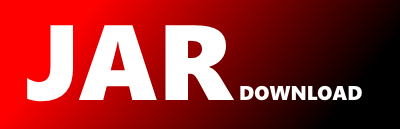
db.FlexUser Maven / Gradle / Ivy
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package db;
import java.util.HashSet;
import java.util.Set;
import org.neo4j.ogm.annotation.GraphId;
import org.neo4j.ogm.annotation.NodeEntity;
/**
*
* @author zua
*/
@NodeEntity
public class FlexUser extends GraphEntity {
private static final long serialVersionUID = -5822981670795508682L;
@GraphId
private String advertisementUrl;
private String username;
private String password;
private Set read;
private Set favorite;
private Set fake;
private AuthUserInfo userInfo;
public FlexUser() {
read = new HashSet<>();
favorite = new HashSet<>();
fake = new HashSet<>();
}
public FlexUser(String username, String password) {
this();
this.username = username;
this.password = password;
}
@Override
public String getPropertyName() {
return "username";
}
@Override
public String getPropertyValue() {
return username;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public void read(GraphEntity article) {
read.add(article);
}
public boolean hasRead(GraphEntity article) {
return read.contains(article);
}
public void favorite(GraphEntity article) {
favorite.add(article);
}
public boolean hasFavorite(GraphEntity article) {
return favorite.contains(article);
}
public void fake(GraphEntity article) {
fake.add(article);
}
public boolean hasFake(GraphEntity article) {
return fake.contains(article);
}
@Override
public boolean hasUrl() {
return false;
}
@Override
public String getUrl() {
return null;
}
public Set getRead() {
return read;
}
public Set getFavorite() {
return favorite;
}
public Set getFake() {
return fake;
}
public void setRead(Set read) {
this.read = read;
}
public void setFavorite(Set favorite) {
this.favorite = favorite;
}
public void setFake(Set fake) {
this.fake = fake;
}
public AuthUserInfo getUserInfo() {
return userInfo;
}
public void setUserInfo(AuthUserInfo userInfo) {
this.userInfo = userInfo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy