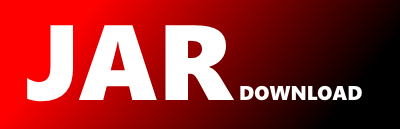
webit.script.global.DefaultGlobalManager Maven / Gradle / Ivy
// Copyright (c) 2013, Webit Team. All Rights Reserved.
package webit.script.global;
import java.util.HashMap;
import java.util.Map;
import webit.script.Engine;
import webit.script.Initable;
/**
*
* @author zqq90
*/
public class DefaultGlobalManager implements GlobalManager, Initable {
private final Map constMap;
private Map globalMap;
private Object[] globalContext;
private Map globalIndexerMap;
private boolean committed = false;
private Class[] registers;
public DefaultGlobalManager() {
this.constMap = new HashMap();
this.globalMap = new HashMap();
}
public void init(Engine engine) {
if (registers != null) {
for (int i = 0, len = registers.length; i < len; i++) {
try {
((GlobalRegister) engine.getBean(registers[i]))
.regist(this);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
}
}
public void commit() {
if (!this.committed) {
this.committed = true;
final int size;
this.globalContext = new Object[size = this.globalMap.size()];
this.globalIndexerMap = new HashMap((size * 4) / 3 + 1, 0.75f);
int i = 0;
for (Map.Entry entry : this.globalMap.entrySet()) {
this.globalContext[i] = entry.getValue();
this.globalIndexerMap.put(entry.getKey(), i);
i++;
}
this.globalMap = null;
}
}
public void setConst(String key, Object value) {
if (!this.committed) {
this.constMap.put(key, value);
}
}
public void setGlobal(String key, Object value) {
if (!this.committed) {
this.globalMap.put(key, value);
}
}
public int getVariantIndex(String name) {
Integer index;
return (index = globalIndexerMap.get(name)) != null ? index : -1;
}
public Object getVariant(int index) {
return globalContext[index];
}
public void setVariant(int index, Object value) {
this.globalContext[index] = value;
}
public boolean hasConst(String name) {
return this.constMap.containsKey(name);
}
public Object getConst(String name) {
return this.constMap.get(name);
}
public void setRegisters(Class[] registers) {
this.registers = registers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy