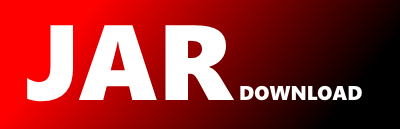
com.zcj.web.mybatisplus.action.BaseAction Maven / Gradle / Ivy
package com.zcj.web.mybatisplus.action;
import com.baomidou.mybatisplus.extension.service.IService;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.zcj.util.UtilDate;
import com.zcj.util.UtilString;
import com.zcj.util.json.gson.GsonFieldNamingStrategy;
import com.zcj.util.validator.ValidatorUtil;
import com.zcj.web.exception.BusinessAssert;
import com.zcj.web.exception.BusinessException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.WebDataBinder;
import org.springframework.web.bind.annotation.InitBinder;
import java.beans.PropertyEditorSupport;
import java.math.BigDecimal;
import java.util.Date;
public class BaseAction {
@Autowired
protected S baseService;
@InitBinder
public void initBinder(WebDataBinder binder) {
binder.setAutoGrowCollectionLimit(10000);
binder.registerCustomEditor(Date.class, new DateEditor());
binder.registerCustomEditor(BigDecimal.class, new BigDecimalEditor());
}
private class DateEditor extends PropertyEditorSupport {
@Override
public void setAsText(String text) {
Date date = UtilDate.format(text);
setValue(date);
}
}
private class BigDecimalEditor extends PropertyEditorSupport {
@Override
public void setAsText(String text) {
if (UtilString.isNotBlank(text)) {
text = text.replace(",", "");
setValue(text);
}
}
}
/**
* 把 searchDateVal 的值通过分隔符(默认“ - ”)拆分后转成 Date 格式
*
* @param searchDateVal 待处理的字符串
* @param splitVal 分隔符
* @return java.util.Date[]
*/
protected Date[] splitSearchDate(String searchDateVal, String splitVal) {
Date[] result = new Date[]{null, null};
if (UtilString.isBlank(splitVal)) {
splitVal = " - ";
}
if (UtilString.isNotBlank(searchDateVal)) {
String[] a = searchDateVal.split(splitVal);
if (a.length == 2) {
result[0] = UtilDate.format(a[0]);
result[1] = UtilDate.format(a[1]);
}
}
return result;
}
/**
* GSON实例(会把待转对象中的pid属性名称改成parentId,name属性名改成title)
*
* @return Gson
*/
protected Gson dtreeGson() {
return new GsonBuilder()
.setFieldNamingStrategy(new GsonFieldNamingStrategy(new String[]{"pid", "name"}, new String[]{"parentId", "title"}))
.create();
}
// 参数验证
protected void validParam(Object param, boolean paramNullable) {
if (param == null && paramNullable) {
return;
} else if (param == null) {
throw new BusinessException("参数不能为空");
}
String validResult = ValidatorUtil.validSingle(param);
BusinessAssert.checkArgument(validResult == null, validResult);
}
// 参数验证
protected void validParam(Object param) {
this.validParam(param, false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy