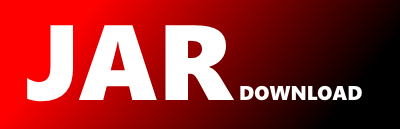
com.github.zeger_tak.enversvalidationplugin.validate.ConfiguredAuditTablesExistValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of envers-validation-maven-plugin Show documentation
Show all versions of envers-validation-maven-plugin Show documentation
This is a Maven plugin that allows for easy validation of database auditing with Hibernate
and is intended to be used by projects that do not always rely on Envers to create the audit history.
package com.github.zeger_tak.enversvalidationplugin.validate;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import javax.annotation.Nonnull;
import com.github.zeger_tak.enversvalidationplugin.annotation.AuditTableInformationMap;
import com.github.zeger_tak.enversvalidationplugin.annotation.ConnectionProvider;
import com.github.zeger_tak.enversvalidationplugin.annotation.Parameterized;
import com.github.zeger_tak.enversvalidationplugin.annotation.TargetPhase;
import com.github.zeger_tak.enversvalidationplugin.annotation.Validate;
import com.github.zeger_tak.enversvalidationplugin.annotation.ValidationType;
import com.github.zeger_tak.enversvalidationplugin.connection.ConnectionProviderInstance;
import com.github.zeger_tak.enversvalidationplugin.entities.AuditTableInformation;
import com.github.zeger_tak.enversvalidationplugin.exceptions.SetupValidationForSpecificAuditTableInformationException;
import org.dbunit.database.CachedResultSetTable;
import org.dbunit.dataset.DataSetException;
@ValidationType(TargetPhase.SETUP)
public class ConfiguredAuditTablesExistValidator
{
private final AuditTableInformation auditTableInformation;
private ConnectionProviderInstance connectionProvider;
public ConfiguredAuditTablesExistValidator(@Nonnull ConnectionProviderInstance connectionProvider, @Nonnull String auditTableName, @Nonnull AuditTableInformation auditTableInformation)
{
this.connectionProvider = connectionProvider;
this.auditTableInformation = auditTableInformation;
}
@Parameterized(name = "{index}: auditTableName: {1}", uniqueIdentifier = "{1}")
public static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy