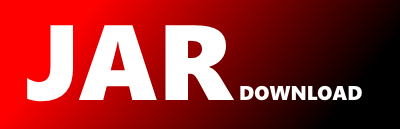
com.github.zella.rxprocess2.IReactiveProcess Maven / Gradle / Ivy
Show all versions of rx-process2 Show documentation
package com.github.zella.rxprocess2;
import io.reactivex.Observable;
import io.reactivex.Single;
import org.reactivestreams.Subscriber;
import java.util.concurrent.TimeUnit;
public interface IReactiveProcess {
/**
* Wait until process exits, Non zero exit code will be captured in {@link Exit}
*
* Subscribe will start process execution
*
* @param timeout timeout
* @param timeUnit timeUnits
* @return Cold Single
*/
Single waitDone(long timeout, TimeUnit timeUnit);
/**
* Wait until process exits with default timeout, Non-zero exit code will be captured in {@link Exit}.
*
* Subscribe will start process execution
*
* No timeout by default. Can be set via system property {@code rxprocess2.timeOutMillis}
*
* @return Cold Single
*/
Single waitDone();
/**
* Process started callback
*
* @return Hot Single
*/
Single started();
/**
* Std input
*
* @return Subscriber allows to push stdin via {@code onNext(byte[] data)} and close stdin via {@code onComplete()}
*/
Subscriber stdIn();
/**
* Real time process stdout/stderr callbacks.
*
*
* @return Hot Observable
*/
Observable stdOutErr();
/**
* Real time process stdout callbacks.
*
* @return Hot Observable
*/
Observable stdOut();
}