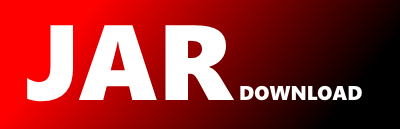
com.zcj.web.mybatisplus.entity.BaseTreeEntity Maven / Gradle / Ivy
package com.zcj.web.mybatisplus.entity;
import com.baomidou.mybatisplus.annotation.TableField;
import com.zcj.util.coder.database.TableColumnType;
import com.zcj.util.coder.page.PageColumnType;
import io.swagger.annotations.ApiModelProperty;
import java.util.*;
public class BaseTreeEntity extends BaseEntity {
private static final long serialVersionUID = -3218419989046314842L;
@ApiModelProperty("上级ID")
@PageColumnType(type = "select")
private Long pid;
@ApiModelProperty("名称")
@TableColumnType(nullable = false)
@PageColumnType(must = true, orderGrid = 1)
private String name;
// -------------------------------------------- 以下非数据库表字段 ------------------------------------------------
@TableField(exist = false)
@TableColumnType(exclude = true)
private List children;
@TableField(exist = false)
@TableColumnType(exclude = true)
private Long counts;// 数量
@TableField(exist = false)
@TableColumnType(exclude = true)
private String pname;// 上级名称
// 移除集合中重复的记录
public static List removeSame(List list) {
List result = new ArrayList<>();
if (list != null && list.size() > 0) {
Map map = new LinkedHashMap<>();
for (T c : list) {
map.put(c.getId(), c);
}
for (Map.Entry entry : map.entrySet()) {
result.add(entry.getValue());
}
}
return result;
}
// 先转Tree结构,再转List结构
public static List convertToTreeList(List list) {
return convertToTreeList(list, null, null);
}
// 先转Tree结构,再转List结构
public static List convertToTreeList(List list, String prefixBegin, String prefix) {
if (list != null && list.size() > 0) {
list = convertTree(list);
list = convertTreeToList(list, prefixBegin, prefix);
}
return list;
}
// 集合数据转换为树形数据(根节点的 pid 必须为 null)
public static List convertTree(List list) {
List result = new ArrayList();
if (list != null && list.size() > 0) {
for (T c : list) {
c.setChildren(null);
}
for (T c : list) {
if (c.getPid() == null) {
addChilds(c, list);
result.add(c);
}
}
}
return result;
}
/**
* 集合数据转换为树形数据
*
* @param list 集合数据
* @param topPidAllowNotNull 根节点的pid是否允许非空(true:只要节点的pid不存在,则就当做根节点;false:pid为空才当做根节点)
* @param 类型
* @return java.util.List
*/
public static List convertTree(List list, boolean topPidAllowNotNull) {
List result = new ArrayList();
if (list != null && list.size() > 0) {
Set ids = new HashSet<>();
if (topPidAllowNotNull) {
for (T c : list) {
ids.add(c.getId());
}
}
for (T c : list) {
if (c.getPid() == null || (topPidAllowNotNull && !ids.contains(c.getPid()))) {
addChilds(c, list);
result.add(c);
}
}
}
return result;
}
private static void addChilds(T link, List list) {
if (link != null && list != null) {
Long cou = link.getCounts() == null ? 0L : link.getCounts();
for (T c : list) {
if (c.getPid() != null && c.getPid().equals(link.getId())) {
List chi = link.getChildren();
if (chi == null) {
chi = new ArrayList();
}
addChilds(c, list);
chi.add(c);
if (c.getCounts() != null && c.getCounts() != 0) {
cou += c.getCounts();
}
link.setChildren(chi);
link.setCounts(cou);
}
}
}
}
// 树形数据转化为集合数据
public static List convertTreeToList(List list, String prefixBegin, String prefix) {
List result = new ArrayList();
if (list != null && list.size() > 0) {
addListChilds(list, result, 0, prefixBegin, prefix);
}
for (T a : result) {
a.setChildren(null);
}
return result;
}
// 树形数据转化为集合数据
public static List convertTreeToList(List list) {
return convertTreeToList(list, null, null);
}
private static List addListChilds(List list, List result, int count,
String prefixBegin, String prefix) {
if (list != null && list.size() > 0) {
for (T obj : list) {
obj.setName(showName(obj.getName(), count, prefixBegin, prefix));
result.add(obj);
result = addListChilds(obj.getChildren(), result, count + 1, prefixBegin, prefix);
}
}
return result;
}
private static String showName(String name, int count, String prefixBegin, String prefix) {
StringBuilder sb = new StringBuilder();
int index = 0;
if (prefixBegin != null) {
sb.append(prefixBegin);
}
while (index < count) {
if (prefix != null) {
sb.append(prefix);
}
index++;
}
sb.append(name);
return sb.toString();
}
public Long getPid() {
return pid;
}
public void setPid(Long pid) {
this.pid = pid;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List getChildren() {
return children;
}
public void setChildren(List children) {
this.children = children;
}
public Long getCounts() {
return counts;
}
public void setCounts(Long counts) {
this.counts = counts;
}
public String getPname() {
return pname;
}
public void setPname(String pname) {
this.pname = pname;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy