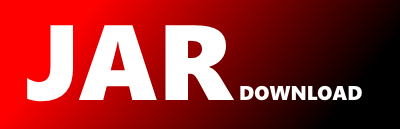
backend.services.AbstractDBService Maven / Gradle / Ivy
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package backend.services;
import backend.session.Neo4jSessionFactory;
import db.GraphEntity;
import java.util.Collection;
import java.util.HashMap;
import org.neo4j.ogm.cypher.query.SortOrder;
import org.neo4j.ogm.session.Session;
/**
*
* @author zua
* @param A graph entity subtype.
*/
public abstract class AbstractDBService {
public final int LIMIT = 30;
public Collection findAll() {
return getSession().loadAll(getClassType(), getSortOrderDesc(), LIMIT);
}
public T find(String id) {
Session session = Neo4jSessionFactory.getInstance().getNeo4jSession();
return session.load(getClassType(), id, 2);
}
public void save(T object) {
Session session = Neo4jSessionFactory.getInstance().getNeo4jSession();
session.save(object, 2);
}
public void delete(String id) {
Session session = Neo4jSessionFactory.getInstance().getNeo4jSession();
session.delete(find(id));
}
public long count() {
Session session = Neo4jSessionFactory.getInstance().getNeo4jSession();
return session.countEntitiesOfType(getClassType());
}
protected Session getSession() {
return Neo4jSessionFactory.getInstance().getNeo4jSession();
}
public Iterable executeQuery(String query) {
return getSession().query(getClassType(), query, new HashMap<>());
}
public Iterable executeQuery(Class aClass, String query) {
return getSession().query(aClass, query, new HashMap<>());
}
public T executeQueryForObject(String query) {
return getSession().queryForObject(getClassType(), query, new HashMap<>());
}
public abstract Class getClassType();
public abstract SortOrder getSortOrderAsc();
public abstract SortOrder getSortOrderDesc();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy