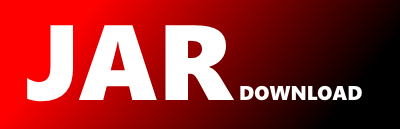
com.zcj.web.redislock.RedisLockAopAspect Maven / Gradle / Ivy
package com.zcj.web.redislock;
import com.zcj.util.UtilString;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.Signature;
import org.aspectj.lang.annotation.AfterThrowing;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.lang.reflect.Method;
import java.util.concurrent.TimeUnit;
@Aspect
@Component
public class RedisLockAopAspect {
private static ThreadLocal REQUEST_ID = new ThreadLocal<>();
@Autowired
private RedisLock redisLock;
@Pointcut(value = "@annotation(com.zcj.web.redislock.RedisLockAop)")
public void lockService() {
// Do nothing
}
@Around("lockService()")
public Object executeAop(ProceedingJoinPoint point) throws Throwable {
RedisLockAop lock = getRedisLockAop(point);
String requestId = UtilString.getUUID();
REQUEST_ID.set(requestId);
if (RedisLockTypeEnum.WAIT.equals(lock.type())) {
while (!redisLock.tryLock(lock.key(), requestId, lock.timeout(), TimeUnit.MINUTES)) {
Thread.sleep(100);
}
} else if (RedisLockTypeEnum.CANCEL.equals(lock.type())) {
if (!redisLock.tryLock(lock.key(), requestId, lock.timeout(), TimeUnit.MINUTES)) {
return null;
}
}
Object val = point.proceed();
REQUEST_ID.remove();
redisLock.releaseLock(lock.key(), requestId);
return val;
}
@AfterThrowing(throwing = "e", pointcut = "lockService()")
public void throwsExecute(JoinPoint joinPoint, Exception e) {
RedisLockAop lock = getRedisLockAop(joinPoint);
redisLock.releaseLock(lock.key(), REQUEST_ID.get());
}
// 获取注解信息
private RedisLockAop getRedisLockAop(JoinPoint joinPoint) {
Method currentMethod = getMethod(joinPoint);
return currentMethod.getAnnotation(RedisLockAop.class);
}
private Method getMethod(JoinPoint joinPoint) {
Signature sig = joinPoint.getSignature();
MethodSignature msig = null;
if (!(sig instanceof MethodSignature)) {
throw new IllegalArgumentException("该注解只能用于方法");
}
msig = (MethodSignature) sig;
Object target = joinPoint.getTarget();
Method currentMethod = null;
try {
currentMethod = target.getClass().getMethod(msig.getName(), msig.getParameterTypes());
} catch (NoSuchMethodException e) {
throw new RuntimeException("该注解只能用于方法");
}
return currentMethod;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy