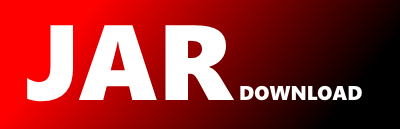
com.github.cassandra.jdbc.CassandraUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cassandra-jdbc-driver Show documentation
Show all versions of cassandra-jdbc-driver Show documentation
Type 4 JDBC driver for Apache Cassandra built on top of existing great libs like java driver from
DataStax. It supports Cassandra 2.x and above with improved SQL compatibility.
/*
*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*
*/
package com.github.cassandra.jdbc;
import org.pmw.tinylog.Logger;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.text.MessageFormat;
import java.util.*;
/**
* This is a utility class.
*
* @author Zhichun Wu
*/
public class CassandraUtils {
static final String BUNDLE_NAME = CassandraUtils.class.getPackage()
.getName() + ".messages";
static final ResultSet DUMMY_RESULT_SET = new DummyCassandraResultSet();
static final ResourceBundle RESOURCE_BUNDLE;
public static final String KEY_DB_MAJOR_VERSION = "dbMajorVersion";
public static final String KEY_DB_MINOR_VERSION = "dbMinorVersion";
public static final String KEY_DRIVER_NAME = "driverName";
public static final String KEY_DRIVER_VERSION = "driverVersion";
public static final String EMPTY_STRING = "";
public static final String[][] SCHEMA_COLUMNS = new String[][]{{
"TABLE_CAT", "text"}};
public static final String[][] COLUMN_COLUMNS = new String[][]{
{"TABLE_CAT", "text"}, {"TABLE_SCHEM", "text"},
{"TABLE_NAME", "text"}, {"COLUMN_NAME", "text"},
{"DATA_TYPE", "int"}, {"TYPE_NAME", "text"},
{"COLUMN_SIZE", "int"}, {"BUFFER_LENGTH", "int"},
{"DECIMAL_DIGITS", "int"}, {"NUM_PREC_RADIX", "int"},
{"NULLABLE", "int"}, {"REMARKS", "text"},
{"COLUMN_DEF", "text"}, {"SQL_DATA_TYPE", "int"},
{"SQL_DATETIME_SUB", "int"}, {"CHAR_OCTET_LENGTH", "int"},
{"ORDINAL_POSITION", "int"}, {"IS_NULLABLE", "text"},
{"SCOPE_CATALOG", "text"}, {"SCOPE_SCHEMA", "text"},
{"SCOPE_TABLE", "text"}, {"SOURCE_DATA_TYPE", "short"},
{"IS_AUTOINCREMENT", "text"}, {"IS_GENERATEDCOLUMN", "text"}};
public static final String CURSOR_PREFIX = "cursor@";
public static final String DEFAULT_DB_MAJOR_VERSION = "2";
public static final String DEFAULT_DB_MINOR_VERSION = "0";
public static final String[][] INDEX_COLUMNS = new String[][]{
{"TABLE_CAT", "text"}, {"TABLE_SCHEM", "text"},
{"TABLE_NAME", "text"}, {"NON_UNIQUE", "boolean"},
{"INDEX_QUALIFIER", "text"}, {"INDEX_NAME", "text"},
{"TYPE", "int"}, {"ORDINAL_POSITION", "int"},
{"COLUMN_NAME", "text"}, {"ASC_OR_DESC", "text"},
{"CARDINALITY", "int"}, {"PAGES", "int"},
{"FILTER_CONDITION", "text"}};
public static final String KEY_APPROXIMATE_INDEX = "approximateIndexInfo";
public static final String KEY_CATALOG = "catalog";
public static final String KEY_COLUMN_PATTERN = "columnNamePattern";
public static final String KEY_NUMERIC_FUNCTIONS = "numericFunctions";
public static final String KEY_PRODUCT_NAME = "productName";
public static final String KEY_PRODUCT_VERSION = "productVersion";
public static final String KEY_SQL_KEYWORDS = "keywords";
public static final String KEY_STRING_FUNCTIONS = "stringFunctions";
public static final String KEY_SYSTEM_FUNCTIONS = "systemFunctions";
public static final String KEY_SCHEMA_PATTERN = "schemaPattern";
public static final String KEY_TABLE_PATTERN = "tableNamePattern";
public static final String KEY_TIMEDATE_FUNCTIONS = "timeDateFunctions";
public static final String KEY_TYPE_PATTERN = "typeNamePattern";
public static final String KEY_UNIQUE_INDEX = "uniqueIndexOnly";
public static final String[][] PK_COLUMNS = new String[][]{
{"TABLE_CAT", "text"}, {"TABLE_SCHEM", "text"},
{"TABLE_NAME", "text"}, {"COLUMN_NAME", "text"},
{"KEY_SEQ", "int"}, {"PK_NAME", "text"}};
public static final String[][] TABLE_COLUMNS = new String[][]{
{"TABLE_CAT", "text"}, {"TABLE_SCHEM", "text"},
{"TABLE_NAME", "text"}, {"TABLE_TYPE", "text"},
{"REMARKS", "text"}, {"TYPE_CAT", "text"},
{"TYPE_SCHEM", "text"}, {"TYPE_NAME", "text"},
{"SELF_REFERENCING_COL_NAME", "text"},
{"REF_GENERATION", "text"}};
// meta data
public static final String[][] TABLE_TYPE_COLUMNS = new String[][]{{
"TABLE_TYPE", "text"}};
public static final Object[][] TABLE_TYPE_DATA = new Object[][]{new Object[]{"TABLE"}};
public static final String[][] TYPE_COLUMNS = new String[][]{
{"TYPE_NAME", "text"}, {"DATA_TYPE", "int"},
{"PRECISION", "int"}, {"LITERAL_PREFIX", "text"},
{"LITERAL_SUFFIX", "text"}, {"CREATE_PARAMS", "text"},
{"NULLABLE", "int"}, {"CASE_SENSITIVE", "boolean"},
{"SEARCHABLE", "int"}, {"UNSIGNED_ATTRIBUTE", "boolean"},
{"FIXED_PREC_SCALE", "boolean"}, {"AUTO_INCREMENT", "boolean"},
{"LOCAL_TYPE_NAME", "text"}, {"MINIMUM_SCALE", "int"},
{"MAXIMUM_SCALE", "int"}, {"SQL_DATA_TYPE", "int"},
{"SQL_DATETIME_SUB", "int"}, {"NUM_PREC_RADIX", "int"}};
public static final String[][] UDT_COLUMNS = new String[][]{
{"TYPE_CAT", "text"}, {"TYPE_SCHEM", "text"},
{"TYPE_NAME", "text"}, {"CLASS_NAME", "text"},
{"DATA_TYPE", "int"}, {"REMARKS", "text"},
{"BASE_TYPE", "int"}};
static {
ResourceBundle bundle = null;
try {
bundle = ResourceBundle.getBundle(BUNDLE_NAME, Locale.getDefault(),
CassandraUtils.class.getClassLoader());
} catch (Throwable t) {
try {
bundle = ResourceBundle.getBundle(BUNDLE_NAME, Locale.US);
} catch (Throwable e) {
throw new RuntimeException(
"Failed to load resource bundle due to underlying exception: "
+ t.toString(), e);
}
} finally {
RESOURCE_BUNDLE = bundle;
}
}
public static Object[][] getAllData(ResultSet rs) throws SQLException {
return getAllData(rs, true);
}
public static Object[][] getAllData(ResultSet rs, boolean closeResultSet)
throws SQLException {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy