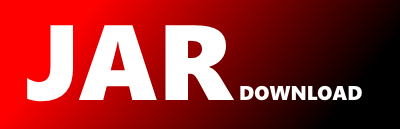
backend.services.news.NewsArticleService Maven / Gradle / Ivy
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package backend.services.news;
import db.news.NewsArticle;
import java.util.Collection;
import java.util.HashMap;
import javax.ejb.Stateless;
import org.neo4j.ogm.cypher.query.Pagination;
import org.neo4j.ogm.cypher.query.SortOrder;
import backend.queries.ArticlesQueries;
import backend.services.AbstractDBService;
/**
*
* @author zua
*/
@Stateless
public class NewsArticleService extends AbstractDBService {
public NewsArticle findArticleWithTitle(String title) {
return find(title);
}
public Iterable findArticlesWithUrl(String url) {
return getSession().query(NewsArticle.class, ArticlesQueries.findArticleWithUrl(url), new HashMap<>());
}
@Override
public Class getClassType() {
return NewsArticle.class;
}
@Override
public SortOrder getSortOrderAsc() {
return new SortOrder().add(SortOrder.Direction.ASC, "publishedAt");
}
@Override
public SortOrder getSortOrderDesc() {
return new SortOrder().add(SortOrder.Direction.DESC, "publishedAt");
}
public Iterable findArticlesWithoutShortUrl() {
String query = "MATCH (n:NewsArticle) ";
query += "WHERE n.shortUrl IS NULL RETURN n ";
query += "ORDER BY n.publishedAt DESC LIMIT " + LIMIT;
System.out.println(query);
return executeQuery(query);
}
public Collection findAll(int page, int pageSize) {
Pagination paging = new Pagination(page, pageSize);
return getSession().loadAll(NewsArticle.class, paging);
}
public Iterable findArticlesTaggedAs(String tag) {
String cypher = ArticlesQueries.findArticlesTaggedAs(tag);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findArticlesTaggedAs(String username, String tag) {
String cypher = ArticlesQueries.findArticlesTaggedAs(username, tag);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findArticlesPublishedBy(String sourceId) {
String cypher = ArticlesQueries.findArticlesPublishedBy(sourceId);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findArticlesPublishedBy(String username, String sourceId) {
String cypher = ArticlesQueries.findArticlesPublishedBy(username, sourceId);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findArticlesWithLanguage(String language) {
String cypher = ArticlesQueries.findArticlesWithLanguage(language, LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findArticlesWithLanguage(String username, String language) {
String cypher = ArticlesQueries.findArticlesWithLanguage(username, language, LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findArticlesWithCountry(String country) {
String cypher = ArticlesQueries.findArticlesWithCountry(country, LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findArticlesWithCountry(String username, String country) {
String cypher = ArticlesQueries.findArticlesWithCountry(username, country, LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findArticlesWithText(String text) {
String cypher = ArticlesQueries.findArticlesWithText(text, LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findLatest(String username) {
String cypher = ArticlesQueries.findLatest(username, LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findLatest() {
String cypher = ArticlesQueries.findLatest(LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findOldest(String username) {
String cypher = ArticlesQueries.findOldest(username, LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findOldest() {
String cypher = ArticlesQueries.findOldest(LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findRead(String username) {
String cypher = ArticlesQueries.findRead(username, LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findFavorite(String username) {
String cypher = ArticlesQueries.findFavorite(username, LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
public Iterable findFake(String username) {
String cypher = ArticlesQueries.findFake(username, LIMIT);
return getSession().query(NewsArticle.class, cypher, new HashMap<>());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy