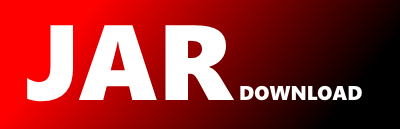
com.mars.mj.helper.JdbcTemplete Maven / Gradle / Ivy
package com.mars.mj.helper;
import com.mars.core.constant.MarsSpace;
import com.mars.core.util.ThreadUtil;
import com.mars.mj.manager.ConnectionManager;
import com.mars.mj.util.DataCheckUtil;
import java.lang.reflect.Field;
import java.sql.Connection;
import java.util.List;
import java.util.Map;
/**
* jdbc模板
*/
public class JdbcTemplete {
private static MarsSpace marsSpace = MarsSpace.getEasySpace();
private String dataSourceName;
private JdbcTemplete() {
}
/**
* 获取JdbcTemplete对象
*
* @return
*/
public static JdbcTemplete get() {
JdbcTemplete jdbcTemplete = new JdbcTemplete();
jdbcTemplete.dataSourceName = jdbcTemplete.getDataSourceName(null);
return jdbcTemplete;
}
/**
* 获取JdbcTemplete对象
*
* @param dataSourceName
* @return
*/
public static JdbcTemplete get(String dataSourceName) {
JdbcTemplete jdbcTemplete = new JdbcTemplete();
jdbcTemplete.dataSourceName = jdbcTemplete.getDataSourceName(dataSourceName);
return jdbcTemplete;
}
/**
* 查询多条数据
*
* @param sql
* @param param
* @return
* @throws Exception
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy