对象,并将服务层对象注入进去
*/
@SuppressWarnings("unchecked")
public static void loadContrl() {
try {
Map controlObjects = new HashMap<>();
/* 获取所有的controller数据 */
Object objs = constants.getAttr("contorls");
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy