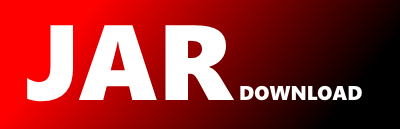
com.zcj.util.UtilImageBase Maven / Gradle / Ivy
package com.zcj.util;
import com.zcj.util.filenameutils.FilenameUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import sun.misc.BASE64Decoder;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
public class UtilImageBase {
private static final Logger logger = LoggerFactory.getLogger(UtilImageBase.class);
/* 根据图片物理路径获取图片的宽和高 */
public static Integer[] getWidthAndHeight(String filePath) {
if (UtilString.isNotBlank(filePath)) {
File file = new File(filePath);
if (file.exists()) {
try {
BufferedImage bi = ImageIO.read(file);
return new Integer[]{bi.getWidth(), bi.getHeight()};
} catch (Exception e) {
logger.error(e.getMessage(), e);
}
}
}
return new Integer[]{null, null};
}
/* 根据图片输入流获取图片的宽和高 */
public static Integer[] getWidthAndHeight(InputStream inputStream) {
if (inputStream != null) {
try {
BufferedImage bi = ImageIO.read(inputStream);
return new Integer[]{bi.getWidth(), bi.getHeight()};
} catch (Exception e) {
logger.error(e.getMessage(), e);
}
}
return new Integer[]{null, null};
}
/**
* BASE64 编码内容转成图片存储到指定目录
*
* @param base64 Base64 编码字符串
* @param savePath 保存图片的目录
* @return boolean
*/
public static boolean base64ToImage(final String base64, final String savePath) {
String absolutePath = FilenameUtils.getFullPath(savePath);
File dir = new File(absolutePath);
if (!dir.exists()) {
dir.mkdirs();
}
try (FileOutputStream write = new FileOutputStream(new File(savePath))) {
BASE64Decoder decoder = new BASE64Decoder();
byte[] decoderBytes = decoder.decodeBuffer(base64);
write.write(decoderBytes);
return true;
} catch (IOException e) {
logger.error(e.getMessage(), e);
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy