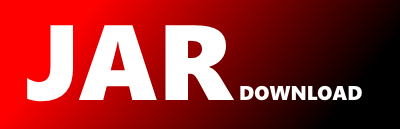
com.mars.mybatis.init.LoadMybatisConfig Maven / Gradle / Ivy
package com.mars.mybatis.init;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.mars.core.constant.MarsConstant;
import com.mars.core.constant.MarsSpace;
import com.mars.core.util.FileUtil;
import com.mars.jdbc.util.JdbcConfigUtil;
import com.mars.mybatis.util.ReadXml;
import com.mars.mybatis.util.extend.MyDataSourceFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
/**
* 组装myBatis配置文件
* @author yuye
*
*/
public class LoadMybatisConfig {
private static Logger logger = LoggerFactory.getLogger(LoadMybatisConfig.class);
private static MarsSpace marsSpace = MarsSpace.getEasySpace();
/**
* 默认配置文件的mapper文件占位符
*/
private static final String MAPPERS = "{mappers}";
/**
* 默认配置文件的数据源占位符
*/
private static final String ENVIRONMENTS ="{environments}";
/**
* 默认配置文件的默认数据源占位符
*/
private static final String DEF = "{def}";
/**
* 获取配置文件并以字符串形式返回
* @return str
*/
public static String getConfigStr() throws Exception {
try {
String localConfig = String.valueOf(JdbcConfigUtil.getJdbcConfig("config-location"));
String str = FileUtil.readFileString("/"+localConfig);
if(str == null) {
logger.warn("自定义mybatis配置文件加载失败或者不存在,将自动使用默认配置");
str = defaultConfig();
str = str.replace(ENVIRONMENTS,getDataSources());
str = str.replace(MAPPERS,getMappers());
} else {
/* 禁止在自定义mybatis配置文件里配置数据源 */
if(str.indexOf("environment") > -1 || str.indexOf("dataSource") > -1 || str.indexOf("environments") > -1) {
throw new Exception("不可以在mybatis配置文件里配置数据源");
}
/* 禁止在自定义mybatis配置文件里配置mappers */
if(str.indexOf("mappers") > -1 || str.indexOf("mapper") > -1) {
throw new Exception("不可以在mybatis配置文件里配置mappers");
}
str = str.replace("","");
str = str + "" + getDataSources() + " ";
str = str + "" + getMappers() + " ";
str = str+"";
}
str = str.replace(DEF, marsSpace.getAttr(MarsConstant.DEFAULT_DATASOURCE_NAME).toString());
return str;
} catch (Exception e) {
throw new Exception("加载mybatis配置出错",e);
}
}
/**
* 获取所有mapper文件路径,并组装成xml格式的字符串返回
* @return str
*/
private static String getMappers() throws Exception {
try {
String mappers = JdbcConfigUtil.getJdbcConfig("mappers").toString();
Set xmls = ReadXml.loadXmlList(mappers);
StringBuffer buffer = new StringBuffer();
for(String str : xmls) {
buffer.append("");
}
return buffer.toString();
} catch (Exception e) {
throw new Exception("加载mybatis配置文件出错",e);
}
}
/**
* 加载数据源配置
* @return str
*/
private static String getDataSources() throws Exception {
try {
String def = "";
JSONArray array = JdbcConfigUtil.getJdbcDataSourceList();
StringBuffer dataSource = new StringBuffer() ;
List daNames = new ArrayList<>();
for (int i = 0; i < array.size(); i++) {
JSONObject jsonObject = array.getJSONObject(i);
ckDsConfig(jsonObject);
if(i == 0) {
def = jsonObject.getString("name");
}
String type = getDataSourceType();
StringBuffer buffer = new StringBuffer();
buffer.append("");
buffer.append("");
buffer.append("");
for(String key : jsonObject.keySet()) {
if(!key.equals("name") && !key.equals("type")) {
buffer.append("");
}
}
buffer.append(" ");
buffer.append(" ");
dataSource.append(buffer);
daNames.add(jsonObject.getString("name"));
}
marsSpace.setAttr(MarsConstant.DATASOURCE_NAMES, daNames);
marsSpace.setAttr(MarsConstant.DEFAULT_DATASOURCE_NAME, def);
return dataSource.toString();
} catch (Exception e) {
throw new Exception("加载mybatis数据源出错",e);
}
}
/**
* 获取数据源类型
* @return str
*/
private static String getDataSourceType() {
return MyDataSourceFactory.class.getName();
}
/**
* 验证数据源配置
* @return str
*/
private static boolean ckDsConfig(JSONObject jsonObject) throws Exception {
if(jsonObject.get("name") == null) {
throw new Exception("数据源没有指定name");
}
return true;
}
/**
* 默认配置
* @return str
*/
private static String defaultConfig() throws Exception {
try {
Object dialect = JdbcConfigUtil.getJdbcConfig("dialect");
if(dialect == null) {
/* 方言 默认mysql */
dialect = "mysql";
}
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("");
stringBuffer.append("");
stringBuffer.append("");
stringBuffer.append("");
stringBuffer.append(" ");
stringBuffer.append(" ");
stringBuffer.append("");
stringBuffer.append("");
stringBuffer.append(" ");
stringBuffer.append(" ");
stringBuffer.append(" ");
stringBuffer.append("");
stringBuffer.append(ENVIRONMENTS);
stringBuffer.append(" ");
stringBuffer.append("");
stringBuffer.append(MAPPERS);
stringBuffer.append(" ");
stringBuffer.append(" ");
return stringBuffer.toString();
} catch (Exception e) {
throw new Exception("加载mybatis配置文件出错",e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy