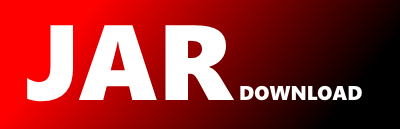
com.github.zh9131101.utils.StringUtils Maven / Gradle / Ivy
/*
* Copyright 2021-2039 ZH9131101.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.zh9131101.utils;
import java.io.ByteArrayOutputStream;
import java.io.UnsupportedEncodingException;
import java.nio.charset.Charset;
import java.util.*;
/**
*
* 字符串 工具类
*
*
* @author zh9131101
* @version V1.0.0
* @date 2021-01-07 22:09
* @since 1.0
*/
public class StringUtils {
private StringUtils() {
}
public static final String[] EMPTY_STRING_ARRAY = {};
public static final String FOLDER_SEPARATOR = "/";
public static final String WINDOWS_FOLDER_SEPARATOR = "\\";
public static final String TOP_PATH = "..";
public static final String CURRENT_PATH = ".";
public static final char EXTENSION_SEPARATOR = '.';
public static final int STRING_BUILDER_SIZE = 256;
/**
* 空格字符的字符串。
*/
public static final String SPACE = " ";
/**
* 空的字符串"" 。
*/
public static final String EMPTY = "";
/**
* 换行符LF(“ \ n”)的字符串。
*/
public static final String LF = "\n";
/**
* 回车CR(“ \ r”)的字符串。
*/
public static final String CR = "\r";
/**
* 表示索引搜索失败。
*/
public static final int INDEX_NOT_FOUND = -1;
/**
* 填充常数可以扩展到的最大大小。
*/
private static final int PAD_LIMIT = 8192;
/* Part of common lang3*/
// Abbreviating
//-----------------------------------------------------------------------
/**
*使用省略号缩写字符串。 这将把“现在是所有好男人的时间”变成“现在是...的时间”。
* 特别:
* 如果str的字符数小于或等于maxWidth ,则返回str 。
* 否则将其缩写为(substring(str, 0, max-3) + "...") 。
* 如果maxWidth小于4 ,则抛出IllegalArgumentException 。
* 在任何情况下都不会返回长度大于maxWidth的String。
*
* @param str 要检查的字符串,可以为null
* @param maxWidth 结果字符串的最大长度,必须至少为4
* @return 简写字符串, null如果空字符串输入
* @throws IllegalArgumentException 如果宽度太小
*/
public static String abbreviate(String str, int maxWidth) {
return abbreviate(str, "...", 0, maxWidth);
}
/**
* 使用省略号缩写字符串。 这会将“现在是所有好男人的时间”变成“ ...是...的时间”
* 与abbreviate(String, int)相似,但允许您指定“左边缘”偏移量。 请注意,此左边缘不一定会成为结果中最左边的字符,也不一定是椭圆后面的第一个字符,但是它将出现在结果中的某个位置。
* 在任何情况下都不会返回长度大于maxWidth的String。
*
*
* @param str 要检查的字符串,可以为null
* @param offset 源字符串的左边缘
* @param maxWidth 结果字符串的最大长度,必须至少为4
* @return 简写字符串, null如果空字符串输入
* @throws IllegalArgumentException 如果宽度太小
*/
public static String abbreviate(String str, int offset, int maxWidth) {
return abbreviate(str, "...", offset, maxWidth);
}
/**
* 使用另一个给定的String作为替换标记来缩写String。 如果将“ ...”定义为替换标记,这会将“现在是所有好男人的时间”变成“现在是...的时间”。
* 特别:
* 如果str的字符数小于或等于maxWidth ,则返回str 。
* 否则将其缩写为(substring(str, 0, max-abbrevMarker.length) + abbrevMarker) 。
* 如果maxWidth小于abbrevMarker.length + 1 ,则抛出IllegalArgumentException 。
* 在任何情况下都不会返回长度大于maxWidth的String。
*
* @param str 要检查的字符串,可以为null
* @param abbrevMarker 用作替换标记的字符串
* @param maxWidth 结果字符串的最大长度,必须至少为abbrevMarker.length + 1
* @return 简写字符串, null如果空字符串输入
* @throws IllegalArgumentException 如果宽度太小
*/
public static String abbreviate(String str, String abbrevMarker, int maxWidth) {
return abbreviate(str, abbrevMarker, 0, maxWidth);
}
/**
* 使用给定的替换标记缩写String。 如果将“ ...”定义为替换标记,这会将“现在是所有好男人的时间”变成“ ...是...的时间”。
* 类似于abbreviate(String, String, int) ,但允许您指定“左边缘”偏移量。 请注意,此左边缘不一定会成为结果中最左边的字符,也不会是替换标记之后的第一个字符,但是它将出现在结果中的某个位置。
* 在任何情况下都不会返回长度大于maxWidth的String。
*
* @param str 要检查的字符串,可以为null
* @param abbrevMarker 用作替换标记的字符串
* @param offset 源字符串的左边缘
* @param maxWidth 结果字符串的最大长度,必须至少为4
* @return 简写字符串, null如果空字符串输入
* @throws IllegalArgumentException 如果宽度太小
*/
public static String abbreviate(String str, String abbrevMarker, int offset, int maxWidth) {
if (isEmpty(str) && isEmpty(abbrevMarker)) {
return str;
} else if (isNotEmpty(str) && EMPTY.equals(abbrevMarker) && maxWidth > 0) {
return str.substring(0, maxWidth);
} else if (isEmpty(str) || isEmpty(abbrevMarker)) {
return str;
}
int abbrevMarkerLength = abbrevMarker.length();
int minAbbrevWidth = abbrevMarkerLength + 1;
int minAbbrevWidthOffset = abbrevMarkerLength + abbrevMarkerLength + 1;
if (maxWidth < minAbbrevWidth) {
throw new IllegalArgumentException(String.format("Minimum abbreviation width is %d", minAbbrevWidth));
}
if (str.length() <= maxWidth) {
return str;
}
if (offset > str.length()) {
offset = str.length();
}
if (str.length() - offset < maxWidth - abbrevMarkerLength) {
offset = str.length() - (maxWidth - abbrevMarkerLength);
}
if (offset <= abbrevMarkerLength+1) {
return str.substring(0, maxWidth - abbrevMarkerLength) + abbrevMarker;
}
if (maxWidth < minAbbrevWidthOffset) {
throw new IllegalArgumentException(String.format("Minimum abbreviation width with offset is %d", minAbbrevWidthOffset));
}
if (offset + maxWidth - abbrevMarkerLength < str.length()) {
return abbrevMarker + abbreviate(str.substring(offset), abbrevMarker, maxWidth - abbrevMarkerLength);
}
return abbrevMarker + str.substring(str.length() - (maxWidth - abbrevMarkerLength));
}
/**
* 将字符串缩写为所传递的长度,用提供的替换字符串替换中间字符。
* 仅在满足以下条件时才会出现此缩写:
* 缩写字符串或替换字符串都不为null或为空
* 要截断的长度小于提供的String的长度
* 要截断的长度大于0
* 缩写的String将有足够的空间容纳所提供的替换字符串的长度以及所提供的String的首个和最后一个字符以供缩写
* 否则,返回的字符串将与提供的缩写字符串相同。
*
* @param str 要缩写的字符串,可以为null
* @param middle 用于替换中间字符的字符串,可以为null
* @param length 缩写为str的长度
* @return 如果满足上述条件,则为缩写字符串,或者提供原始的缩写字符串。
*/
public static String abbreviateMiddle(String str, String middle, int length) {
if (isEmpty(str) || isEmpty(middle)) {
return str;
}
if (length >= str.length() || length < middle.length()+2) {
return str;
}
int targetSting = length-middle.length();
int startOffset = targetSting/2+targetSting%2;
int endOffset = str.length()-targetSting/2;
return str.substring(0, startOffset) +
middle +
str.substring(endOffset);
}
// Compare
//-----------------------------------------------------------------------
/**
* 按照String.compareTo(String)字典上比较两个String.compareTo(String) ,返回:
* 如果str1等于str2 (或者均为null ),则int = 0
* 如果str1小于str2 ,则int < 0
* 如果str1大于str2 ,则int > 0
* 这是的null安全版本:
* str1.compareTo(str2)
* null值被认为小于非null值。 两个null引用被视为相等。
*
* @param str1 要比较的字符串
* @param str2 要比较的字符串
* @return <0、0,> 0,如果str1分别小于,等于或大于str2
*/
public static int compare(String str1, String str2) {
return compare(str1, str2, true);
}
/**
* 按照String.compareTo(String)字典上比较两个String.compareTo(String) ,返回:
* 如果str1等于str2 (或者均为null ),则int = 0
* 如果str1小于str2 ,则int < 0
* 如果str1大于str2 ,则int > 0
* 这是的null安全版本:
* str1.compareTo(str2)
* null输入将根据nullIsLess参数进行处理。 两个null引用被视为相等。
*
* @param str1 要比较的字符串
* @param str2 要比较的字符串
* @param nullIsLess 是否考虑null值小于非null值
* @return <0、0,> 0,如果str1分别小于或等于ou大于str2
*/
public static int compare(String str1, String str2, boolean nullIsLess) {
if (str1 == str2) {
return 0;
}
if (str1 == null) {
return nullIsLess ? -1 : 1;
}
if (str2 == null) {
return nullIsLess ? 1 : - 1;
}
return str1.compareTo(str2);
}
/**
* 按照String.compareToIgnoreCase(String)字典上比较两个String,忽略大小写差异,返回:
* 如果str1等于str2 (或者均为null ),则int = 0
* 如果str1小于str2 ,则int < 0
* 如果str1大于str2 ,则int > 0
* 这是的null安全版本:
* str1.compareToIgnoreCase(str2)
* null值被认为小于非null值。 两个null引用被视为相等。 比较不区分大小写。
*
* @param str1 要比较的字符串
* @param str2 要比较的字符串
* @return <0、0,> 0,如果str1分别小于,等于ou或大于str2 ,则忽略大小写差异。
*/
public static int compareIgnoreCase(String str1, String str2) {
return compareIgnoreCase(str1, str2, true);
}
/**
* 按照String.compareToIgnoreCase(String)字典上比较两个String,忽略大小写差异,返回:
* 如果str1等于str2 (或者均为null ),则int = 0
* 如果str1小于str2 ,则int < 0
* 如果str1大于str2 ,则int > 0
* 这是的null安全版本:
* str1.compareToIgnoreCase(str2)
* null输入将根据nullIsLess参数进行处理。 两个null引用被视为相等。 比较不区分大小写。
*
* @param str1 要比较的字符串
* @param str2 要比较的字符串
* @param nullIsLess 是否考虑null值小于非null值
* @return <0、0,> 0,如果str1分别小于,等于ou或大于str2 ,则忽略大小写差异。
*/
public static int compareIgnoreCase(String str1, String str2, boolean nullIsLess) {
if (str1 == str2) {
return 0;
}
if (str1 == null) {
return nullIsLess ? -1 : 1;
}
if (str2 == null) {
return nullIsLess ? 1 : - 1;
}
return str1.compareToIgnoreCase(str2);
}
// ContainsNone
//-----------------------------------------------------------------------
/**
* 检查CharSequence是否不包含某些字符。
* null CharSequence将返回true 。 null无效字符数组将返回true 。 空的CharSequence(length()= 0)始终返回true。
*
* @param cs 要检查的CharSequence,可以为null
* @param searchChars 无效字符数组,可以为null
* @return 如果不包含任何无效字符,则为true;否则为null
*/
public static boolean containsNone(CharSequence cs, char... searchChars) {
if (cs == null || searchChars == null) {
return true;
}
int csLen = cs.length();
int csLast = csLen - 1;
int searchLen = searchChars.length;
int searchLast = searchLen - 1;
for (int i = 0; i < csLen; i++) {
char ch = cs.charAt(i);
for (int j = 0; j < searchLen; j++) {
if (searchChars[j] == ch) {
if (Character.isHighSurrogate(ch)) {
if (j == searchLast) {
// missing low surrogate, fine, like String.indexOf(String)
return false;
}
if (i < csLast && searchChars[j + 1] == cs.charAt(i + 1)) {
return false;
}
} else {
// ch is in the Basic Multilingual Plane
return false;
}
}
}
}
return true;
}
/**
* 检查CharSequence是否不包含某些字符。
* null CharSequence将返回true 。 null无效字符数组将返回true 。 空的字符串(“”)始终返回true。
*
* @param cs 要检查的CharSequence,可以为null
* @param invalidChars 无效字符的字符串,可以为null
* @return 如果不包含任何无效字符,则为true;否则为null
*/
public static boolean containsNone(CharSequence cs, String invalidChars) {
if (cs == null || invalidChars == null) {
return true;
}
return containsNone(cs, invalidChars.toCharArray());
}
// ContainsOnly
//-----------------------------------------------------------------------
private static void convertRemainingAccentCharacters(StringBuilder decomposed) {
for (int i = 0; i < decomposed.length(); i++) {
if (decomposed.charAt(i) == '\u0141') {
decomposed.deleteCharAt(i);
decomposed.insert(i, 'L');
} else if (decomposed.charAt(i) == '\u0142') {
decomposed.deleteCharAt(i);
decomposed.insert(i, 'l');
}
}
}
/**
* 计算char在给定字符串中出现多少次。
* null或空(“”)字符串输入返回0 。
*
* @param str 要检查的CharSequence,可以为null
* @param ch 要计数的字符
* @return 出现的次数,如果CharSequence为null ,则为0
*/
public static int countMatches(CharSequence str, char ch) {
if (isEmpty(str)) {
return 0;
}
int count = 0;
// We could also call str.toCharArray() for faster look ups but that would generate more garbage.
for (int i = 0; i < str.length(); i++) {
if (ch == str.charAt(i)) {
count++;
}
}
return count;
}
// Count matches
/**
* 返回传入的CharSequence,或者如果CharSequence为空或null ,则返回defaultStr的值。
*
* @param CharSequence的特定种类
* @param str 要检查的CharSequence,可以为null
* @param defaultStr 如果输入为空(“”)或null ,则返回的默认CharSequence,可以为null
* @return 传入CharSequence或默认值
*/
public static T defaultIfEmpty(T str, T defaultStr) {
return isEmpty(str) ? defaultStr : str;
}
/**
* 返回传入的String,或者如果String为null ,则返回一个空String(“”)。
*
* @param str 要检查的字符串,可以为null
* @return 传入的字符串,如果为null ,则返回空字符串
*/
public static String defaultString(String str) {
return defaultString(str, EMPTY);
}
/**
* 返回传入的String,或者如果String为null ,则返回defaultStr的值。
*
* @param str 要检查的字符串,可以为null
* @param defaultStr 如果输入为null ,则返回的默认String,可以为null
* @return 传入的String,如果为null则为默认null
*/
public static String defaultString(String str, String defaultStr) {
return str == null ? defaultStr : str;
}
// Delete
//-----------------------------------------------------------------------
/**
* 从Character.isWhitespace(char)定义的String中删除所有空格。
*
* @param str 要从中删除空格的字符串,可以为null
* @return 没有空格的字符串, null如果空字符串输入
*/
public static String deleteWhitespace(String str) {
if (isEmpty(str)) {
return str;
}
int sz = str.length();
char[] chs = new char[sz];
int count = 0;
for (int i = 0; i < sz; i++) {
if (!Character.isWhitespace(str.charAt(i))) {
chs[count++] = str.charAt(i);
}
}
if (count == sz) {
return str;
}
return new String(chs, 0, count);
}
// Difference
//-----------------------------------------------------------------------
/**
* 比较两个字符串,并返回它们不同的部分。 更准确地说,从第二个String与第一个String不同的地方开始,返回其余的String。 这意味着“ abc”和“ ab”之间的区别是空字符串而不是“ c”。
* 例如, difference("i am a machine", "i am a robot") -> "robot" 。
*
* @param str1 第一个String,可以为null
* @param str2 第二个String,可以为null
* @return str2与str1不同的部分; 返回
*/
public static String difference(String str1, String str2) {
if (str1 == null) {
return str2;
}
if (str2 == null) {
return str1;
}
int at = indexOfDifference(str1, str2);
if (at == INDEX_NOT_FOUND) {
return EMPTY;
}
return str2.substring(at);
}
// Equals
//-----------------------------------------------------------------------
/**
* 比较两个CharSequences,如果它们表示相等的字符序列,则返回true 。
* null的处理没有例外。 两个null引用被认为是相等的。 比较是区分大小写的。
*
* @param cs1 第一个CharSequence,可以为null
* @param cs2 第二个CharSequence,可以为null
* @return 如果CharSequences相等(区分大小写),则为true ,或者两者都为null
*/
public static boolean equals(CharSequence cs1, CharSequence cs2) {
if (cs1 == cs2) {
return true;
}
if (cs1 == null || cs2 == null) {
return false;
}
if (cs1.length() != cs2.length()) {
return false;
}
if (cs1 instanceof String && cs2 instanceof String) {
return cs1.equals(cs2);
}
// Step-wise comparison
int length = cs1.length();
for (int i = 0; i < length; i++) {
if (cs1.charAt(i) != cs2.charAt(i)) {
return false;
}
}
return true;
}
/**
* 返回数组中不为空的第一个值。
* 如果所有值都为空,或者数组为null或空,则返回null 。
*
* @param CharSequence的特定种类
* @param values 要测试的值,可以为null或为空
* @return 从所述第一值values ,其不为空,或者null ,如果没有非空值
*/
@SafeVarargs
public static T firstNonEmpty(T... values) {
if (values != null) {
for (T val : values) {
if (isNotEmpty(val)) {
return val;
}
}
}
return null;
}
/**
* 检查String str包含Unicode数字,如果是,则连接str所有数字并将其作为String返回。
* 如果在str找不到数字,则将返回一个空(“”)字符串。
*
* @param str 要从中提取数字的字符串,可以为null
* @return 仅包含数字的字符串,如果没有数字,则为空(“”)字符串,或者为null如果str为null
*/
public static String getDigits(String str) {
if (isEmpty(str)) {
return str;
}
int sz = str.length();
StringBuilder strDigits = new StringBuilder(sz);
for (int i = 0; i < sz; i++) {
char tempChar = str.charAt(i);
if (Character.isDigit(tempChar)) {
strDigits.append(tempChar);
}
}
return strDigits.toString();
}
/**
* 比较两个CharSequences,并返回CharSequences开始不同的索引。
* 例如, indexOfDifference("i am a machine", "i am a robot") -> 7
*
* @param cs1 第一个CharSequence,可以为null
* @param cs2 第二个CharSequence,可以为null
* @return cs1和cs2开始不同的索引; 如果它们相等,则为-1
*/
public static int indexOfDifference(CharSequence cs1, CharSequence cs2) {
if (cs1 == cs2) {
return INDEX_NOT_FOUND;
}
if (cs1 == null || cs2 == null) {
return 0;
}
int i;
for (i = 0; i < cs1.length() && i < cs2.length(); ++i) {
if (cs1.charAt(i) != cs2.charAt(i)) {
break;
}
}
if (i < cs2.length() || i < cs1.length()) {
return i;
}
return INDEX_NOT_FOUND;
}
/**
* 检查CharSequence是否仅包含小写字符。
* null将返回false 。 空的CharSequence(length()= 0)将返回false 。
*
* @param cs 要检查的CharSequence,可以为null
* @return {如果仅包含小写字符并且为非null,则为true
*/
public static boolean isAllLowerCase(CharSequence cs) {
if (isEmpty(cs)) {
return false;
}
int sz = cs.length();
for (int i = 0; i < sz; i++) {
if (!Character.isLowerCase(cs.charAt(i))) {
return false;
}
}
return true;
}
/**
* 检查CharSequence是否仅包含大写字符。
* null将返回false 。 空的String(length()= 0)将返回false 。
*
* @param cs 要检查的CharSequence,可以为null
* @return 如果仅包含大写字符且为非null,则为true
*/
public static boolean isAllUpperCase(CharSequence cs) {
if (isEmpty(cs)) {
return false;
}
int sz = cs.length();
for (int i = 0; i < sz; i++) {
if (!Character.isUpperCase(cs.charAt(i))) {
return false;
}
}
return true;
}
// Character Tests
//-----------------------------------------------------------------------
/**
* 检查CharSequence是否仅包含Unicode字母。
* null将返回false 。 空的CharSequence(length()= 0)将返回false 。
*
* @param cs 要检查的CharSequence,可以为null
* @return 如果仅包含字母并且为非null,则为true
*/
public static boolean isAlpha(CharSequence cs) {
if (isEmpty(cs)) {
return false;
}
int sz = cs.length();
for (int i = 0; i < sz; i++) {
if (!Character.isLetter(cs.charAt(i))) {
return false;
}
}
return true;
}
/**
* 检查CharSequence是否仅包含Unicode字母或数字。
* null将返回false 。 空的CharSequence(length()= 0)将返回false 。
*
* @param cs 要检查的CharSequence,可以为null
* @return 如果仅包含字母或数字,并且为非null,则为true
*/
public static boolean isAlphanumeric(CharSequence cs) {
if (isEmpty(cs)) {
return false;
}
int sz = cs.length();
for (int i = 0; i < sz; i++) {
if (!Character.isLetterOrDigit(cs.charAt(i))) {
return false;
}
}
return true;
}
/**
* 检查CharSequence是否仅包含Unicode字母,数字或空格( ' ' )。
* null将返回false 。 空的CharSequence(length()= 0)将返回true 。
*
* @param cs 要检查的CharSequence,可以为null
* @return 如果仅包含字母,数字或空格,并且为非null,则为true
*/
public static boolean isAlphanumericSpace(CharSequence cs) {
if (cs == null) {
return false;
}
int sz = cs.length();
for (int i = 0; i < sz; i++) {
if (!Character.isLetterOrDigit(cs.charAt(i)) && cs.charAt(i) != ' ') {
return false;
}
}
return true;
}
/**
* 检查CharSequence是否仅包含Unicode字母和空格('')。
* null将返回false 。空CharSequence(length()= 0)将返回true 。
*
* @param cs 要检查的CharSequence,可以为null
* @return 如果仅包含字母和空格,并且为非null,则为true
*/
public static boolean isAlphaSpace(CharSequence cs) {
if (cs == null) {
return false;
}
int sz = cs.length();
for (int i = 0; i < sz; i++) {
if (!Character.isLetter(cs.charAt(i)) && cs.charAt(i) != ' ') {
return false;
}
}
return true;
}
/**
* 检查CharSequence是否包含大小写混合的大小写。
* null将返回false 。 空的CharSequence( length()=0 )将返回false 。
*
* @param cs 要检查的CharSequence,可以为null
* @return 如果CharSequence同时包含大写和小写字符,则为true
*/
public static boolean isMixedCase(CharSequence cs) {
if (isEmpty(cs) || cs.length() == 1) {
return false;
}
boolean containsUppercase = false;
boolean containsLowercase = false;
int sz = cs.length();
for (int i = 0; i < sz; i++) {
if (containsUppercase && containsLowercase) {
return true;
} else if (Character.isUpperCase(cs.charAt(i))) {
containsUppercase = true;
} else if (Character.isLowerCase(cs.charAt(i))) {
containsLowercase = true;
}
}
return containsUppercase && containsLowercase;
}
/**
*检查CharSequence是否不为空(“”)并且不为null。
*
* @param cs 要检查的CharSequence,可以为null
* @return 如果CharSequence不为空且不为null,则返回true
*/
public static boolean isNotEmpty(CharSequence cs) {
return !isEmpty(cs);
}
/**
* 检查CharSequence是否仅包含Unicode数字。 小数点不是Unicode数字,并且返回false。
* null将返回false 。 空的CharSequence(length()= 0)将返回false 。
* 请注意,该方法不允许使用正号或负号的前导符号。 另外,如果字符串通过数字测试,则在由Integer.parseInt或Long.parseLong解析时,例如,如果值分别超出int或long的范围,则它仍可能生成NumberFormatException。
*
* @param cs 要检查的CharSequence,可以为null
* @return 如果仅包含数字并且为非null,则为true
*/
public static boolean isNumeric(CharSequence cs) {
if (isEmpty(cs)) {
return false;
}
int sz = cs.length();
for (int i = 0; i < sz; i++) {
if (!Character.isDigit(cs.charAt(i))) {
return false;
}
}
return true;
}
/**
* 检查CharSequence是否仅包含Unicode数字或空格( ' ' )。 小数点不是Unicode数字,并且返回false。
* null将返回false 。 空的CharSequence(length()= 0)将返回true 。
*
* @param cs 要检查的CharSequence,可以为null
* @return 如果仅包含数字或空格并且为非null,则为true
*/
public static boolean isNumericSpace(CharSequence cs) {
if (cs == null) {
return false;
}
int sz = cs.length();
for (int i = 0; i < sz; i++) {
if (!Character.isDigit(cs.charAt(i)) && cs.charAt(i) != ' ') {
return false;
}
}
return true;
}
/**
* 检查CharSequence是否仅包含空格。
* 空格由Character.isWhitespace(char)定义。
* null将返回false 。 空的CharSequence(length()= 0)将返回true 。
*
* @param cs 要检查的CharSequence,可以为null
* @return 如果仅包含空格并且为非null,则为true
*/
public static boolean isWhitespace(CharSequence cs) {
if (cs == null) {
return false;
}
int sz = cs.length();
for (int i = 0; i < sz; i++) {
if (!Character.isWhitespace(cs.charAt(i))) {
return false;
}
}
return true;
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @return 接合的字符串, null如果空数组输入
*/
public static String join(byte[] array, char separator) {
if (array == null) {
return null;
}
return join(array, separator, 0, array.length);
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @return 接合的字符串, null如果空数组输入
*/
public static String join(char[] array, char separator) {
if (array == null) {
return null;
}
return join(array, separator, 0, array.length);
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @param startIndex 第一个开始加入的索引。 将起始索引传递到数组的末尾是错误的
* @param endIndex 停止从其开始的索引(不包括)。 将结束索引传递到数组的末尾是错误的
* @return 接合的字符串, null如果空数组输入
*/
public static String join(char[] array, char separator, int startIndex, int endIndex) {
if (array == null) {
return null;
}
int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
StringBuilder buf = new StringBuilder(noOfItems);
buf.append(array[startIndex]);
for (int i = startIndex + 1; i < endIndex; i++) {
buf.append(separator);
buf.append(array[i]);
}
return buf.toString();
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @return 接合的字符串, null如果空数组输入
*/
public static String join(double[] array, char separator) {
if (array == null) {
return null;
}
return join(array, separator, 0, array.length);
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @param startIndex 第一个开始加入的索引。 将起始索引传递到数组的末尾是错误的
* @param endIndex 停止从其开始的索引(不包括)。 将结束索引传递到数组的末尾是错误的
* @return 接合的字符串, null如果空数组输入
*/
public static String join(double[] array, char separator, int startIndex, int endIndex) {
if (array == null) {
return null;
}
int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
StringBuilder buf = new StringBuilder(noOfItems);
buf.append(array[startIndex]);
for (int i = startIndex + 1; i < endIndex; i++) {
buf.append(separator);
buf.append(array[i]);
}
return buf.toString();
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @return 接合的字符串, null如果空数组输入
*/
public static String join(float[] array, char separator) {
if (array == null) {
return null;
}
return join(array, separator, 0, array.length);
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @param startIndex 第一个开始加入的索引。 将起始索引传递到数组的末尾是错误的
* @param endIndex 停止从其开始的索引(不包括)。 将结束索引传递到数组的末尾是错误的
* @return 接合的字符串, null如果空数组输入
*/
public static String join(float[] array, char separator, int startIndex, int endIndex) {
if (array == null) {
return null;
}
int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
StringBuilder buf = new StringBuilder(noOfItems);
buf.append(array[startIndex]);
for (int i = startIndex + 1; i < endIndex; i++) {
buf.append(separator);
buf.append(array[i]);
}
return buf.toString();
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @return 接合的字符串, null如果空数组输入
*/
public static String join(int[] array, char separator) {
if (array == null) {
return null;
}
return join(array, separator, 0, array.length);
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @param startIndex 第一个开始加入的索引。 将起始索引传递到数组的末尾是错误的
* @param endIndex 停止从其开始的索引(不包括)。 将结束索引传递到数组的末尾是错误的
* @return 接合的字符串, null如果空数组输入
*/
public static String join(int[] array, char separator, int startIndex, int endIndex) {
if (array == null) {
return null;
}
int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
StringBuilder buf = new StringBuilder(noOfItems);
buf.append(array[startIndex]);
for (int i = startIndex + 1; i < endIndex; i++) {
buf.append(separator);
buf.append(array[i]);
}
return buf.toString();
}
/**
* 将提供的Iterable的元素连接到包含提供的元素的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或迭代中的空字符串由空字符串表示。
* 请参见此处的示例: join(Object[], char) 。
*
* @param iterable 提供值连接在一起的Iterable ,可以为null
* @param separator 要使用的分隔符
* @return 在加入字符串, null如果空迭代器输入
*/
public static String join(Iterable> iterable, char separator) {
if (iterable == null) {
return null;
}
return join(iterable.iterator(), separator);
}
/**
* 将提供的Iterable的元素连接到包含提供的元素的单个String中。
* 在列表之前或之后没有添加定界符。 null分隔符与空字符串(“”)相同。
* 请参见此处的示例: join(Object[], String) 。
*
* @param iterable 提供值连接在一起的Iterable ,可以为null
* @param separator 要使用的分隔符,将null视为“”
* @return 在加入字符串, null如果空迭代器输入
*/
public static String join(Iterable> iterable, String separator) {
if (iterable == null) {
return null;
}
return join(iterable.iterator(), separator);
}
/**
* 将提供的Iterator的元素连接到包含提供的元素的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或迭代中的空字符串由空字符串表示。
* 请参见此处的示例: join(Object[], char) 。
*
* @param iterator 要连接在一起的值的Iterator ,可以为null
* @param separator 要使用的分隔符
* @return 在加入字符串, null如果空迭代器输入
*/
public static String join(Iterator> iterator, char separator) {
// handle null, zero and one elements before building a buffer
if (iterator == null) {
return null;
}
if (!iterator.hasNext()) {
return EMPTY;
}
Object first = iterator.next();
if (!iterator.hasNext()) {
return Objects.toString(first, EMPTY);
}
// two or more elements
StringBuilder buf = new StringBuilder(STRING_BUILDER_SIZE); // Java default is 16, probably too small
if (first != null) {
buf.append(first);
}
while (iterator.hasNext()) {
buf.append(separator);
Object obj = iterator.next();
if (obj != null) {
buf.append(obj);
}
}
return buf.toString();
}
/**
* 将提供的Iterator的元素连接到包含提供的元素的单个String中。
* 在列表之前或之后没有添加定界符。 null分隔符与空字符串(“”)相同。
* 请参见此处的示例: join(Object[], String) 。
*
* @param iterator 要连接在一起的值的Iterator ,可以为null
* @param separator 要使用的分隔符,将null视为“”
* @return 在加入字符串, null如果空迭代器输入
*/
public static String join(Iterator> iterator, String separator) {
// handle null, zero and one elements before building a buffer
if (iterator == null) {
return null;
}
if (!iterator.hasNext()) {
return EMPTY;
}
Object first = iterator.next();
if (!iterator.hasNext()) {
return Objects.toString(first, "");
}
// two or more elements
StringBuilder buf = new StringBuilder(STRING_BUILDER_SIZE); // Java default is 16, probably too small
if (first != null) {
buf.append(first);
}
while (iterator.hasNext()) {
if (separator != null) {
buf.append(separator);
}
Object obj = iterator.next();
if (obj != null) {
buf.append(obj);
}
}
return buf.toString();
}
/**
* 将提供的List的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param list 要连接在一起的值List ,可以为null
* @param separator 要使用的分隔符
* @param startIndex 第一个开始加入的索引。 将起始索引传递到列表末尾是错误的
* @param endIndex 停止从其开始的索引(不包括)。 将结束索引传递到列表末尾是错误的
* @return 在加入字符串, null如果空列表输入
*/
public static String join(List> list, char separator, int startIndex, int endIndex) {
if (list == null) {
return null;
}
int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
List> subList = list.subList(startIndex, endIndex);
return join(subList.iterator(), separator);
}
/**
* 将提供的List的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param list 要连接在一起的值List ,可以为null
* @param separator 要使用的分隔符
* @param startIndex 第一个开始加入的索引。 将起始索引传递到列表末尾是错误的
* @param endIndex 停止从其开始的索引(不包括)。 将结束索引传递到列表末尾是错误的
* @return 在加入字符串, null如果空列表输入
*/
public static String join(List> list, String separator, int startIndex, int endIndex) {
if (list == null) {
return null;
}
int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
List> subList = list.subList(startIndex, endIndex);
return join(subList.iterator(), separator);
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @return 接合的字符串, null如果空数组输入
*/
public static String join(long[] array, char separator) {
if (array == null) {
return null;
}
return join(array, separator, 0, array.length);
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @param startIndex 第一个开始加入的索引。 将起始索引传递到列表末尾是错误的
* @param endIndex 停止从其开始的索引(不包括)。 将结束索引传递到列表末尾是错误的
* @return 接合的字符串, null如果空数组输入
*/
public static String join(long[] array, char separator, int startIndex, int endIndex) {
if (array == null) {
return null;
}
int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
StringBuilder buf = new StringBuilder(noOfItems);
buf.append(array[startIndex]);
for (int i = startIndex + 1; i < endIndex; i++) {
buf.append(separator);
buf.append(array[i]);
}
return buf.toString();
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @return 接合的字符串, null如果空数组输入
*/
public static String join(Object[] array, char separator) {
if (array == null) {
return null;
}
return join(array, separator, 0, array.length);
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @param startIndex 第一个开始加入的索引。 将起始索引传递到数组的末尾是错误的
* @param endIndex 停止从其开始的索引(不包括)。 将结束索引传递到数组的末尾是错误的
* @return 接合的字符串, null如果空数组输入
*/
public static String join(Object[] array, char separator, int startIndex, int endIndex) {
if (array == null) {
return null;
}
int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
StringBuilder buf = new StringBuilder(noOfItems);
if (array[startIndex] != null) {
buf.append(array[startIndex]);
}
for (int i = startIndex + 1; i < endIndex; i++) {
buf.append(separator);
if (array[i] != null) {
buf.append(array[i]);
}
}
return buf.toString();
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 null分隔符与空字符串(“”)相同。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符,将null视为“”
* @return 接合的字符串, null如果空数组输入
*/
public static String join(Object[] array, String separator) {
if (array == null) {
return null;
}
return join(array, separator, 0, array.length);
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 null分隔符与空字符串(“”)相同。 空对象或数组中的空字符串由空字符串表示。
*
* @param array the array of values to join together, may be null
* @param separator the separator character to use, null treated as ""
* @param startIndex the first index to start joining from.
* @param endIndex the index to stop joining from (exclusive).
* @return the joined String, {@code null} if null array input; or the empty string
*/
public static String join(Object[] array, String separator, int startIndex, int endIndex) {
if (array == null) {
return null;
}
if (separator == null) {
separator = EMPTY;
}
// endIndex - startIndex > 0: Len = NofStrings *(length(firstString) + length(separator))
// (Assuming that all Strings are roughly equally long)
int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
StringBuilder buf = new StringBuilder(noOfItems);
if (array[startIndex] != null) {
buf.append(array[startIndex]);
}
for (int i = startIndex + 1; i < endIndex; i++) {
buf.append(separator);
if (array[i] != null) {
buf.append(array[i]);
}
}
return buf.toString();
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @return 接合的字符串, null如果空数组输入
*/
public static String join(short[] array, char separator) {
if (array == null) {
return null;
}
return join(array, separator, 0, array.length);
}
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 在列表之前或之后没有添加定界符。 空对象或数组中的空字符串由空字符串表示。
*
* @param array 要连接在一起的值的数组,可以为null
* @param separator 要使用的分隔符
* @param startIndex 第一个开始加入的索引。 将起始索引传递到数组的末尾是错误的
* @param endIndex 停止从其开始的索引(不包括)。 将结束索引传递到数组的末尾是错误的
* @return 接合的字符串, null如果空数组输入
*/
public static String join(short[] array, char separator, int startIndex, int endIndex) {
if (array == null) {
return null;
}
int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
StringBuilder buf = new StringBuilder(noOfItems);
buf.append(array[startIndex]);
for (int i = startIndex + 1; i < endIndex; i++) {
buf.append(separator);
buf.append(array[i]);
}
return buf.toString();
}
// Joining
//-----------------------------------------------------------------------
/**
* 将提供的数组的元素连接到包含提供的元素列表的单个String中。
* 没有分隔符添加到连接的字符串。 空对象或数组中的空字符串由空字符串表示
*
* @param 连接在一起的特定值类型
* @param elements 要连接在一起的值,可以为null
* @return 接合的字符串, null如果空数组输入
*/
@SafeVarargs
public static String join(T... elements) {
return join(elements, null);
}
/**
* 将提供的varargs的元素连接到包含提供的元素的单个String中。
* 在列表之前或之后没有添加定界符。 null元素和分隔符被视为空字符串(“”)。
* @param separator 要使用的分隔符,将null视为“”
* @param objects 提供连接值的变量。 null元素被视为“”
* @return 联接的字符串
* @throws java.lang.IllegalArgumentException 抛出异常
*/
public static String joinWith(String separator, Object... objects) {
if (objects == null) {
throw new IllegalArgumentException("Object varargs must not be null");
}
String sanitizedSeparator = defaultString(separator);
StringBuilder result = new StringBuilder();
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy