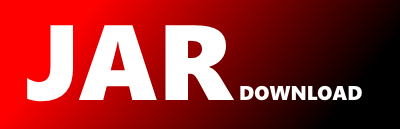
cdc.applic.demos.ActCctPctDemo Maven / Gradle / Ivy
package cdc.applic.demos;
import java.io.File;
import java.io.IOException;
import java.io.PrintStream;
import org.apache.logging.log4j.Level;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.io.IoBuilder;
import cdc.applic.dictionaries.NamingConvention;
import cdc.applic.dictionaries.handles.DictionaryHandle;
import cdc.applic.dictionaries.impl.RegistryImpl;
import cdc.applic.dictionaries.impl.RepositoryImpl;
import cdc.applic.dictionaries.impl.io.RepositoryIo;
import cdc.applic.dictionaries.s1000d.S1000DProperty;
import cdc.applic.expressions.Expression;
import cdc.applic.expressions.literals.Name;
import cdc.applic.s1000d.S1000DActCctPctCollector;
import cdc.applic.s1000d.S1000DActGenerator;
import cdc.applic.s1000d.S1000DCctGenerator;
import cdc.applic.s1000d.S1000DPctGenerator;
import cdc.applic.s1000d.S1000DProfile;
import cdc.applic.s1000d.core.S1000DActGeneratorImpl;
import cdc.applic.s1000d.core.S1000DCctGeneratorImpl;
import cdc.applic.s1000d.core.S1000DPctGeneratorImpl;
import cdc.util.debug.Verbosity;
import cdc.util.events.ProgressController;
import cdc.util.lang.FailureReaction;
public class ActCctPctDemo {
private static final Logger LOGGER = LogManager.getLogger(ActCctPctDemo.class);
private static final PrintStream OUT = IoBuilder.forLogger(LOGGER).setLevel(Level.INFO).buildPrintStream();
private final RepositoryImpl repository;
private final RegistryImpl registry;
private final DictionaryHandle handle;
public ActCctPctDemo(File file) throws IOException {
LOGGER.info("Load {}", file);
this.repository = RepositoryIo.load(file, FailureReaction.FAIL);
LOGGER.info("Loaded {}", file);
repository.print(OUT, Verbosity.ESSENTIAL);
this.registry = repository.getRegistry("/Encoded");
this.handle = new DictionaryHandle(registry);
}
private void testAct() {
LOGGER.info("Test ACT");
handle.clearComputationsCaches();
final S1000DActCctPctCollector handler = new S1000DActCctPctCollector();
final S1000DActGenerator generator = new S1000DActGeneratorImpl();
generator.generateAct(handle,
Expression.TRUE,
NamingConvention.DEFAULT,
handler,
ProgressController.VERBOSE);
LOGGER.info("Tested ACT");
handler.print(OUT, 1, Verbosity.ESSENTIAL);
handle.print(OUT, 1, Verbosity.ESSENTIAL);
}
private void testCct() {
LOGGER.info("Test CCT");
handle.clearComputationsCaches();
final S1000DActCctPctCollector handler = new S1000DActCctPctCollector();
final S1000DCctGenerator generator = new S1000DCctGeneratorImpl();
final S1000DProfile profile =
S1000DProfile.builder()
.supportAll()
.enable(S1000DProfile.Hint.NO_CONDITION_DEPENDENCIES)
.build();
generator.generateCct(handle,
Expression.TRUE,
NamingConvention.DEFAULT,
handler,
ProgressController.VERBOSE,
profile);
LOGGER.info("Tested CCT");
handler.print(OUT, 1, Verbosity.ESSENTIAL);
handle.print(OUT, 1, Verbosity.ESSENTIAL);
}
private void testPct() {
LOGGER.info("Test PCT");
handle.clearComputationsCaches();
final S1000DActCctPctCollector handler = new S1000DActCctPctCollector();
final S1000DPctGenerator generator = new S1000DPctGeneratorImpl();
final S1000DProperty master = registry.getProperty(Name.of("P5664"));
final S1000DProfile profile =
S1000DProfile.builder()
.supportAll()
.enable(S1000DProfile.Hint.NO_CONDITION_DEPENDENCIES)
.build();
generator.generatePct(handle,
new Expression("P5664<:{1~2}"),
NamingConvention.DEFAULT,
master,
handler,
ProgressController.VERBOSE,
profile);
LOGGER.info("Tested PCT");
handler.print(OUT, 1, Verbosity.NORMAL);
handle.print(OUT, 1, Verbosity.ESSENTIAL);
handle.removeComputationsCaches();
handle.print(OUT, 1, Verbosity.ESSENTIAL);
}
public static void main(String[] args) throws IOException {
final File file = new File("src/main/resources/repository.xlsx");
final ActCctPctDemo instance = new ActCctPctDemo(file);
instance.testAct();
instance.testCct();
instance.testPct();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy