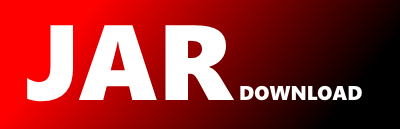
com.zcj.util.coder.page.PageColumnBean Maven / Gradle / Ivy
package com.zcj.util.coder.page;
import com.zcj.util.UtilConvert;
import com.zcj.util.UtilString;
import com.zcj.util.coder.CodeGenerateException;
import com.zcj.util.coder.CoderUtil;
import com.zcj.util.coder.consant.FieldTypeEnum;
import com.zcj.util.coder.page.constant.InputTypeEnum;
import com.zcj.util.coder.visual.FieldInfoDto;
import org.springframework.core.annotation.AnnotatedElementUtils;
import java.lang.reflect.Field;
import java.util.*;
public class PageColumnBean {
// bigDecimalScale 支持的最大值
private static final Integer BIG_DECIMAL_SCALE_MAX = 4;
// bigDecimalScale 支持的最小值
private static final Integer BIG_DECIMAL_SCALE_MIN = 2;
// 下拉key的默认属性
private static final String SELECT_OPTION_KEY_DEFAULT = "id";
// 下拉title的默认属性
private static final String SELECT_OPTION_VALUE_DEFAULT = "name";
// 中文名
private String name = "";
// 属性名
private String fieldName = "";
// 是否可编辑(默认是)
private boolean modify = true;
// 编辑页面中的辅助文字
private String modifyAuxWord = null;
// 编辑页中此字段单独一行显示(默认否)
private boolean modifyLineAlone = false;
// 是否必填(默认否)
private boolean must = false;
// 类型,可取值:text[默认]、textarea、select、img、date、datetime、ueditor
private String type = InputTypeEnum.text.name();
// 验证方式(当 type="text||textarea||select||date" 时可用)(默认空[不验证])
// 必填验证[data-check="must"]不需要设置,通过是否必填属性[PageColumnBean.must]决定是否必填,因为关系到页面的星号[*]显示
private String check = null;
// 输入的最大长度(当 type="text" 时可用)(默认空[不限制])
private Integer maxlength = null;
// 默认值(当 type="text||textarea||img||date||ueditor" 时可用)(默认空[无默认值])
private String defaultValue = null;
// 数据字典(当 type="select" 时可用):【key="1",value="系统管理员"】
private Map keyValue = new HashMap<>();
// 数据字典(当 type="select" 时可用):['depts', 'id', 'name']
private String[] selectOption = new String[3];
// 是否表格中显示(默认否)
private boolean grid = false;
// 是否提供查询(默认否)
private boolean search = false;
// 是否为 BigDecimal 属性
private boolean bigDecimal = false;
// BigDecimal 属性保留的小数位
private int bigDecimalScale = 2;
private int orderSearch = 999;
private int orderGrid = 999;
private int orderModify = 999;
// FieldInfoDto 转 PageColumnBean
public static PageColumnBean byFieldInfoDto(final FieldInfoDto fieldInfoDto) {
if (fieldInfoDto == null) {
throw new CodeGenerateException("fieldInfoDto 不能为空");
}
PageColumnBean result = new PageColumnBean();
result.setFieldName(fieldInfoDto.getFieldName());
result.setName(UtilString.firstUnEmpty(fieldInfoDto.getCnName(), fieldInfoDto.getFieldName()));
if (fieldInfoDto.getModifyLineAlone() != null) {
result.setModifyLineAlone(fieldInfoDto.getModifyLineAlone());
}
if (fieldInfoDto.getModifyMust() != null) {
result.setMust(fieldInfoDto.getModifyMust());
}
if (UtilString.isNotBlank(fieldInfoDto.getInputType())) {
result.setType(fieldInfoDto.getInputType());
}
if (UtilString.isNotBlank(fieldInfoDto.getModifyAuxWord())) {
result.setModifyAuxWord(fieldInfoDto.getModifyAuxWord());
}
if (fieldInfoDto.getModifyMaxLength() != null) {
result.setMaxlength(fieldInfoDto.getModifyMaxLength());
}
String modifyCheck = UtilConvert.collection2String(fieldInfoDto.getModifyCheck());
if (UtilString.isNotBlank(modifyCheck)) {
result.setCheck(modifyCheck);
}
if (UtilString.isNotBlank(fieldInfoDto.getModifyDefaultValue())) {
result.setDefaultValue(fieldInfoDto.getModifyDefaultValue());
}
if (InputTypeEnum.select.name().equals(fieldInfoDto.getInputType())) {
if (UtilString.isNotBlank(fieldInfoDto.getSelectKeyValues())) {
Map kvMap = new LinkedHashMap<>();
String[] kvArray = fieldInfoDto.getSelectKeyValues().split("&");
if (kvArray != null && kvArray.length > 0) {
for (String kvString : kvArray) {
String[] kv2Array = kvString.split("=");
kvMap.put(addQuot(kv2Array[0]), addQuot(kv2Array[1]));
}
}
result.setKeyValue(kvMap);
} else {
result.setSelectOption(new String[]{
UtilString.empty2String(fieldInfoDto.getSelectOptionList(), fieldInfoDto.getFieldName() + "s"),
UtilString.empty2String(fieldInfoDto.getSelectOptionKey(), SELECT_OPTION_KEY_DEFAULT),
UtilString.empty2String(fieldInfoDto.getSelectOptionValue(), SELECT_OPTION_VALUE_DEFAULT)});
}
}
if (FieldTypeEnum.BigDecimal.name().equals(fieldInfoDto.getFieldType())) {
result.setBigDecimal(true);
Integer scale = fieldInfoDto.getModifyBigDecimalScale();
if (scale != null && scale >= BIG_DECIMAL_SCALE_MIN && scale <= BIG_DECIMAL_SCALE_MAX) {
result.setBigDecimalScale(scale);
}
}
if (fieldInfoDto.getPageOrderGrid() != null && fieldInfoDto.getPageOrderGrid() > 0) {
result.setOrderGrid(fieldInfoDto.getPageOrderGrid());
result.setGrid(true);
}
if (fieldInfoDto.getPageOrderModify() != null && fieldInfoDto.getPageOrderModify() > 0) {
result.setOrderModify(fieldInfoDto.getPageOrderModify());
result.setModify(true);
} else {
result.setModify(false);
}
if (fieldInfoDto.getPageOrderSearch() != null && fieldInfoDto.getPageOrderSearch() > 0) {
result.setOrderSearch(fieldInfoDto.getPageOrderSearch());
result.setSearch(true);
}
return result;
}
// 注解(@PageColumnType、@CnName、@ApiModelProperty) 转 PageColumnBean 集合
public static List byClassAnnotation(final Class> c) {
if (c == null) {
throw new CodeGenerateException("class 不能为空");
}
List columnList = new ArrayList<>();
List fs = CoderUtil.allField(c, false);
for (Field f : fs) {
PageColumnType ct = AnnotatedElementUtils.findMergedAnnotation(f, PageColumnType.class);
PageColumnBean b = new PageColumnBean();
b.setFieldName(f.getName());
if ("class java.math.BigDecimal".equals(f.getType().toString())) {
b.setBigDecimal(true);
if (ct != null && ct.bigDecimalScale() >= BIG_DECIMAL_SCALE_MIN && ct.bigDecimalScale() <= BIG_DECIMAL_SCALE_MAX) {
b.setBigDecimalScale(ct.bigDecimalScale());
}
}
if (ct != null) {
if (UtilString.isNotBlank(ct.check())) {
b.setCheck(ct.check());
}
if (UtilString.isNotBlank(ct.defaultValue())) {
b.setDefaultValue(ct.defaultValue());
}
b.setType(ct.type());
Map kvMap = new LinkedHashMap<>();
String[] kvArray = ct.keyValue();
if (kvArray != null && kvArray.length > 0) {
for (String kvString : kvArray) {
String[] kv2Array = kvString.split("=");
kvMap.put(addQuot(kv2Array[0]), addQuot(kv2Array[1]));
}
b.setType(InputTypeEnum.select.name());
}
b.setKeyValue(kvMap);
if (ct.maxlength() != 0) {
b.setMaxlength(ct.maxlength());
}
b.setModify(ct.modify());
if (UtilString.isNotBlank(ct.modifyAuxWord())) {
b.setModifyAuxWord(ct.modifyAuxWord());
}
b.setModifyLineAlone(ct.modifyLineAlone());
b.setMust(ct.must());
b.setName(ct.name());
String selectOptionList = ct.selectOptionList();
if (UtilString.isBlank(selectOptionList)) {
selectOptionList = f.getName() + "s";
}
b.setSelectOption(new String[]{selectOptionList, ct.selectOptionKey(), ct.selectOptionValue()});
b.setOrderGrid(ct.orderGrid());
b.setOrderModify(ct.orderModify());
b.setOrderSearch(ct.orderSearch());
if (ct.orderGrid() < 999) {
b.setGrid(true);
}
if (ct.orderSearch() < 999) {
b.setSearch(true);
}
if (ct.orderModify() < 999) {
b.setModify(true);
}
}
if (UtilString.isBlank(b.getName())) {
String cnName = CoderUtil.getCnName(f);
String apiModelValue = CoderUtil.getApiModelPropertyValue(f);
String tableColumnComment = CoderUtil.getTableColumnComment(f);
String filedName = f.getName();
b.setName(UtilString.firstUnEmpty(cnName, apiModelValue, tableColumnComment, filedName));
}
columnList.add(b);
}
return columnList;
}
private static final String REGEX = "[0-9.]+";
/**
* 处理字符串左右的单引号
*
*
*
* 'a' --> 'a'
* a --> 'a'
* 'a --> ''a'
* 1 --> 1
* 1.2 --> 1.2
* '1' --> '1'
* '12 --> ''12'
*
*
* @param val 待处理的字符串
* @return 处理后的字符串
*/
private static String addQuot(String val) {
if (UtilString.isNotBlank(val)) {
if (!val.matches(REGEX)) {
if (!val.startsWith("'") || !val.endsWith("'")) {
return "'" + val + "'";
}
}
}
return val;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getOrderSearch() {
return orderSearch;
}
public void setOrderSearch(int orderSearch) {
this.orderSearch = orderSearch;
}
public int getOrderGrid() {
return orderGrid;
}
public void setOrderGrid(int orderGrid) {
this.orderGrid = orderGrid;
}
public boolean isModifyLineAlone() {
return modifyLineAlone;
}
public void setModifyLineAlone(boolean modifyLineAlone) {
this.modifyLineAlone = modifyLineAlone;
}
public int getOrderModify() {
return orderModify;
}
public void setOrderModify(int orderModify) {
this.orderModify = orderModify;
}
public String getFieldName() {
return fieldName;
}
public void setFieldName(String fieldName) {
this.fieldName = fieldName;
}
public boolean isModify() {
return modify;
}
public void setModify(boolean modify) {
this.modify = modify;
}
public boolean isMust() {
return must;
}
public boolean isBigDecimal() {
return bigDecimal;
}
public void setBigDecimal(boolean bigDecimal) {
this.bigDecimal = bigDecimal;
}
public int getBigDecimalScale() {
return bigDecimalScale;
}
public void setBigDecimalScale(int bigDecimalScale) {
this.bigDecimalScale = bigDecimalScale;
}
public void setMust(boolean must) {
this.must = must;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getCheck() {
return check;
}
public String getModifyAuxWord() {
return modifyAuxWord;
}
public void setModifyAuxWord(String modifyAuxWord) {
this.modifyAuxWord = modifyAuxWord;
}
public String[] getSelectOption() {
return selectOption;
}
public void setSelectOption(String[] selectOption) {
this.selectOption = selectOption;
}
public void setCheck(String check) {
this.check = check;
}
public Integer getMaxlength() {
return maxlength;
}
public void setMaxlength(Integer maxlength) {
this.maxlength = maxlength;
}
public String getDefaultValue() {
return defaultValue;
}
public void setDefaultValue(String defaultValue) {
this.defaultValue = defaultValue;
}
public Map getKeyValue() {
return keyValue;
}
public void setKeyValue(Map keyValue) {
this.keyValue = keyValue;
}
public boolean isGrid() {
return grid;
}
public void setGrid(boolean grid) {
this.grid = grid;
}
public boolean isSearch() {
return search;
}
public void setSearch(boolean search) {
this.search = search;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy